JTextArea
- 글자가 표시되는 다중 라인지역
- 사용자 편집 가능
- JTextComponent 상속
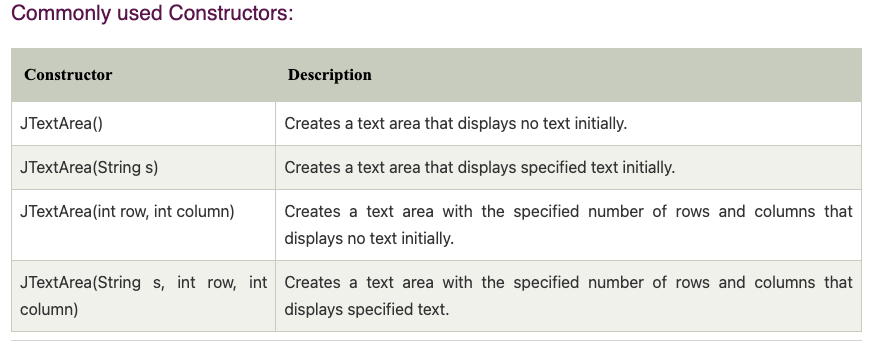
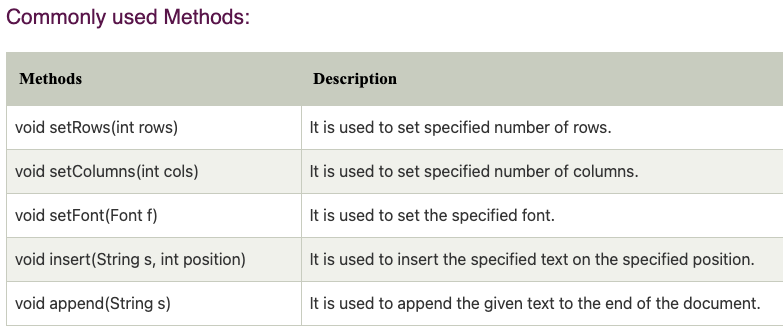
public static void main(String[] args){
JFrame f= new JFrame();
JTextArea area=new JTextArea("Welcome to javatpoint");
area.setBounds(10,30, 200,200);
f.add(area);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
}
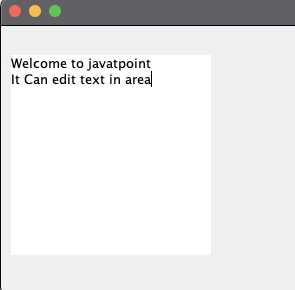
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TextAreaExample extends JFrame implements ActionListener {
JLabel l1,l2;
JTextArea area;
JButton b;
TextAreaExample(){
l1 = new JLabel();
l1.setBounds(50,25,100,30);
l2 = new JLabel();
l2.setBounds(160,25,100,30);
area = new JTextArea();
area.setBounds(25,75,250,200);
b= new JButton("Count Words");
b.setBounds(100,300,120,30);
b.addActionListener(this);
add(l1); add(l2); add(area); add(b);
setSize(450, 450);
setLayout(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
String text = area.getText();
String words[] = text.split("\\s");
l1.setText("Words: " + words.length);
l2.setText("Characters: "+text.length());
}
public static void main(String[] args){
new TextAreaExample();
}
}
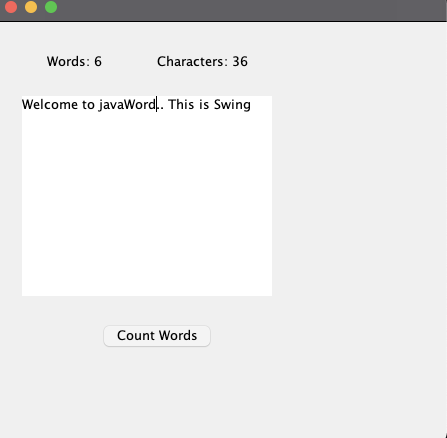
JPasswordField
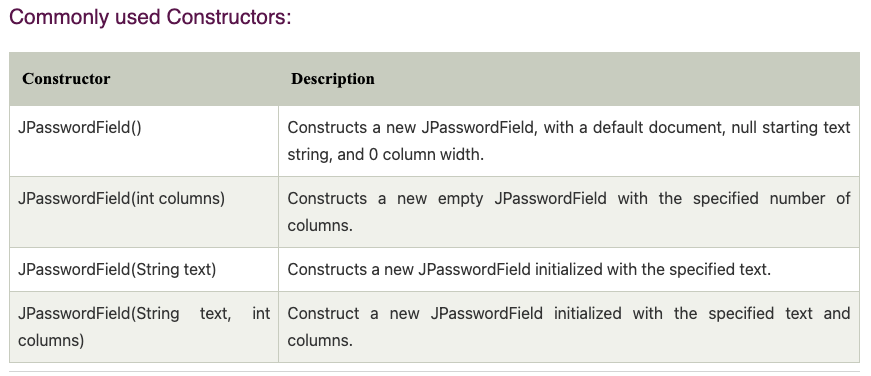
public static void main(String[] args){
JFrame f=new JFrame("Password Field Example");
JPasswordField value = new JPasswordField();
JLabel l1=new JLabel("Password:");
l1.setBounds(20,100, 80,30);
value.setBounds(100,100,100,30);
f.add(value); f.add(l1);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
}
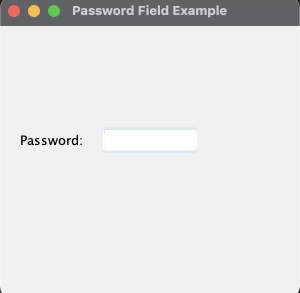
import javax.swing.*;
import java.awt.event.*;
public class PasswordFieldExample {
public static void main(String[] args){
