ex49
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box {
width: 150px; height: 150px;
position: absolute; left: 0; top: 0;
cursor: default;
}
#box1 { background-color: tomato;}
#box2 { background-color: gold;}
#box3 { background-color: cornflowerblue;}
#box4 { background-color: greenyellow;}
#box5 { background-color: plum;}
</style>
</head>
<body onselectstart="return false;">
<div id="box1" class="box">상자1</div>
<div id="box2" class="box">상자2</div>
<div id="box3" class="box">상자3</div>
<div id="box4" class="box">상자4</div>
<div id="box5" class="box">상자5</div>
<script>
const box1 = document.getElementById('box1');
const list = document.getElementsByClassName('box');
let index = 0;
let zindex = 1;
window.onmousedown = function(event) {
list[index].style.left = event.clientX - 75 + 'px';
list[index].style.top = event.clientY - 75 + 'px';
list[index].style.zIndex = zindex;
index++;
zindex++;
if (index >= list.length) index = 0;
};
</script>
</body>
</html>
✅ 마우스 우클릭 금지 방법
oncontextmenu = "return false"
: 우클릭 방지
onseletstart = "return false"
: 마우스 드래그 방지
ondragstart = "return false"
: 이미지 복사 드래그 방지
onkeydown = "return false"
: 키보드 단축키 복사 방지
- script 코드를 삽입
<script type="text/javascript">
document.oncontextmenu = function(){return false;}
</script>
- 코드를 삽입
<body oncontextmenu="return false" onselectstart="return false" ondragstart="return false" onkeydown="return false">
ex50
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box { width: 150px; height: 150px;
position: absolute; left: 0; top: 0;
cursor: default;}
#box1 { background-color: tomato;}
#box2 { background-color: gold;}
#box3 { background-color: cornflowerblue;}
#box4 { background-color: greenyellow;}
#box5 { background-color: plum;}
</style>
</head>
<body>
<script>
window.onmousedown =function(event) {
if (event.buttons == 1) {
let box = document.createElement('div');
box.classList.add('box');
box.style.left = event.clientX - 75 + 'px';
box.style.top = event.clientY - 75 + 'px';
let r = parseInt(Math.random() * 256);
let g = parseInt(Math.random() * 256);
let b = parseInt(Math.random() * 256);
box.style.backgroundColor = `rgb(${r},${g},${b})`;
document.body.appendChild(box);
} else if (event.buttons == 2) {
document.body.removeChild(event.target);
}
};
</script>
</body>
</html>
ex51
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box { width: 150px; height: 150px;
position: absolute; left: 0; top: 0;
cursor: default;
background-color: gold;
}
</style>
</head>
<body>
<div class="box" id="box"></div>
<script>
const box = document.getElementById('box');
let isDown = false;
let x = 0;
let y = 0;
window.onmousedown = function(event) {
if (event.target.id == 'box') {
isDown = true;
x = event.offsetX;
y = event.offsetY;
}
};
window.onmousemove = function(event) {
if (isDown) {
box.style.left = event.clientX - x + 'px';
box.style.top = event.clientY - y + 'px';
}
};
window.onmouseup = function(event) {
isDown = false;
};
</script>
</body>
</html>
ex52
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.draggable {
position: absolute;
left: 0;
top: 0;
}
.box {
width: 150px;
height: 150px;
cursor: default;
background-color: gold;
border: 1px solid black;
}
</style>
</head>
<!-- 마우스 드래그 방지 -->
<body onselectstart="return false;">
<h1 class="draggable">제목입니다.</h1>
<div class="box draggable">상자1</div>
<div class="box draggable">상자2</div>
<div class="box draggable">상자3</div>
<input type="text" class="draggable">
<img src="images/cat01.jpg" class="draggable">
<script>
let isDown = false;
let x = 0;
let y = 0;
let obj;
window.onmousedown = function(event) {
if (event.target.classList.contains('draggable')) {
isDown = true;
x = event.offsetX;
y = event.offsetY;
obj = event.target;
}
};
window.onmousemove = function(event) {
if (isDown) {
obj.style.left = event.clientX - x + 'px';
obj.style.top = event.clientY - y + 'px';
event.stopPropagation();
return false;
}
};
window.onmouseup = function(event) {
isDown = false;
};
</script>
</body>
</html>
ex53
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box {
width: 150px;
height: 150px;
cursor: default;
background-color: gold;
border: 1px solid black;
}
</style>
</head>
<body>
<div class="box" id="box" style="width: 150px; height: 150px;"></div>
<script>
const box = document.getElementById('box');
let isDown = false;
window.onmousedown = function(event) {
if (event.target.id == 'box'){
if (isValid(event)) {
isDown = true;
box.style.cursor = 'nw-resize';
box.style.opacity = .3;
}
}
};
function isValid(event) {
let w = parseInt(box.style.width);
let h = parseInt(box.style.height);
if (event.offsetX >= (w-10) && event.offsetX <= w && event.offsetY >=(h-10) && event.offsetY <= h) {
return true;
} else {
return false;
}
}
window.onmousemove = function(event) {
if (isDown) {
box.style.width = event.clientX + 'px';
box.style.height = event.clientY + 'px';
}
};
window.onmouseup = function(event) {
isDown = false;
box.style.cursor = 'default';
box.style.opacity = 1;
};
</script>
</body>
</html>
ex54
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#cat3 {
position: absolute;
left: 125px;
top: 50px;
}
</style>
</head>
<body>
<!-- 250 X 188 -->
<img src="images/cat01.jpg" id="cat1">
<img src="images/cat02.jpg" id="cat2">
<img src="images/cat03.jpg" id="cat3">
<script>
window.onmousedown = function(event) {
let x = event.clientX;
let y = event.clientY;
console.log(document.elementsFromPoint(x, y));
};
console.log(document.elementFromPoint(100, 350).nodeName);
</script>
</body>
</html>
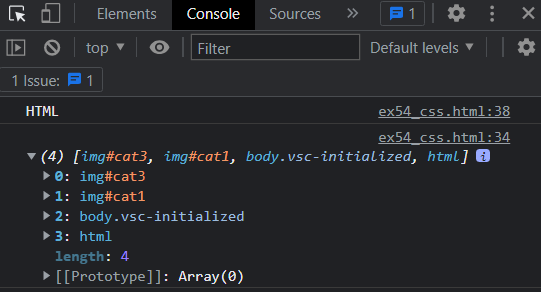