문제📖
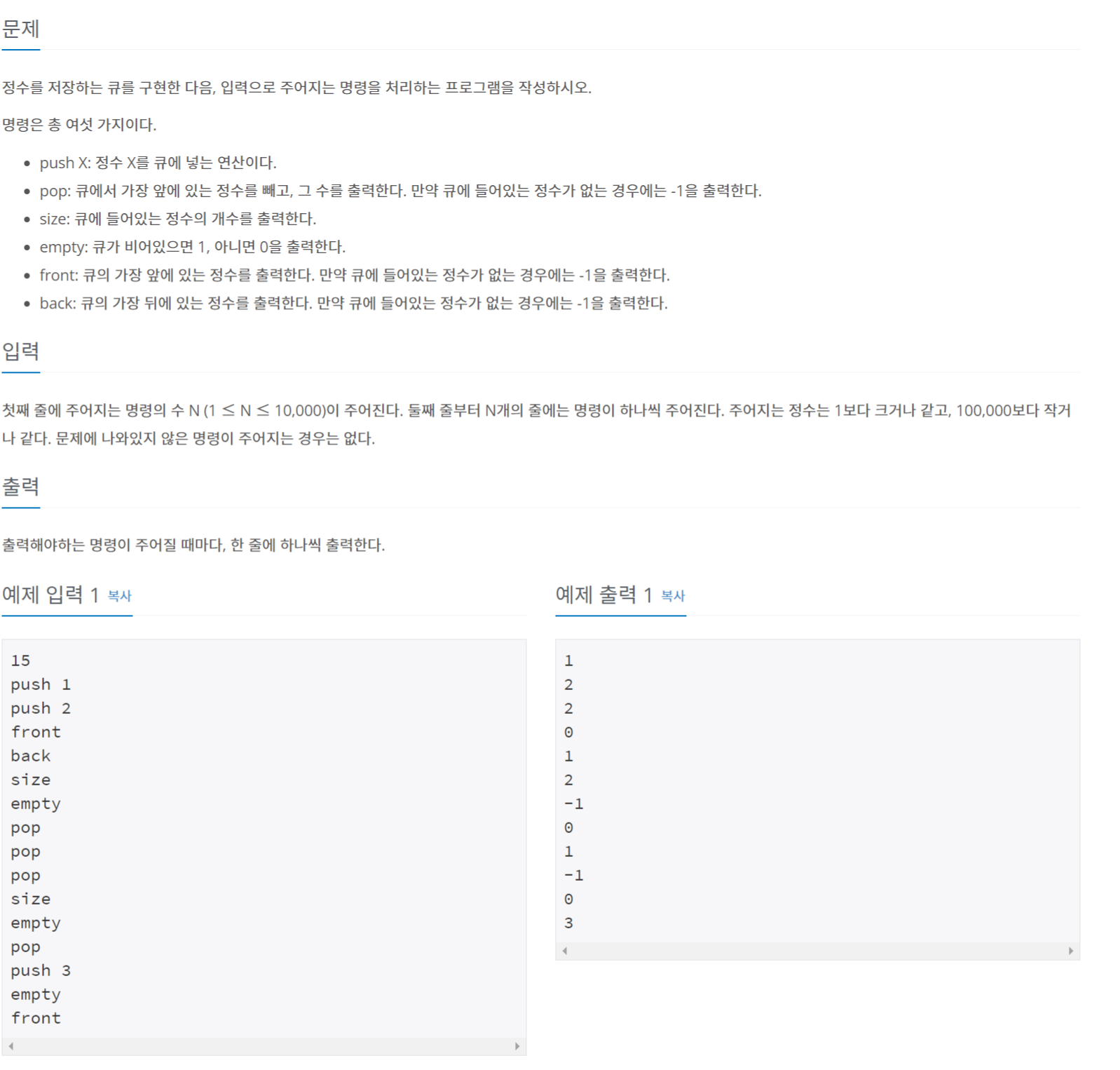
풀이🙏
- 큐를 사용하기 위해 deque (덱)을 사용한다. (선입선출 FIFO)
q.append()
는 큐의 맨 뒤에 삽입한다.
q.popleft()
는 큐의 맨 앞 요소를 삭제한다.
len(q)
를 이용하여 큐가 비어있는지 확인하다.
- 큐의 맨 앞 요소를 확인하기 위해서는
q[0]
, 큐의 맨 뒤 요소를 확인하기 위해서는 q[-1]
을 이용한다.
코드💻
import sys
from collections import deque
input = sys.stdin.readline
q = deque()
for _ in range(int(input())):
command = input().split()
if command[0] == "push":
q.append(command[1])
elif command[0] == "pop":
if len(q) == 0:
print(-1)
else:
print(q.popleft())
elif command[0] == "size":
print(len(q))
elif command[0] == "empty":
if len(q) == 0:
print(1)
else:
print(0)
elif command[0] == "front":
if len(q) == 0:
print(-1)
else:
print(q[0])
else:
if len(q) == 0:
print(-1)
else:
print(q[-1])