JFrame f=new JFrame("Password Field Example");
JLabel label = new JLabel();
label.setBounds(20,150, 300,50);
JPasswordField value = new JPasswordField();
value.setBounds(100,75,100,30);
JLabel l1=new JLabel("Username:");
l1.setBounds(20,20, 80,30);
JLabel l2=new JLabel("Password:");
l2.setBounds(20,75, 80,30);
JButton b = new JButton("Login");
b.setBounds(100,120, 80,30);
JTextField text = new JTextField();
text.setBounds(100,20, 100,30);
f.add(value); f.add(l1); f.add(label); f.add(l2); f.add(b); f.add(text);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
b.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String data = "Username " +text.getText();
data += ", Password: " + new String(value.getPassword());
label.setText(data);
}
});
}
}
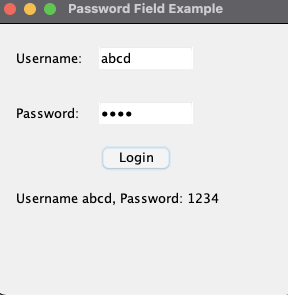
JCheckBox
- 체크박스를 만듬
- 옵션을 켜거나(true) 비활성화(false)하는 데 사용
- on -> off || off -> on , Toggle 기능을 함
- JToggleButton 을 상속함
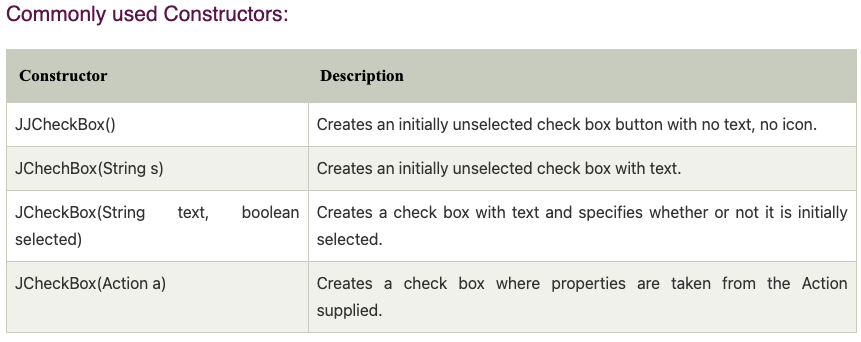
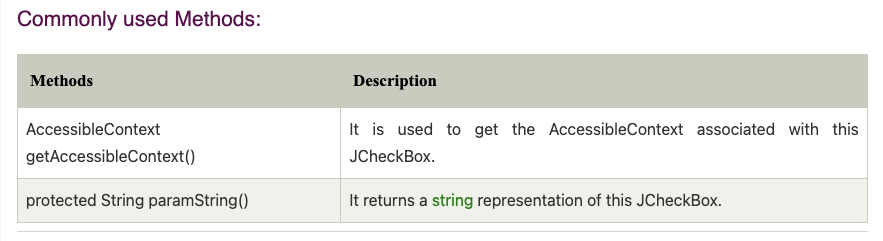
public class CheckBoxExample {
CheckBoxExample(){
JFrame f= new JFrame("CheckBox Example");
JCheckBox checkBox1 = new JCheckBox("male",true);
checkBox1.setBounds(100,100,100,50);
JCheckBox checkBox2 = new JCheckBox("female");
checkBox2.setBounds(100,150,100,50);
f.add(checkBox1); f.add(checkBox2);
f.setSize(400,400);
f.setLayout(null);
f.setVisible(true);
}
public static void main(String[]args){
new CheckBoxExample();
}
}
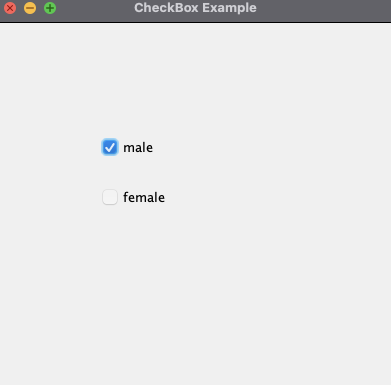
CheckBox Example with ItemListener
import javax.swing.*;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
public class CheckBoxExample {
CheckBoxExample(){
JFrame f= new JFrame("CheckBox Example");
final JLabel label = new JLabel();
