Test-Driven Development
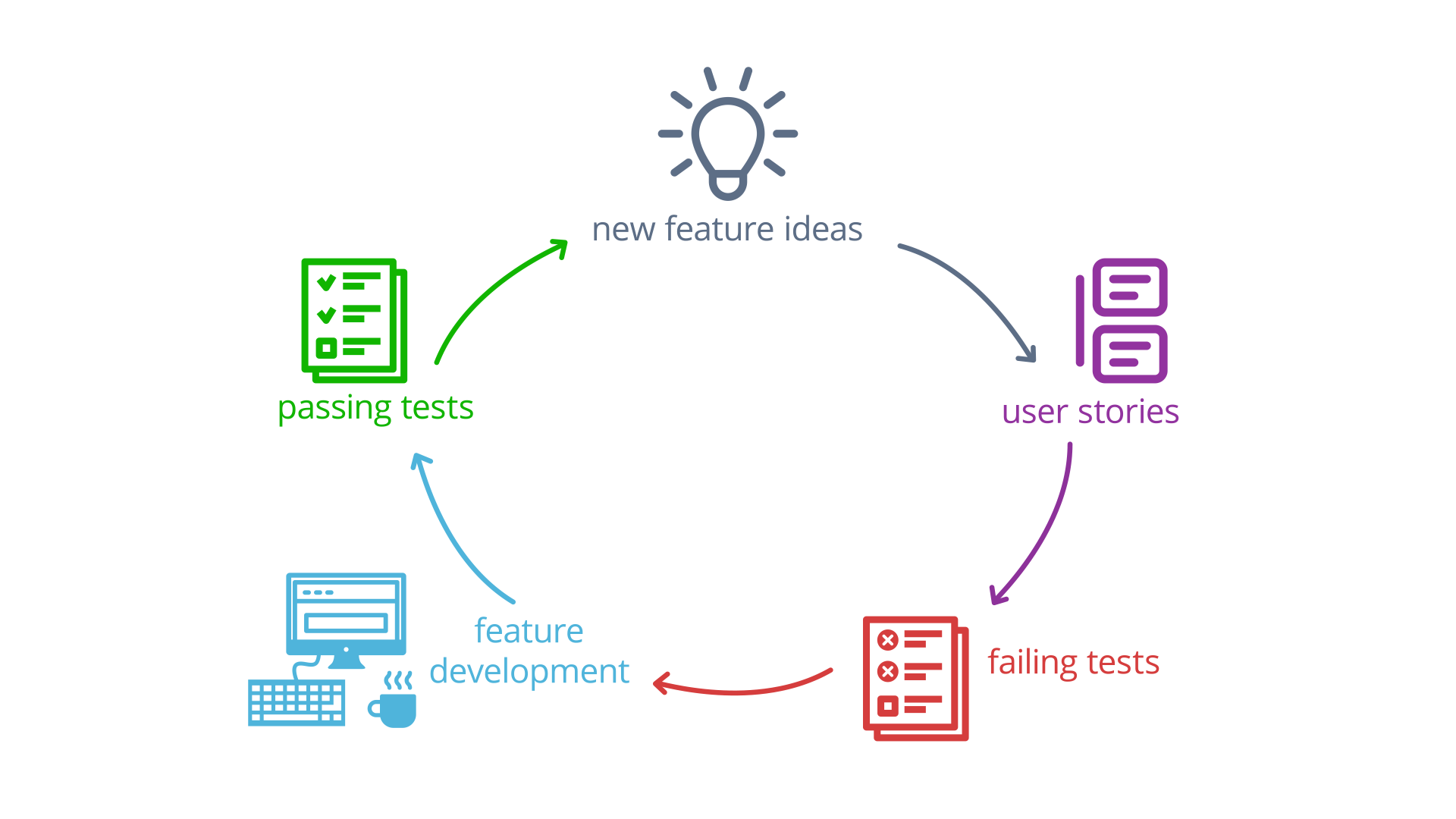
- 테스팅은 소프트웨어 개발에서 매우 유용하며 개발 시간을 단축시킨다.
- TDD(Test-Driven Development)는 코드를 작성하기 전에 테스트를 미리 작성하여 개발하는 것을 말한다.
- 테스트는 유저의 스토리를 담는다. 그 예시는 다음과 같다. As a user, I can take some action in order to achieve some goal.
- Unit Test는 개별적 요소에 대한 테스트이며, Integration Test는 여러개의 요소에 대한 유저의 상호작용이나 흐름에 대한 테스트이다.
