브라우저 요청과 응답하는 코드를 try-with-resource를 사용하지 않고,
try catch 문으로 복습 차원으로 다시 작성해봤다.
try-with-resources를 사용하면 사용한 IO들을 알아서 닫아주는 역할을 한다.
사용할 땐 몰랐지만 t-w-r을 사용하지 않았을 때 자원을 어디서 닫아야 하는지
무지했기에 이번 복습 기회에 배움을 얻었다.😊
try-with-resources
try (
} catch() {
}
1. Client / Server
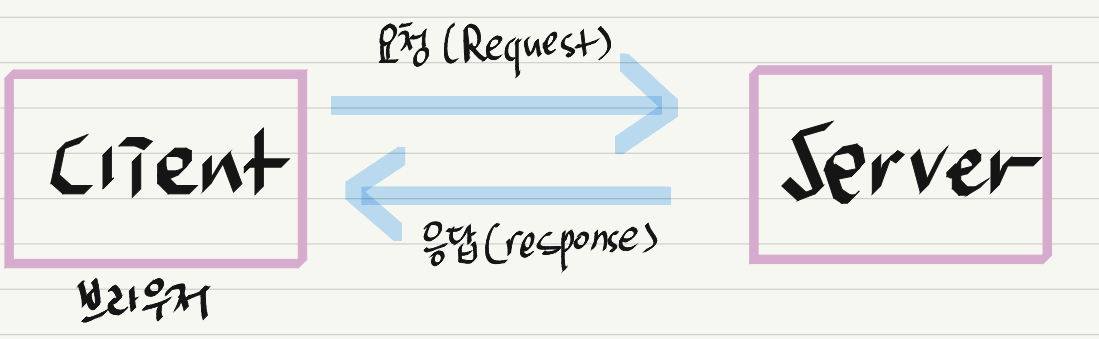
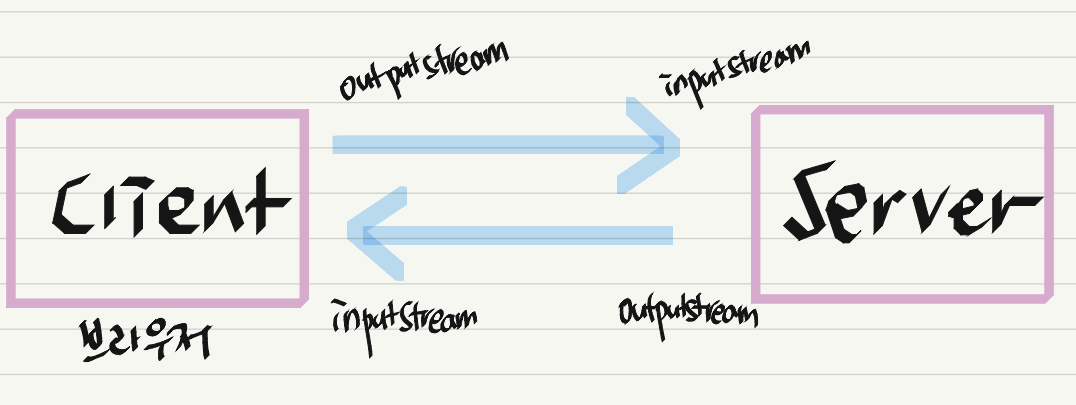
2. 요청과 응답하기
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.charset.StandardCharsets;
public class HTTP {
public static void main(String[] args) {
String html = "<!DOCTYPE html>\n" +
"<html>\n" +
" <head>\n" +
" <title>Hello HTTP Server!</title>\n" +
" </head>\n" +
" <body>\n" +
" <h1>HEADER</h1>\n" +
" <p>Contents</p>\n" +
" </body>\n" +
"</html>";
try {
ServerSocket serverSocket = new ServerSocket(12345);
System.out.println("클라이언트 대기 중");
Socket socket = serverSocket.accept();
System.out.println("클라이언트 접속");
InputStream in = socket.getInputStream();
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String line;
while(!(line = br.readLine()).equals("")) {
System.out.println(line);
}
OutputStream out = socket.getOutputStream();
String statusLine = "HTTP/1.1 200 OK \r\n";
out.write(convertStringToBytes(statusLine));
String contentType = "Content-Type : text/html; charset=utf-8;";
out.write(convertStringToBytes(contentType+"\r\n"));
String contentLength = "Content-Length :" + html.length();
out.write(convertStringToBytes(contentLength+"\r\n"));
out.write(convertStringToBytes("\r\n"));
out.write(convertStringToBytes(html));
out.flush();
socket.close();
serverSocket.close();
in.close();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static byte[] convertStringToBytes(String st) {
return st.getBytes(StandardCharsets.UTF_8);
}
}
References
- 🎈2020.11.27
- 🎈정리 : song, ipad