label.setHorizontalAlignment(JLabel.CENTER);
label.setSize(400,100);
JCheckBox checkbox1 = new JCheckBox("C++");
checkbox1.setBounds(150,100, 100,50);
JCheckBox checkbox2 = new JCheckBox("Java");
checkbox2.setBounds(150,150, 100,50);
f.add(checkbox1); f.add(checkbox2); f.add(label);
checkbox1.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
label.setText("C++ Checkbox: " +(e.getStateChange()==1 ? "checked" : "unChecked"));
System.out.println(e.getStateChange());
}
});
checkbox2.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
label.setText("Java CheckBox: " + ( e.getStateChange()== 1 ?"checked" : "unChecked"));
}
});
f.setSize(400,400);
f.setLayout(null);
f.setVisible(true);
}
public static void main(String[]args){
new CheckBoxExample();
}
}
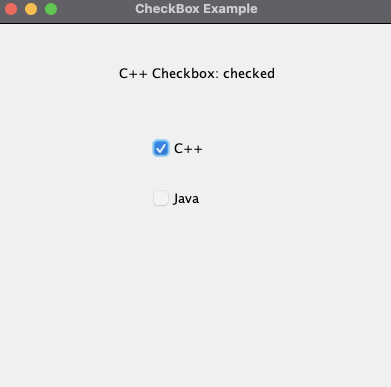
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class CheckBoxExample2 extends JFrame implements ActionListener {
JLabel l;
JCheckBox cb1, cb2, cb3;
JButton b;
CheckBoxExample2(){
l = new JLabel("Food Ordering System");
l.setBounds(50,50,300,20);
cb1=new JCheckBox("Pizza @ 100");
cb1.setBounds(100,100,150,20);
cb2=new JCheckBox("Burger @ 30");
cb2.setBounds(100,150,150,20);
cb3=new JCheckBox("Tea @ 10");
cb3.setBounds(100,200,150,20);
b=new JButton("Order");
b.setBounds(100,250,80,30);
b.addActionListener(this);
add(l);add(cb1);add(cb2);add(cb3);add(b);
setSize(400,400);
setLayout(null);
setVisible(true);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
float amount = 0;
String msg = "";
if(cb1.isSelected()){
amount+=100;
msg="Pizza: 100\n";
}
if(cb2.isSelected()){
amount+=30;
msg+="Burger: 30\n";
}
if(cb3.isSelected()){
amount+=10;
msg+="Tea: 10\n";
}
msg+="-----------------\n";
