✏️ 형변환
명시적 형변환
자료형 함수를 이용해 명시적으로 형 변환을 수행
console.log(String(123));
console.log(String(1/0));
console.log(String(-1/0));
console.log(String(NaN));
console.log(String(true));
console.log(String(false));
console.log(String(undefined));
console.log(String(null));
console.log(String("hello world"));
console.log(Number(""));
console.log(Number("123"));
console.log(Number("hello world"));
console.log(Number("123hello"));
console.log(Number(true));
console.log(Number(false));
console.log(Number(null));
console.log(Number(undefined));
console.log(parseInt("123.123"));
console.log(parseFloat("123.123"));
console.log(Boolean(""));
console.log(Boolean("123"));
console.log(Boolean("hello"));
console.log(Boolean("0"));
console.log(Boolean(0));
console.log(Boolean(123));
console.log(Boolean(NaN));
console.log(Boolean(null));
console.log(Boolean(undefined));
✏️ 연산자
연산자의 종류
1. 단항 연산자
부호연산자: +, -
증감연산자: ++, --
논리연산자: !
비트연산자: ~
2. 이항 연산자
산술연산자
+, -, *, /, %
대입연산자
=, +=, -=
비교연산자
==, !=
논리연산자
&&, ||
2.1 산술 연산자 우선순위
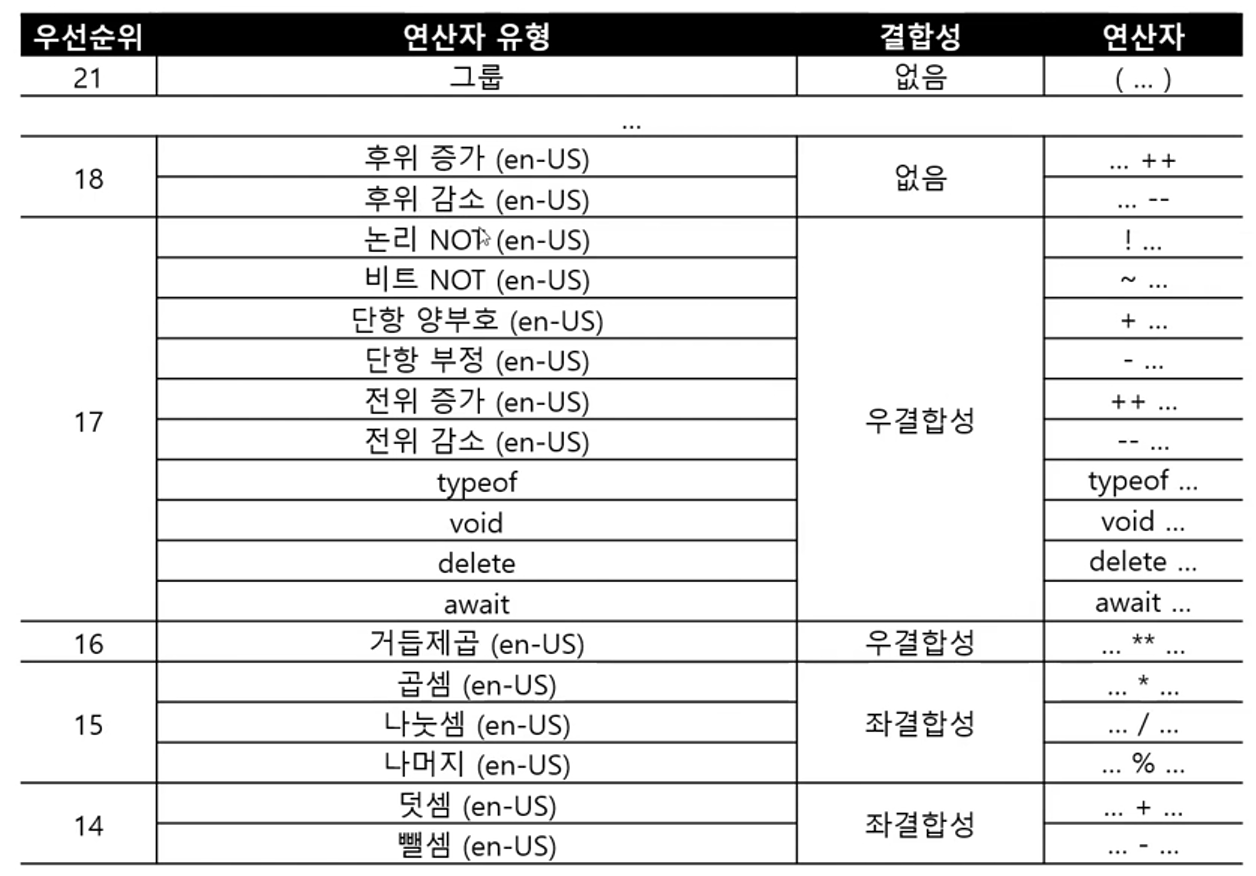
2.2 증가 감소 연산자
증가 감소 연산자를 살펴보면 후위연산자일 경우 변수에 들어 있는 값을 먼저 할당해준 뒤에 변수의 값에
들어 있는 값을 변경시켜준다.
