1. 문제
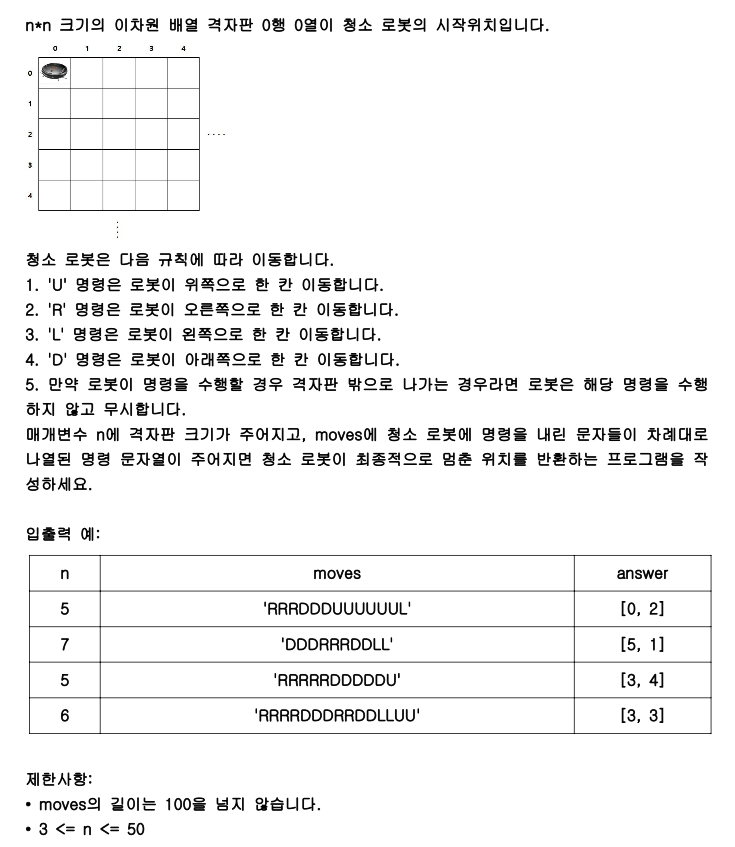
2. 코드
public class Main {
public int[] solution(String moves) {
int x = 0, y = 0;
int[] dx = {-1, 0, 1, 0};
int[] dy = {0, 1, 0, -1};
char[] direction = {'U', 'R', 'D', 'L'};
for (char move : moves.toCharArray()) {
for (int i = 0; i < direction.length; i++) {
if (move == direction[i]) {
int nx = x + dx[i];
int ny = y + dy[i];
if (0 <= nx && nx < 100 && 0 <= ny && ny < 100) {
x = nx;
y = ny;
}
break;
}
}
}
return new int[]{x, y};
}
public static void main(String[] args) {
Main T = new Main();
System.out.println(Arrays.toString(T.solution("RRRDDDLU")));
}
}
public class Main {
public int[] solution(String moves) {
int x = 0, y = 0;
int[] dx = {-1, 0, 1, 0};
int[] dy = {0, 1, 0, -1};
for (char c : moves.toCharArray()) {
if (c == 'U') {
x = x + dx[0];
y = y + dy[0];
} else if (c == 'R') {
x = x + dx[1];
y = y + dy[1];
} else if (c == 'D') {
x = x + dx[2];
y = y + dy[2];
} else if (c == 'L') {
x = x + dx[3];
y = y + dy[3];
}
}
return new int[]{x, y};
}
public static void main(String[] args) {
Main T = new Main();
System.out.println(Arrays.toString(T.solution("RRRDDDLU")));
}
}
HashMap<Character, Integer> map = new HashMap<>();
map.put('U', 0);
map.put('R', 1);
map.put('D', 2);
map.put('L', 3);
int[] dx = {-1, 0, 1, 0};
int[] dy = {0, 1, 0, -1};
int x = 0;
int y = 0;
for (char ch : s.toCharArray()) {
x += dx[map.get(ch)];
y += dy[map.get(ch)];
}
System.out.printf("[%d, %d]\n", x, y);
3. 풀이
- Map이 탐색이 O(1)인 거는 맞지만, 기본적으로 Map 라이브러리 자체가 가볍지는 않아서 해당 유형에서는 배열로 하는 것을 추천한다.