JOptionPane.showMessageDialog(this, msg+"Total: "+ amount);
}
public static void main(String[] args){
new CheckBoxExample2();
}
}
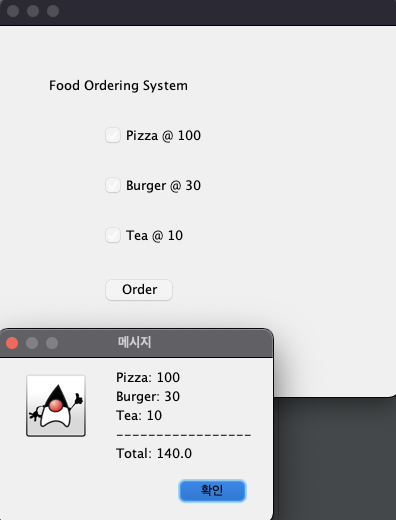
- 라이오 버튼을 만들때 사용
- 여러개의 옵션 중, 한개를 선택할때
- 시험, 퀴즈에서 주로 사용
- JToggleButton 상속
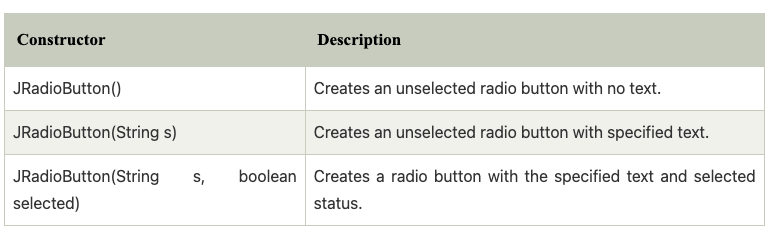
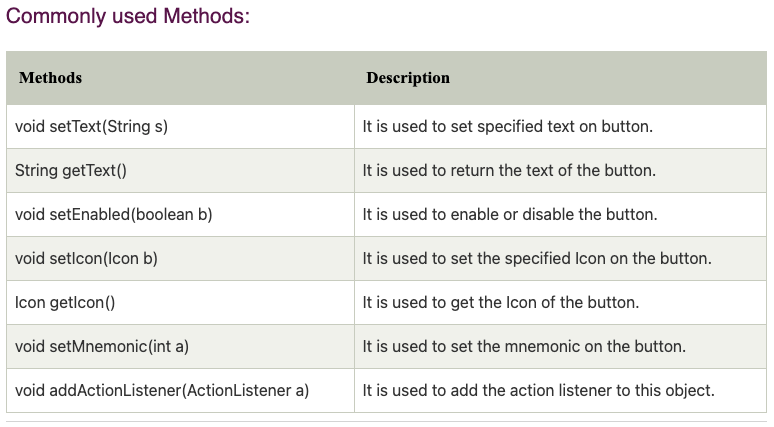
import javax.swing.*;
public class RadioButtonExample {
JFrame f;
RadioButtonExample(){
f= new JFrame();
JRadioButton r1 = new JRadioButton("A) Male");
JRadioButton r2 = new JRadioButton("A) Female");
r1.setBounds(75,50,100,30);
r2.setBounds(75,100,100,30);
ButtonGroup bg =new ButtonGroup();
bg.add(r1); bg.add(r2);
f.add(r1); f.add(r2);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
}
public static void main(String[] args) {
new RadioButtonExample();
}
}
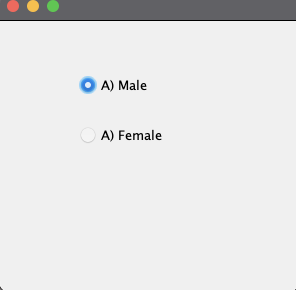
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class RadioButtonExample extends JFrame implements ActionListener {
JRadioButton rb1,rb2;
JButton b;
RadioButtonExample(){
rb1=new JRadioButton("Male");
rb1.setBounds(100,50,100,30);
rb2=new JRadioButton("Female");
rb2.setBounds(100,100,100,30);
ButtonGroup bg=new ButtonGroup();
bg.add(rb1);bg.add(rb2);
b=new JButton("click");
b.setBounds(100,150,80,30);
b.addActionListener(this);
add(rb1);add(rb2);add(b);
setSize(300,300);
setLayout(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(rb1.isSelected()){
JOptionPane.showMessageDialog(this,"You are Male");
}
if(rb2.isSelected()){
JOptionPane.showMessageDialog(this,"You are Female");
}
}
public static void main(String[] args) {
new RadioButtonExample();
}
}
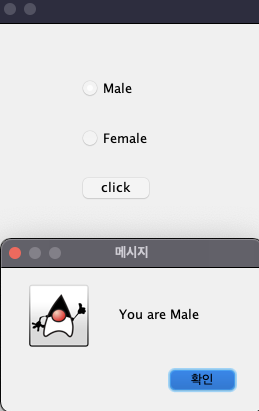
JComboBox
- Choice Class의 객체는 선택들의 팝업 매뉴를 보여주는데 사용하다.
- JConponent 를 상속받는다.