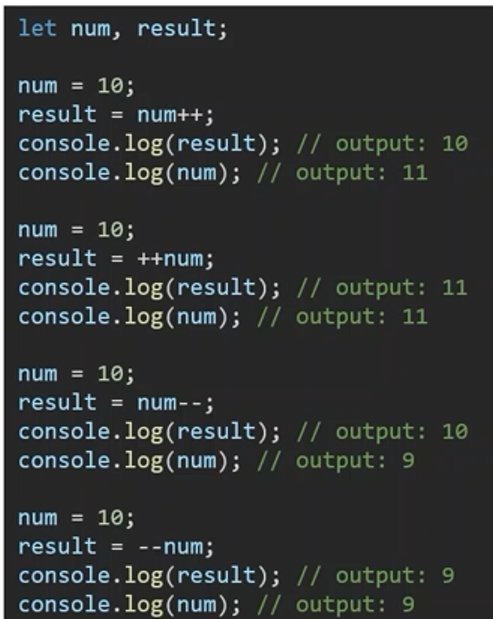
3. 삼항 연산자
(조건식) ? 참일 경우의 식 : 거짓일 경우의 식
✏️ 비교 연산자
아래와 같이 '=='으로 값을 비교할 때 javascript 특성상 자료형이 좌측은 Number 우측은 String으로
서로 다른 자료형이더라도 값이 같기 때문에 true이다.
5 == '5'
console.log("Z" > "A")
console.log("Hello" < "Hez")
console.log("Hello" > "Helloz")
console.log("5" < 10);
console.log(true == 1);
console.log(false != 123);
console.log(true === 1);
✏️ Scope
변수 혹은 상수에 접근할 수 있는 범위
Global Scope
전역에 선언되어 어디에서도 접근 가능하다.
Local Scope
지역에 선언되어 어디에서도 접근 가능하다.
예제_1)
let x = 1;
let y = 2;
console.log(x);
console.log(y);
{
let x = 3;
let y = 4;
console.log(x);
console.log(y);
}
예제_2) local scope 안의 local scope
let x = 1;
let y = 2;
console.log(x);
console.log(y);
{
let x = 3;
let y = 4;
console.log(x);
console.log(y);
{
let x = 5;
let y = 6;
console.log(x);
console.log(y);
}
✏️ 조건문
논리적 비교를 할 때 사용함.
조건식은 아래와 같이 여러개의 구조를 가질 수 있고 필요한 알고리즘을 작성하는데 사용자 방식으로
만들어서 작성하면 된다.
