빈칸 체크 함수 만들기
- 클릭 버튼 눌렀을 때 입력한 글자로 alert 띄우기
- 버튼을 눌렀을 때 칸에 아무것도 없으면 '입력하세요' alert 띄우기

<div>
<input id="input-q1" /><button onclick="q1()">클릭</button>
</div>
----------------------------------------------------
<script>
function q1() {
let txt = $('#input-q1').val(); ✅ id값 가져온다.
console.log(txt) ✅항상 먼저 찍어서 확인하고 넘어가기. 그래야 실수를 줄인다.
}
</script>
[2] if, else 함수
<script>
function q1() {
let txt = $('#input-q1').val();
🔸 if (txt == '') {
alert('입력하세요!')
} else {
alert(txt)
}
}
</script>
이메일 판별 함수 만들기
- 입력받은 값에 @가 있으면 이메일 도메인만 alert 띄우기
- 이메일이 아니면(@가 없으면) '이메일 아님' alert 띄우기
<script>
function q2() {
let txt = $('#input-q2').val();
console.log(txt);
}
</script>
[1-2] includes() 이용
<script>
function q2() {
let txt = $('#input-q2').val();
🔸 console.log(txt.include('@'));
}
✅include 값이 포함 되어 있으면 콘솔창에 true, 없으면 false라고 뜬다.
</script>
[2-1] 알럿 띄우기
<script>
function q2() {
let txt = $('#input-q2').val();
🔸 if (txt.include('@')) {
alert('이메일');
} else {
alert('이메일 아니다');
}
}
</script>
[2-2] txt에 domain값만 때서 띄우기
<script>
function q2() {
let txt = $('#input-q2').val();
if (txt.include('@')) {
🔸 let domain = txt.split('@')[1].split('.')[0];
alert(domain);
} else {
alert('이메일 아니다');
}
}
</script>
HTML 붙이기/지우기
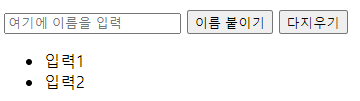
[1-1] id값 가져오기
<script>
function q3() {
let txt = $('#input-q3').val();
let temp_html = `<li>${txt}</li>` ❗ backtick(``)
🔺목록 나타내는 태크
console.log(temp_html) ✅항상 확인!
}
</script>
[1-2] 태그 붙이기
<script>
function q3() {
let txt = $('#input-q3').val();
let temp_html = `<li>${txt}</li>`
🔸 $('#names-q3').append(temp_html)
}
</script>
[2] remove() 이용
<script>
function q3_remove() {
$('#names-q3').empty(); ✅names-q3의 내부 태그를 모두 비운다.
}
</script>