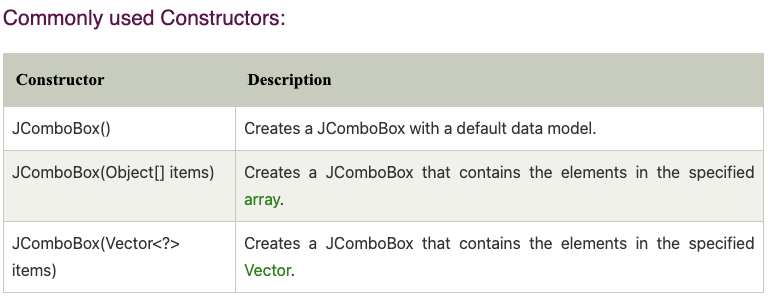
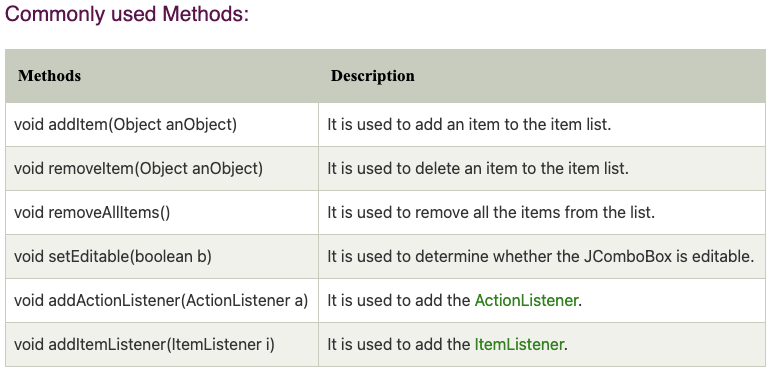
public class ComboBoxExample {
JFrame j ;
ComboBoxExample(){
j = new JFrame("ComboBox Example");
String country[] = {"Korea", "Japan","India", "Aus", "U.S.A", "England"};
JComboBox cb = new JComboBox(country);
cb.setBounds(50,50,90,20);
j.add(cb);
j.setLayout(null);
j.setSize(400,500);
j.setVisible(true);
}
public static void main(String[] args) {
new ComboBoxExample();
}
}
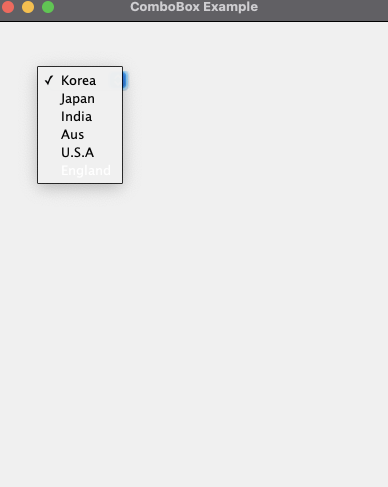
Java JComboBox Example with ActionListener
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ComboBoxExample {
JFrame j ;
ComboBoxExample(){
j = new JFrame("ComboBox Example");
final JLabel label = new JLabel();
label.setHorizontalAlignment(JLabel.CENTER);
label.setSize(400,100);
JButton b=new JButton("Show");
b.setBounds(200,100,75,20);
String languages[]={"C","C++","C#","Java","PHP"};
final JComboBox cb=new JComboBox(languages);
cb.setBounds(50, 100,90,20);
j.add(cb); j.add(label); j.add(b);
j.setLayout(null);
j.setSize(350,350);
j.setVisible(true);
b.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String data = "Programming language Selected: " + cb.getItemAt(cb.getSelectedIndex());
System.out.println(cb.getSelectedIndex());
label.setText(data);
}
});
}
public static void main(String[] args) {
new ComboBoxExample();
}
}
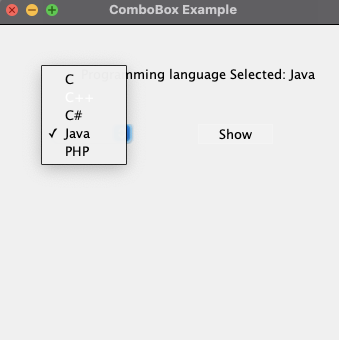
JTable
- 표로 된 폼의 데이터를 보여주는데 사용된다.
