- EntityFramework Core 설치
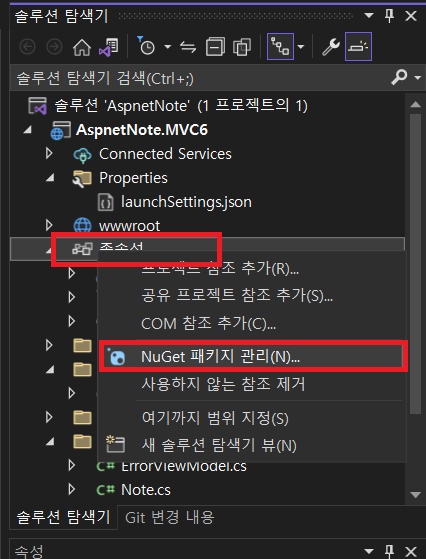
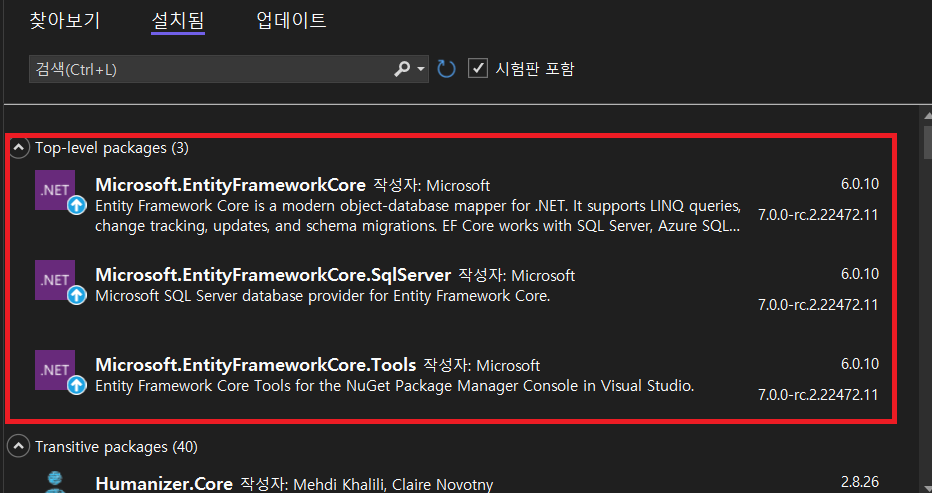
- Model 생성
using System.ComponentModel.DataAnnotations;
namespace AspnetNote.MVC6.Models
{
public class User
{
/// <summary>
/// 사용자 번호
/// </summary>
[Key] // PK 설정 어노테이션
public int UserNo { get; set; }
/// <summary>
/// 사용자 이름
/// </summary>
[Required] // Not Null 설정
public string UseName { get; set; }
/// <summary>
/// 사용자 ID
/// </summary>
[Required] // Not Null 설정
public string UserId { get; set; }
/// <summary>
/// 사용자 비밀번호
/// </summary>
[Required] // Not Null 설정
public string UserPassword { get; set; }
}
}
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace AspnetNote.MVC6.Models
{
public class Note
{
/// <summary>
/// 게시물 번호
/// </summary>
[Key]
public int NoteNo { get; set; }
/// <summary>
/// 게시물 제목
/// </summary>
[Required]
public string NoteTitle { get; set; }
/// <summary>
/// 게시물 내용
/// </summary>
[Required]
public string NoteContents { get; set; }
/// <summary>
/// 작성자 번호
/// </summary>
[Required]
public int UserNo { get; set; }
[ForeignKey("UserNo")]
public virtual User User { get; set; }
}
}
- DbContext 생성 -> Table 생성할 수 있는 코드를 작성
using AspnetNote.MVC6.Models;
using Microsoft.EntityFrameworkCore;
namespace AspnetNote.MVC6.DataContext
{
public class AspnetNoteDbContext : DbContext // : (상속)
{
public DbSet<User> Users { get; set; }
public DbSet<Note> Notes { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer(@"Server=localhost;Database=AspnetNoteDb;User Id=sa;Password=sa1234;");
}
}
}
- DbContext -> 실제 테이블 생성
add-migration FirstMigration
update-database
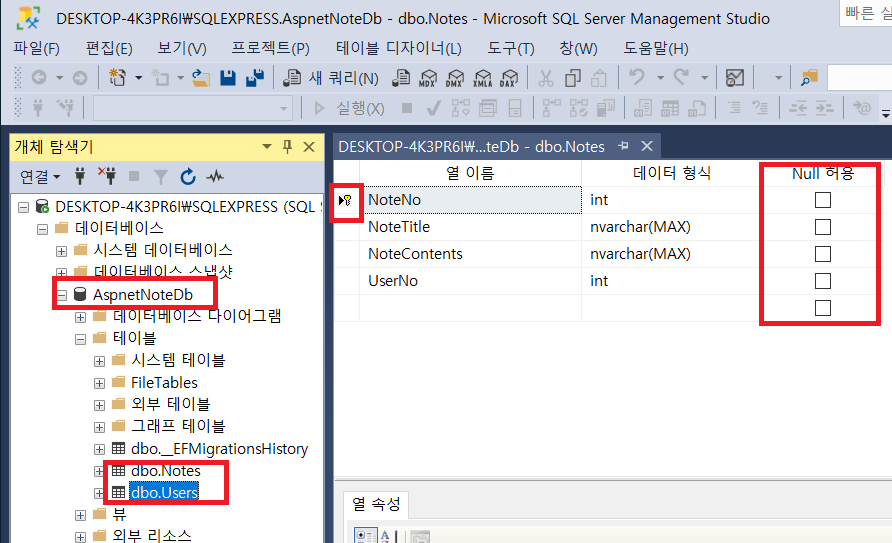