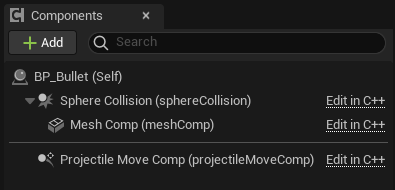
📄 Bullet.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "Bullet.generated.h"
UCLASS()
class MYTPS_API ABullet : public AActor
{
GENERATED_BODY()
public:
ABullet();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
UPROPERTY(EditAnywhere)
class USphereComponent* sphereCollision;
UPROPERTY(EditAnywhere)
class UStaticMeshComponent* meshComp;
UPROPERTY(EditAnywhere)
class UProjectileMovementComponent* projectileMoveComp;
};
📄 Bullet.cpp
#include "Bullet.h"
#include "Components/SphereComponent.h"
#include "GameFramework/ProjectileMovementComponent.h"
ABullet::ABullet()
{
PrimaryActorTick.bCanEverTick = true;
sphereCollision = CreateDefaultSubobject<USphereComponent>(TEXT("sphereCollision"));
sphereCollision->SetSphereRadius(10.f);
sphereCollision->SetCollisionProfileName(TEXT("BlockAll"));
RootComponent = sphereCollision;
meshComp = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("meshComp"));
ConstructorHelpers::FObjectFinder<UStaticMesh> tempMesh(TEXT("StaticMesh'/Engine/BasicShapes/Sphere.Sphere'"));
if (tempMesh.Succeeded())
{
meshComp->SetStaticMesh(tempMesh.Object);
meshComp->SetRelativeScale3D(FVector(0.2f));
meshComp->SetCollisionProfileName(TEXT("NoCollision"));
}
meshComp->SetupAttachment(RootComponent);
projectileMoveComp = CreateDefaultSubobject<UProjectileMovementComponent>(TEXT("projectileMoveComp"));
projectileMoveComp->SetUpdatedComponent(sphereCollision);
projectileMoveComp->InitialSpeed = 5000;
projectileMoveComp->MaxSpeed = 5000;
projectileMoveComp->bShouldBounce = true;
projectileMoveComp->Bounciness = 0.3f;
}
void ABullet::BeginPlay()
{
Super::BeginPlay();
SetLifeSpan(2.f);
}
void ABullet::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}