- 행과 열로 구성되어 있다.
public class TableExample {
JFrame f;
TableExample(){
f=new JFrame();
String data[][]={ {"101","Amit","670000"},
{"102","Jai","780000"},
{"101","Sachin","700000"}};
String column[]={"ID","NAME","SALARY"};
JTable jt=new JTable(data,column);
jt.setBounds(30,40,200,300);
JScrollPane sp=new JScrollPane(jt);
f.add(sp);
f.setSize(300,400);
f.setVisible(true);
}
public static void main(String[] args) {
new TableExample();
}
}
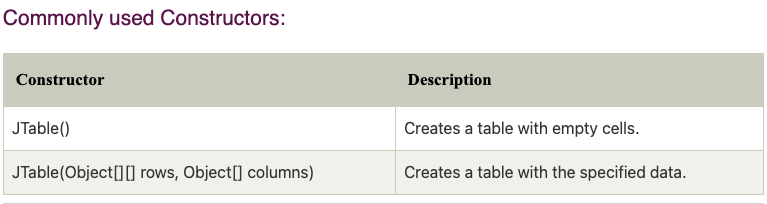
JList
- text item 의 list를 보여준다.
- 유저가 하나 이상의 아이템을 선택할 수 있게 아이템 리스트를 설정할 수 있다.
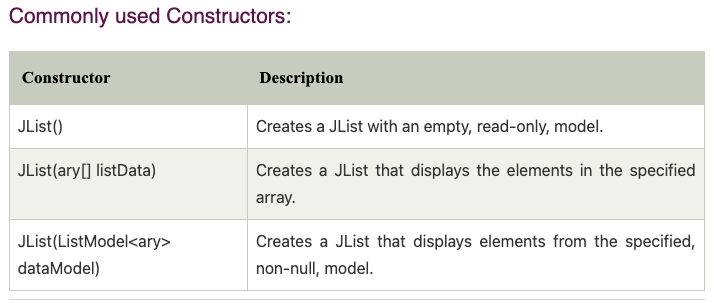
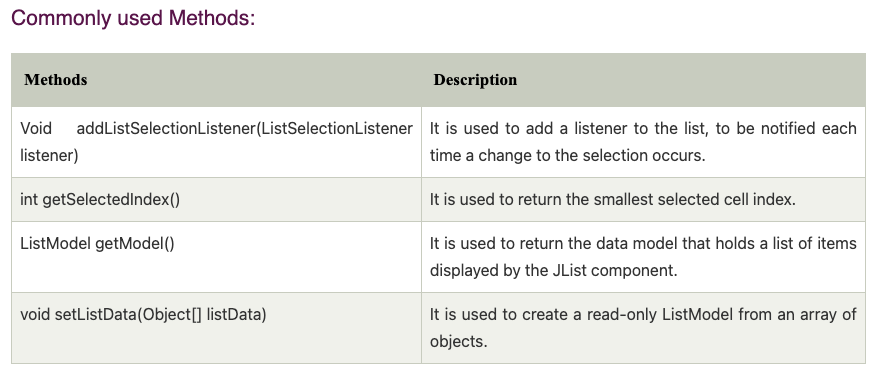
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ListExample {
ListExample(){
JFrame f= new JFrame();
final JLabel label = new JLabel();
label.setSize(500,100);
JButton b=new JButton("Show");
b.setBounds(200,150,80,30);
final DefaultListModel<String> l1 = new DefaultListModel<>();
l1.addElement("C");
l1.addElement("C++");
l1.addElement("Java");
l1.addElement("PHP");
final JList<String> list1 = new JList<>(l1);
list1.setBounds(100,100, 75,75);
DefaultListModel<String> l2 = new DefaultListModel<>();
l2.addElement("Turbo C++");
l2.addElement("Struts");
l2.addElement("Spring");
l2.addElement("YII");
final JList<String> list2 = new JList<>(l2);
list2.setBounds(100,200, 75,75);
f.add(list1); f.add(list2); f.add(b); f.add(label);
f.setSize(450,450);
f.setLayout(null);
f.setVisible(true);
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String data = "";
if (list1.getSelectedIndex() != -1) {
data = "Programming language Selected: " + list1.getSelectedValue();
label.setText(data);
}
if(list2.getSelectedIndex() != -1){
data += ", FrameWork Selected: ";
for(Object frame :list2.getSelectedValues()){
data += frame + " ";
}
}
label.setText(data);
}
});
}
public static void main(String args[])
{
new ListExample();
}
}
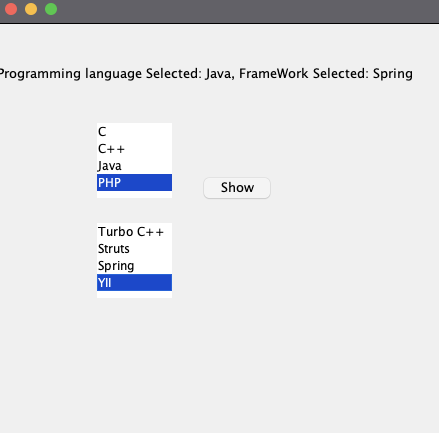
JOptionPane
- The JOptionPane class is used to provide standard dialog boxes such as message dialog box, confirm dialog box and input dialog box. These dialog boxes are used to display information or get input from the user. The JOptionPane class inherits JComponent class.