JUnit
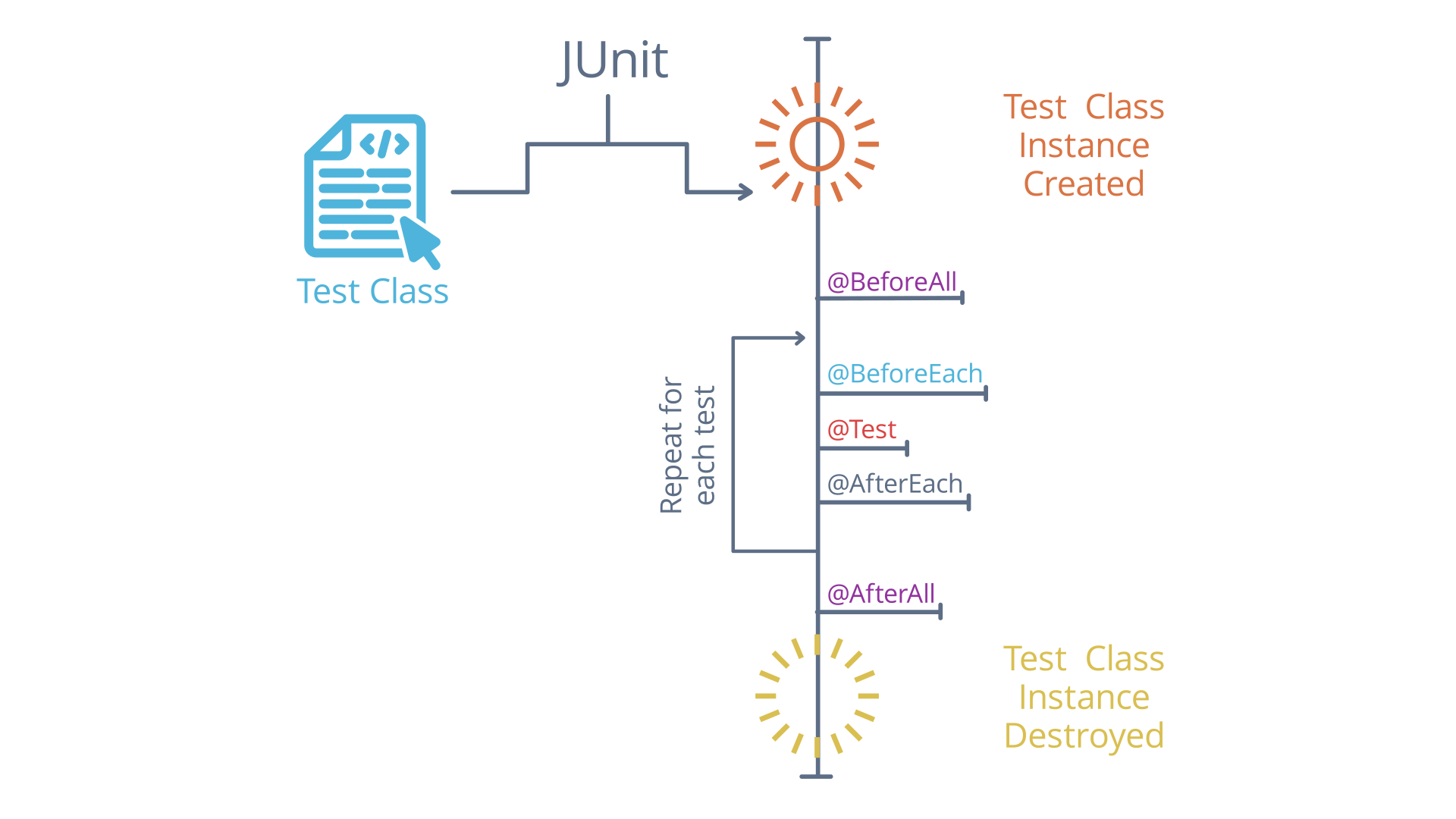
- JUnit은 Java의 표준 테스트 프레임워크이다. 이름처럼 Unit Test 기능 이상을 할 수 있다.
- @Test, @BeforeAll, @BeforeEach 등과 같은 annotation을 쓸 수 있으며 assertEqual이나 assertThrows와 같은 방식으로 테스트 할 수 있다.
- IntelliJ와 같은 IDE에서 code coverage(코드 실행 횟수), Debugging, Unit Test Report를 활용할 수 있다.