1. if만 있는 경우
if (condition) {
content
}
2. if와 else가 있는 경우
if (condition_1) {
content
} else {
content
}
3. if와 else if else가 있는 경우
if (condition_1) {
content
} else if (condition_2) {
content_2
} else (condition_3) {
content_3
}
4. if와 else if(if, else if, else), else if, else가 있는 경우
if (condition_1) {
content
} else if (condition_2) {
if(condition_3) {
content
} else if (condition_4) {
content
} else {
content
}
else if (condition_5) {
content
}
else (condition_5) {
content
}
}
삼항 연산자
변수 = (조건식) ? 참일 때의 값 : 거짓일 때의 값
let res = 10 > 5 ? "true" : "false"
switch
표현식을 평가하여 그 값이 일치하는 case문을 실행하는 조건문 switch() 괄호 안에 들어가는 조건에
맞는 값에 대응 하는 case로 가서 안의 해당 지점부터 break; 구문을 만날 때 까지 아래 계속해서 순
차적으로 code를 실행한다.
ley day_number = 1;
let day = "";
switch (day_number) {
case 0:
day = "Sunday";
break;
case 1:
day = "Monday";
break;
case 2:
day = "Tue";
break;
case 3:
day = "Wed";
break;
case 4:
day = "Thu";
break;
case 5:
day = "Fri";
break;
case 6:
day = "Sat";
break;
default:
day = "error";
break;
}
✏️ 반복문
선언문, 조건문, 증감문 형태로 이루어진 반복문
조건문이 fail이 되기 전까지 코드 블록을 계속적으로 반복 수행
선언문, 조건문, 증감문 자리에 공백 입력 가능
Init Expression (선언문)
Test Expression (조건문)
Update Expression (증감문)
for (Init Expression; Test Expression; Update Expression) {
Statement Block ...
};
반복문 for(확장)
1) for .. in
객체의 key, value 형태를 반복하여 수행하는데 최적화 된 유형
첫번째부터 마지막까지, 객체의 키 개수만큼 반복
const person = {
name: "john",
age: 27,
weight: 72
}
text = '';
for (key in person) {
text += person[key];
}
console.log(text);
2) for .. of
객체의 Symbol.iterator 속성에 특정 형태의 함수가 들어있다면,
이를 반복 가능한 객체(iterable object) 혹은 줄여서 iterable이라 부르고,
해당 객체는 iterable protocol을 만족한다고 한다.
let word = "apple";
let text = "";
for (alph of word) {
text += alpha;
}
console.log(text)
const characters = [...'hello'];
console.log('characters: ', characters);
const [c1, c2] = 'hello';
console.log(c1, c2);
console.log(Array.from('hello'));
반복문 while
1. while 반복문
while (Test Expression) {
content
}
while 반복문의 경우 Test Exression 조건을 만족하면 content 부문을 수행하고 조건이 맞지 않을
경우 다시 탈출하게 된다.
2. do ... while 반복문
do {
block
} while (Test Expression);
do ... while 반복문의 경우 block 내용을 먼저 수행 한후 표현식에 대한 조건이 맞으면 다시 black
을 수행하게 되고 그렇지 않을 경우 종료된다.
반복문 제어
1. break
조건문과 함께 쓰이는 keyword 이다. 아래 구조가 기본 단위이다. break를 만날 경우 반복문에서
탈출하는 역할을 한다.
1.1 기본구조
if (condition to break) {
break;
}
1.2 반복문과 함께 쓰일 때
반복문과 함께 쓰일 경우 조건에 부합할 경우 while문 에서 빠져나온다
while(condition) {
code_1
if(condition to break) {
break;
}
code_2
}
2. continue
조건문과 함께 쓰이는 keyword 이다. 아래 구조가 기본 단위이다.
2.1 기본구조
if (condition to continue) {
continue;
}
2.2 반복문과 함께 쓰일 때 조건에 부합할 때 code_2 부분을 실행하지 않고 다시 반복문안에 있는
code를 수행한다. 언제까지? 반복문에 condition에 부합하지 않을 때 까지
while(condition) {
code_1
if (condition to continue) {
continue;
}
code_2
}
3. Label 예제
코드 블럭에서 특정 반복문의 부분의 반복을 배제시키고 싶을 때 사용한다.
3.1 기본구조
end: (code_block)
break end;
3.2 예시)
end: for(let i = 0; i < 3; i++) {
for(let j = 0; j < 3; j++) {
console.log(i + "*" + j + "=" + i*j);
break end;
}
}