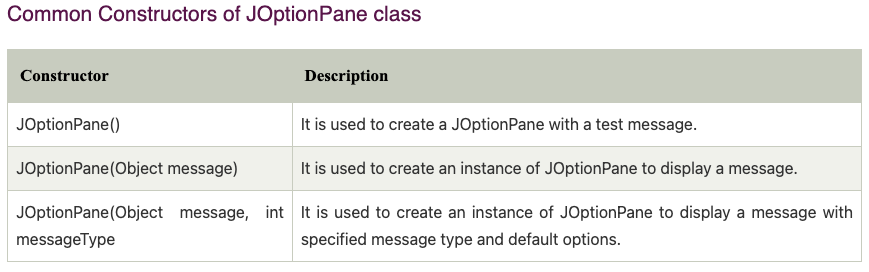
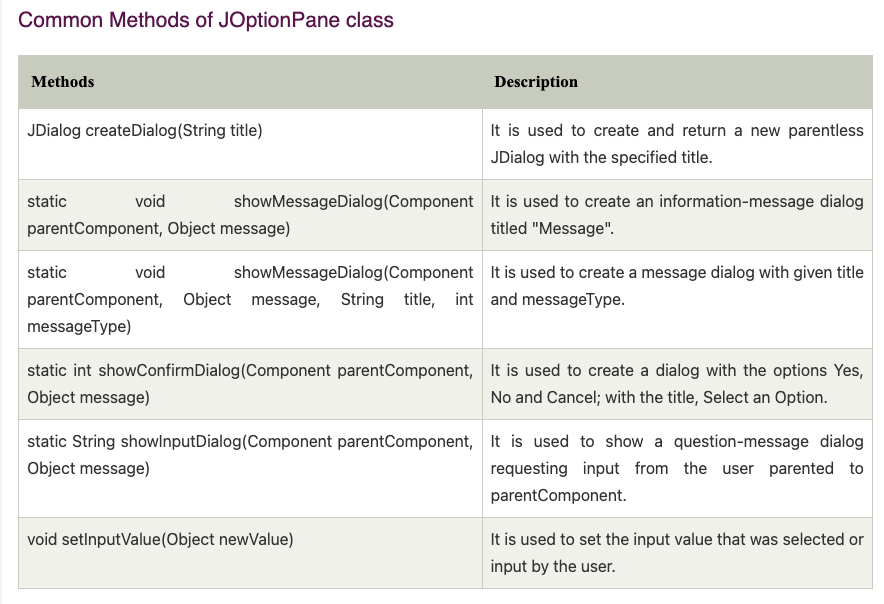
import javax.swing.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class OptionPaneExample extends WindowAdapter {
JFrame f;
OptionPaneExample(){
f=new JFrame();
f.addWindowListener(this);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);
}
public void windowClosing(WindowEvent e){
int a = JOptionPane.showConfirmDialog(f,"Are you sure?");
if(a==JOptionPane.YES_OPTION){
System.out.println(a);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
else if(a == JOptionPane.NO_OPTION){
System.out.println(a);
}
else if(a == JOptionPane.CANCEL_OPTION){
System.out.println(a);
}
}
public static void main(String[] args) {
new OptionPaneExample();
}
}
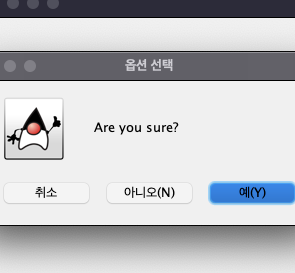
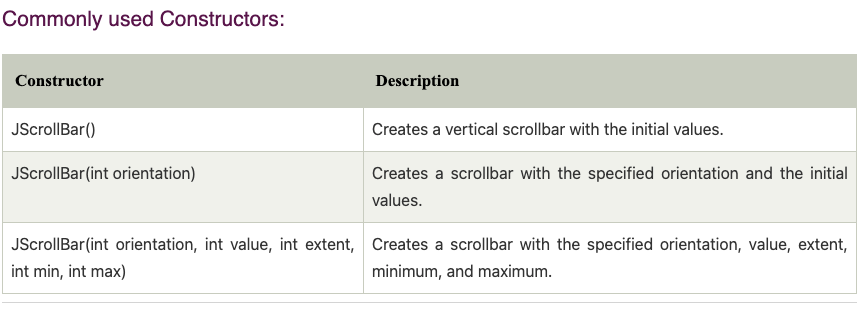
import javax.swing.*;
import java.awt.event.AdjustmentEvent;
import java.awt.event.AdjustmentListener;
public class ScrollBarExample {
ScrollBarExample(){
JFrame j = new JFrame("Scrollbar Example");
final JLabel label = new JLabel();
label.setHorizontalAlignment(JLabel.CENTER);
label.setSize(400,100);
final JScrollBar s = new JScrollBar();
s.setBounds(100,100,50,100);
j.add(s); j.add(label);
j.setSize(400,400);
j.setLayout(null);
j.setVisible(true);
s.addAdjustmentListener(new AdjustmentListener() {
@Override
public void adjustmentValueChanged(AdjustmentEvent e) {
label.setText("Vertical Scrollbar value is: "+ s.getValue());
