유튭을 더 재밌게 -> 튜브둥둥
- 유툽 검색결과(이번 달, 조회순 많은 순)를 파일로 한눈에 볼 수 있게 정리
- 검색키워드별 누적 조회수를 비교하여 관심 정도 확인
- 영상제목의 키워드를 워드클라우드로 시각화
-> 영상검색, 마케팅 등 의사결정 정보로 활용
import sys
import os
import time
import warnings
warnings.filterwarnings('ignore')
import chromedriver_autoinstaller
import pandas as pd
import numpy as np
from selenium import webdriver
1. 자료추출
user = "먹방"
path = chromedriver_autoinstaller.install()
driver = webdriver.Chrome(path)
driver.get("https://www.youtube.com/results?search_query={}".format(user))
time.sleep(1)
driver.find_element_by_css_selector('.style-scope.ytd-toggle-button-renderer.style-text').click()
time.sleep(1)
driver.find_element_by_link_text("이번 달").click( )
def scroll_down(driver):
driver.execute_script("window.scrollTo(0, 21431049)")
n = 10
i = 0
while i < n:
scroll_down(driver)
i = i+1
time.sleep(0.5)
a_id = "#video-title"
raws = driver.find_elements_by_css_selector(a_id)
len(raws)
128
aria_list = []
url_list = []
for raw in raws:
aria = raw.get_attribute('aria-label')
aria_list.append(aria)
for u in raws:
url = u.get_attribute('href')
url_list.append(url)
print(len(aria_list), len(url_list))
128 128
2. 데이터정제 및 분류
dt = list(filter(None,aria_list))
url_list = list(filter(None, url_list))
print(len(dt), len(url_list))
128 128
dt[0]
'[ENG]사장님 "어머 유튜버 OO보다 많이 먹었어!!" 가뿐하게 부산가서 신기록 세우고 왔습니다..^^ 떡볶이꼬치 최대 몇개까지 먹어봤니? 게시자: 웅이woongei 22시간 전 13분 24초 조회수 344,543회'
title_list = []
view_cnt_list =[]
for i in range(len(dt)):
content = dt[i].split(' 게시자: ')
title = content[0]
title_list.append(title)
t_remain = content[1].split(' ')
view_cnt = t_remain[-1]
view_cnt = view_cnt.replace('회','')
view_cnt = view_cnt.replace(',','')
view_cnt = view_cnt.replace('없음','0')
view_cnt_list.append(int(view_cnt))
time.sleep(1)
print(len(title_list), len(view_cnt_list), len(url_list))
128 128 128
3. DF 정렬 및 파일저장
df = pd.DataFrame({'title':title_list, 'view_cnt':view_cnt_list, 'url':url_list})
df = df.sort_values('view_cnt',ascending=False)
df = df.reset_index(drop=True)
df.head(2)
|
title |
view_cnt |
url |
0 |
편의점 사장님 어리둥절🙄 짜파게티 김밥 만두 편의점 털기ㅋㅋ |
4967815 |
https://www.youtube.com/watch?v=t3Bt1ooBrnk |
1 |
리얼먹방:) 밥도둑 "묵은지 닭볶음탕" ★ 바삭바삭 부추전 ㅣKimchi Dak-b... |
