Line Break (Multiple lines)
- Backtics
\n
with quotes (newline character)
let str1 = `Hello
World`;
let str2 = "Hello\nWorld";
Special Character
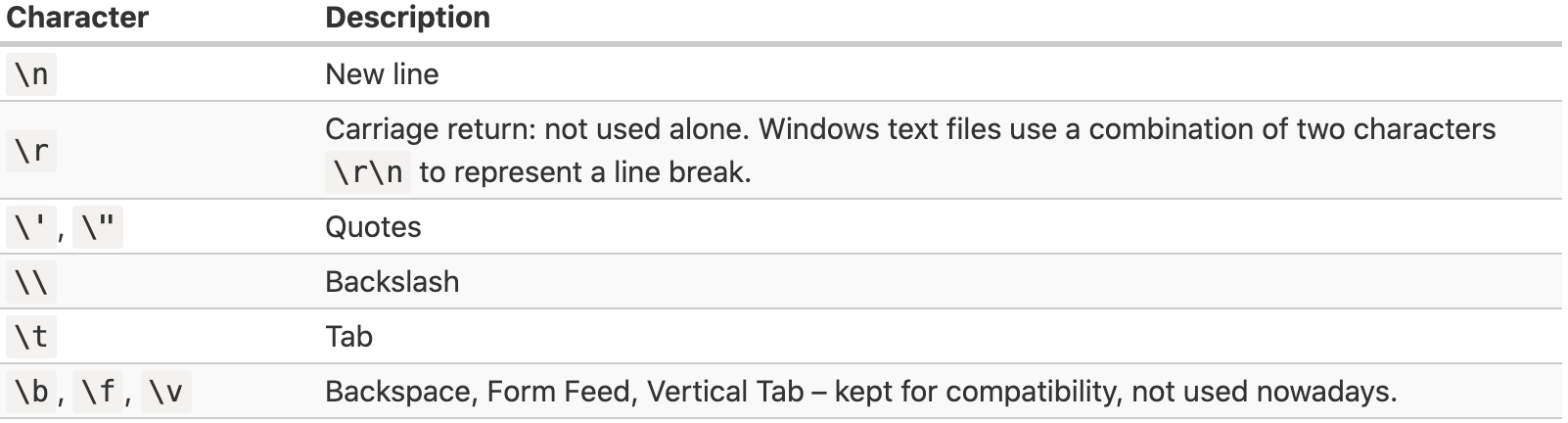
Accessing characters
let str = `Hello`;
alert( str[0] );
alert( str.charAt(0) );
alert( str[str.length - 1] );
alert( str[1000] );
alert( str.charAt(1000) );
for (let char of "Hello") {
alert(char);
}
Searching for a substring
let str = 'Widget with id';
alert( str.indexOf('Widget') );
alert( str.indexOf('widget') );
alert( str.indexOf("id") );
alert( str.indexOf('id', 2) )
var str = "scissors";
var indices = [];
for(var i=0; i<str.length;i++) {
if (str[i] === "s") indices.push(i);
}
Be careful when use "if" statement
let str = "Widget with id";
if (str.indexOf("Widget")) {
alert("We found it");
}
if (str.indexOf("Widget") != -1) {
alert("We found it");
}
Getting a substring
let str = "stringify";
alert( str.slice(0, 5) );
alert( str.slice(0, 1) );
alert( str.slice(2) );
alert( str.substring(2, 6) );
alert( str.substring(6, 2) );
alert( str.substr(2, 4) );
alert( str.substr(-4, 2) );
The others methods
alert( "Widget with id".includes("Widget") );
alert( "Widget".startsWith("Wid") );
alert( "Widget".endsWith("get") );
alert( 'Österreich'.localeCompare('Zealand') );