1. 문제
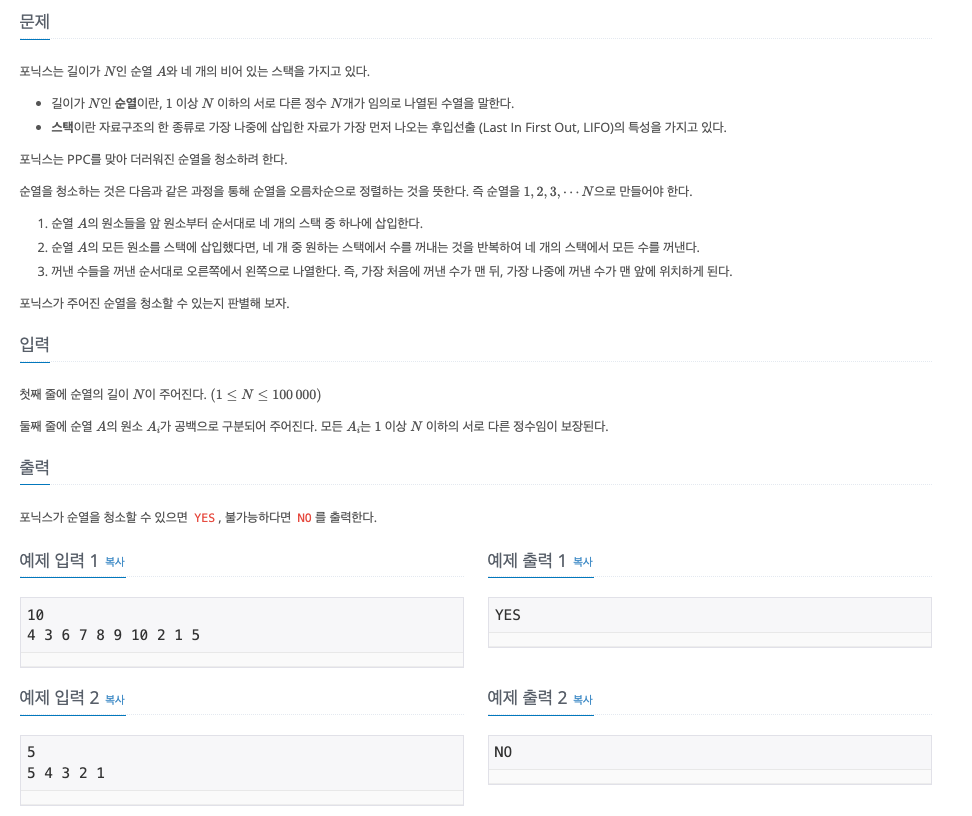
2. Code
import java.util.Arrays;
import java.util.Scanner;
import java.util.Stack;
class p25556 {
public static void solution() {
Scanner scanner = new Scanner(System.in);
int numberCount = scanner.nextInt();
if (! (numberCount >= 1 && numberCount<=100000)){
return;
}
scanner.nextLine();
String number = scanner.nextLine();
String[] tokens = number.split(" ");
int[] arr = new int[tokens.length];
for (int i = 0; i < tokens.length; i++) {
arr[i] = Integer.parseInt(tokens[i]);
}
Stack[] stacks = new Stack[4];
for (int i = 0; i < 4; i++)
stacks[i] = new Stack<Integer>();
int idx = 0;
for (int i = 0; i < numberCount; i++) {
int target = arr[i];
boolean flag = false;
for (int j = 0; j < 4; j++) {
if (stacks[j].isEmpty()) {
flag = true;
push(stacks[j], target);
break;
} else if (target > (Integer) stacks[j].peek()) {
flag = true;
push(stacks[j], target);
break;
}
}
if (!flag) {
System.out.println("NO");
return;
}
}
System.out.println("YES");
}
public static boolean push(Stack stacks, int target) {
stacks.push(target);
return true;
}
public static void main(String[] args) {
solution();
}
}
3. 풀이
- push를 하기 이전에 stack의 최상단의 값이 넣으려는 값보다 작다면 다른 stack에 넣어야 한다
- 모든 수를 스택에 넣을 수 있다면 굳이 pop 하지 않더라도 성공한다.
4. 링크
https://www.acmicpc.net/problem/25556