3716054 |
https://www.youtube.com/watch?v=6fPTXRA2apQ |
df.to_csv("tube_dungdung({}).csv".format(user), encoding='utf-8-sig')
4. 둥둥 시각화
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib as mpl
from matplotlib import font_manager, rc
import nltk
mpl.rcParams['font.family'] = 'Malgun Gothic'
mpl.rcParams['font.size'] = 15
mpl.rcParams['axes.unicode_minus'] = False
dung = pd.read_csv('./tube_dungdung(영화).csv')
dung = dung.drop(['Unnamed: 0'], axis=1)
dung = dung.head(30)
dung2 = pd.read_csv('./tube_dungdung(먹방).csv')
dung2 = dung2.drop(['Unnamed: 0'], axis=1)
dung2 = dung2.head(30)
라인차트 그리기 (키워드 조회수 비교)
x = range(1,31)
y1 = dung['view_cnt'].cumsum()
y2 = dung2['view_cnt'].cumsum()
y1[-1:]
29 32236858
Name: view_cnt, dtype: int64
plt.plot(x, y2, marker = '*', label='영화', color='r')
plt.plot(x, y1, marker = 'o', label='먹방')
plt.xlabel('영상수', color='g', loc='right')
plt.ylabel('조회수', color='g', loc='top')
plt.title('< ' + '누적 조회수 ' + '>')
plt.legend(loc=(0.7, 0.2))
plt.text(x[29], y1[-1:] + 0.25, '%d' %y1[-1:], ha='center', va='bottom', size=12)
plt.text(x[29], y2[-1:] + 0.25, '%d' %y2[-1:], ha='center', va='bottom', size=12);
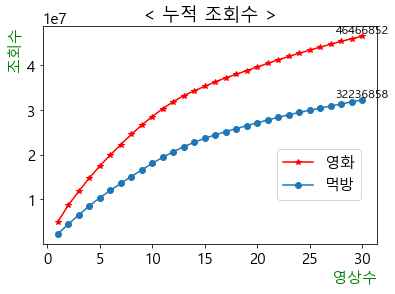
from konlpy.tag import Okt
from collections import Counter
from wordcloud import WordCloud, STOPWORDS
script = dung2['title']
script.head(2)
0 편의점 사장님 어리둥절🙄 짜파게티 김밥 만두 편의점 털기ㅋㅋ
1 리얼먹방:) 밥도둑 "묵은지 닭볶음탕" ★ 바삭바삭 부추전 ㅣKimchi Dak-b...
Name: title, dtype: object
script.to_csv('word.txt', encoding='utf-8-sig')
text = open('word.txt', encoding='utf-8-sig').read()
def token_konlpy(text):
okt=Okt()
return [word for word in okt.nouns(text) if len(word)>1]
noun = token_konlpy(text)
len(noun)
257
noun_set = set(noun)
len(noun_set)
192
f = open('noun_set.txt','w', encoding='utf-8')
f.write(str(noun_set))
f.close()
count = Counter(noun)
count.pop('먹방')
len(count)
191
word = dict(count.most_common(30))
wc = WordCloud(max_font_size=200, font_path = 'C:\Windows\Fonts\malgun.ttf',background_color="white",width=2000, height=500).generate_from_frequencies(word)
plt.figure(figsize = (40,40))
plt.imshow(wc)
plt.tight_layout(pad=0)
plt.axis('off')
plt.show()
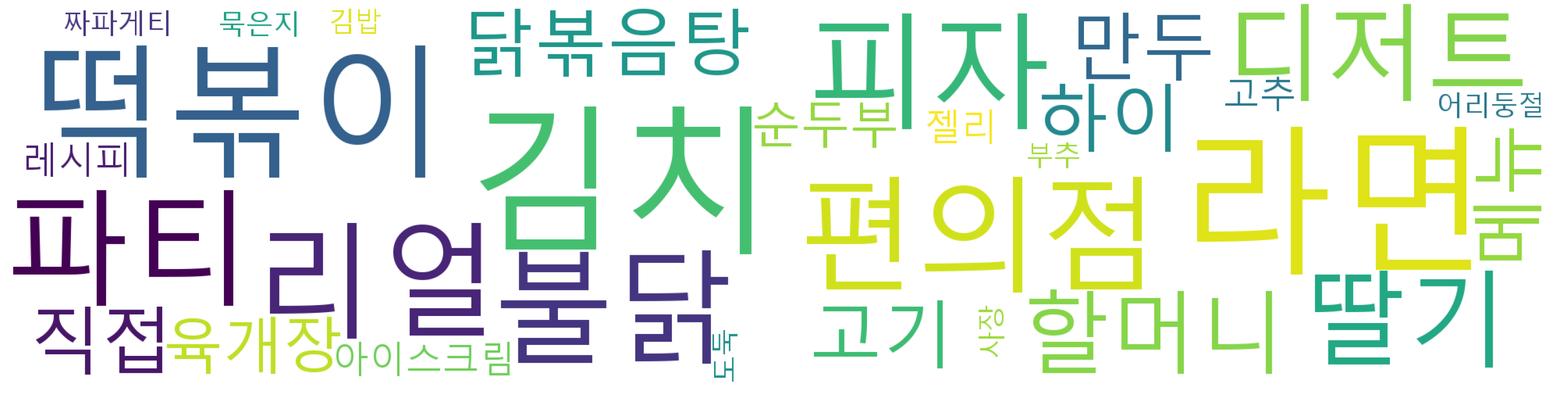