Selenium
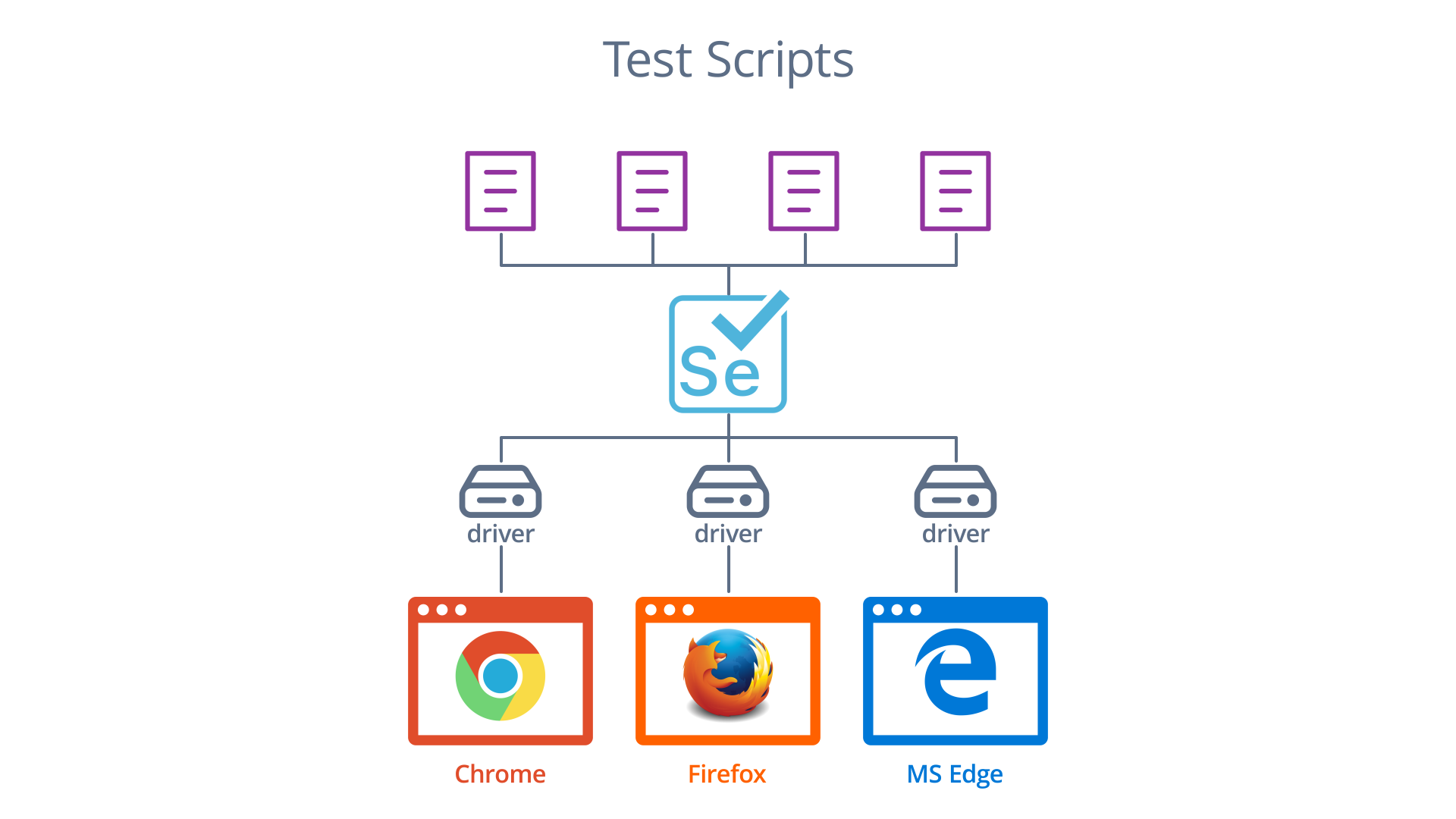
- Selenium은 Web Driver를 통해 웹 브라우저의 자동화를 가능하게 하고 지원하는 다양한 도구와 라이브러리를 포함한 도구이다.
public static void main(String[] args) throws InterruptedException {
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.get("http://www.google.com");
WebElement inputField = driver.findElement(By.name("q"));
inputField.sendKeys("selenium");
inputField.submit();
List<WebElement> results = driver.findElements(By.cssSelector("div.g a"));
for (WebElement element : results) {
String link = element.getAttribute("href");
System.out.println(link);
}
Thread.sleep(5000);
driver.quit();
}
- WebDriver를 통해 브라우져를 설정하고 WebElement를 통해 특정 요소를 가져와 조작할 수 있다.
@FindBy(id = "reset-button")
private WebElement resetButton;
public CounterPage(WebDriver driver) {
PageFactory.initElements(driver, this);
}
- JUnit과 Selenium을 같이 쓰면 위와 같이 손쉽게 쓸 수도 있다.