}
});
}
public static void main(String[] args){
new ScrollBarExample();
}
}
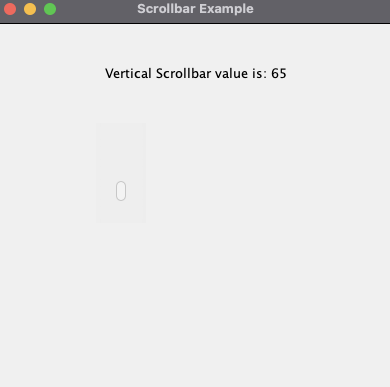
JMenuBar, JMenu and JMenuItem
- JMenuBar: 윈도우, 프레임에 매뉴바를 보여주는데 사용됨(여러개의 매뉴를 갖음)
- JMenu: 매뉴바에 나타나는 풀다운 매뉴 컴포넌트
- JMenuItem: 레이블이 지정된 간단한 메뉴 항목을 추가, 메뉴에 사용된 항목은 JMenuItem 또는 해당 하위 클래스에 속해야 함
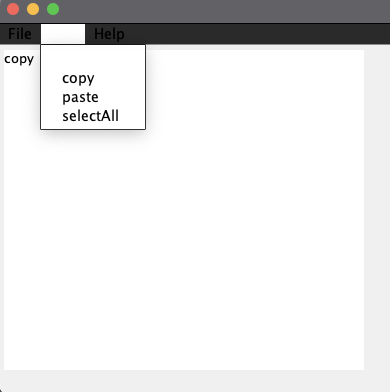
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MenuExample implements ActionListener {
JFrame f;
JMenuBar mb;
JMenu file,edit, help;
JMenuItem cut,copy,paste, selectAll;
JTextArea ta;
MenuExample(){
f= new JFrame();
cut=new JMenuItem("cut");
copy=new JMenuItem("copy");
paste=new JMenuItem("paste");
selectAll=new JMenuItem("selectAll");
cut.addActionListener(this);
copy.addActionListener(this);
paste.addActionListener(this);
selectAll.addActionListener(this);
mb=new JMenuBar();
file=new JMenu("File");
edit=new JMenu("Edit");
help=new JMenu("Help");
edit.add(cut); edit.add(copy); edit.add(paste); edit.add(selectAll);
mb.add(file);mb.add(edit);mb.add(help);
ta=new JTextArea();
ta.setBounds(5,5,360,320);
f.add(ta);
f.setJMenuBar(mb);
f.setLayout(null);
f.setSize(400,400);
f.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==cut)
ta.append(cut.getText());
if(e.getSource()==paste)
ta.append(paste.getText());
if(e.getSource()==copy)
ta.append(copy.getText());
if(e.getSource()==selectAll)
ta.append(selectAll.getText());
}
public static void main(String[] args) {
new MenuExample();
}
}