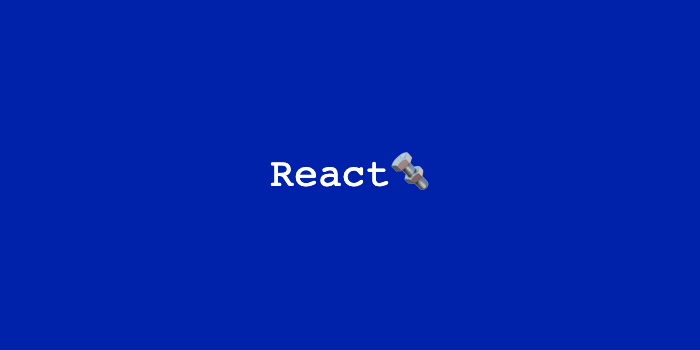
의사 코드
1. useState를 사용해 input값을 저장한다.
2. array 메소드인 filter로 입력된 input값을 포함한 요소들만 배열로 리턴한다.
3. array 메소드인 map으로 필터링된 배열을 드롭다운 형태로 렌더링 한다.
먼저, 훅(useState, useEffect)과 CSS용 스타일 컴포넌트를 불러온다.
import { useState, useEffect } from 'react';
import styled from 'styled-components';
styled-components 영역
const boxShadow = '0 4px 6px rgb(32 33 36 / 28%)';
const activeBorderRadius = '1rem 1rem 0 0';
const inactiveBorderRadius = '1rem 1rem 1rem 1rem';
export const InputContainer = styled.div`
margin-top: 8rem;
background-color: #ffffff;
display: flex;
flex-direction: row;
padding: 1rem;
border: 1px solid rgb(223, 225, 229);
border-radius: ${inactiveBorderRadius};
z-index: 3;
box-shadow: 0;
&:focus-within {
box-shadow: ${boxShadow};
}
> input {
flex: 1 0 0;
background-color: transparent;
border: none;
margin: 0;
padding: 0;
outline: none;
font-size: 16px;
}
> div.delete-button {
cursor: pointer;
}
`;
export const DropDownContainer = styled.ul`
background-color: #ffffff;
display: block;
margin-left: auto;
margin-right: auto;
list-style-type: none;
margin-block-start: 0;
margin-block-end: 0;
margin-inline-start: 0px;
margin-inline-end: 0px;
padding-inline-start: 0px;
margin-top: -1px;
padding: 0.5rem 0;
border: 1px solid rgb(223, 225, 229);
border-radius: 0 0 1rem 1rem;
box-shadow: ${boxShadow};
z-index: 3;
> li {
padding: 0 1rem;
&.selected {
background-color: lightgray;
}
}
`;
자동 완성을 위한 요소들을 넣은 배열을 선언한다.
const deselectedOptions = [
'rustic',
'antique',
'vinyl',
'vintage',
'refurbished',
'신품',
'빈티지',
'중고A급',
'중고B급',
'골동품'
];
자동 완성 구현 영역
export const Autocomplete = () => {
const [hasText, setHasText] = useState(false);
const [inputValue, setInputValue] = useState('');
const [options, setOptions] = useState(deselectedOptions);
useEffect(() => {
if (inputValue === '') {
setHasText(false);
setOptions([]);
} else {
setOptions(deselectedOptions.filter((option) => {
return option.includes(inputValue)
}))
}
}, [inputValue]);
const handleInputChange = (event) => {
setInputValue(event.target.value);
setHasText(true);
};
const handleDropDownClick = (clickedOption) => {
setInputValue(clickedOption);
};
const handleDeleteButtonClick = (event) => {
setInputValue('');
};
return (
<div className='autocomplete-wrapper'>
<InputContainer>
<input onChange={handleInputChange} value={inputValue}></input>
<div className='delete-button' onClick={handleDeleteButtonClick}>×</div>
</InputContainer>
{hasText && <DropDown options={options} handleComboBox={handleDropDownClick} />}
</div>
);
};
export const DropDown = ({ options, handleComboBox, selected }) => {
return (
<DropDownContainer>
{options.map((option, index) => {
return (
<li key = {index}
onClick = {() => handleComboBox(option)}>
{option}
</li>
)
})}
</DropDownContainer>
);
};
```