활용
사전준비
랜덤으로 바뀔 버튼과 배경화면 생성
벤트를 적용할 영역(color_bg, btn)
<div id="color_bg">
<button id="btn" type="button">클릭</button>
</div>
#color_bg {
width: 500px;
height: 500px;
background-color: black;
}
#btn {
width: 100px;
height: 50px;
line-height: 50px;
background-color: purple;
color: #ffffff;
font-size: 20px;
}
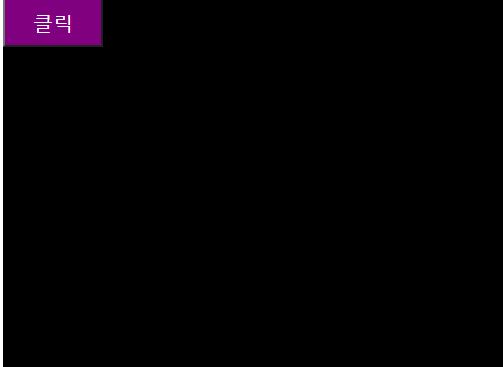
5개의 색상 배열 만들기
var colors = [
'yellow',
'green',
'pink',
'#dc143c',
'rgba(123, 123, 123, 0.5)'
];
자바스크립트로 id와 class에 접근
변수(여기서는 bg)를 만들고 document.getElementById() 안에 접근하고자 하는 id값을 넣으면 됨
var bg = document.getElementById('color_bg');
console.log(bg);
//추가
var btn = document.getElementById('btn');
console.log(btn);

이 구조도 잘보면 student.sum(10, 20) 구조랑 비슷함
document도 객체이고 getElementById는 객체 안에 들어있는 메서드 인것이다
document라는 객체는 html 문서에 영향력을 발휘하는 객체이다
초반부에 말한 자바스크립트 3가지 영역 중에 지금까지 배운 이론적인 내용은 자바스크립트 코어이고 document라는 객체가 들어가는 내용은 클라이언트 측 자바스크립트이다.
html문서를 제어하고 브라우저를 제어하는 역할을 담당, 그러한 역할을 담당하는 자바스크립트 영역이 바로 클라이언트측 자바스크립트다
만들기 시작
클릭했을때 라는 행동 자체를 버튼 태그안에 적용시키기
addEventListener 메서드를 활용해서 크게 두가지 인수를 전달받게 된다
1. 문자열로 이벤트의 종류
첫번째에 들어가는 인수는 정해져있다. (click, mouseover...)
2. 함수
함수이름이 없는 함수를 익명함수라 한다
우리가 가져온 btn이라는 영역 전체도 결과적으로 객체라고 할 수 있다.
그렇기 때문에 지금과 같은 구조가 나오는 것
console.log, student.sum(10,20)
즉 getElementById로 가져오게되면 가져온 영역도 자바스크립트는 객체로 인식한다.
클릭시 함수의 중갈호 안에 코드는 함수를 호출해야지만 실행이 된다라고 배움 호출하지 않았는데 결과 가 나옴
그 이유는 클릭했을때 두번째로 만들어 놓은 함수 (function(){ console.log("Hello");})를 호출해라 라고 addEventListenenr에서 정의를 해둔 것이다. 그래서 호출하지 않아도 된다(불필요하다)
클릭을 할때 addEventListener로 인해서 2번째 인수로 전달된 함수가 호출이 되면서 중갈호 안에 있는 코드가 실행되는것
특정조건하에서 호출기호 없이 호출이 되는 함수를 콜백함수 라고 한다.
btn.addEventListener('click', function(){
console.log("Hello");
})

1 ~ 6 숫자를 랜덤하게 가져오기
Math는 숫자를 제어할때 사용되는 객체
Math 객체 안에 있는 random 메서드를 사용
0 ~ < 1 사이 실수형태로 표현 (0은 되지만 1은 절대 될 수 없다)
6을 곱하면 0 ~ 5.99999 안에서 랜덤으로 가져오게 된다
console.log(Math.random());
console.log(Math.random() * 6);

Math.floor
소수점 이하 숫자는 다 버릴 때 사용
console.log(Math.floor(Math.random() * 6));
console.log(Math.floor(Math.random() * 6) + 1);

간단한 정리
// Math.random() : 0 ~ < 1 실수형태로 표현 : 0 ~ 0.999999~~
// Math.random() * 6 : 0 ~ 5.999999~~~
// Math.floor(Math.random() * 6) : 0 ~ 5
// Math.floor(Math.random() * 6) + 1 : 1 ~ 6
공식 활용하여 배열 안의 데이터 가져오기(랜덤)
btn.addEventListener('click', function(){
var random = Math.floor(Math.random() * 5)
console.log(random);
})
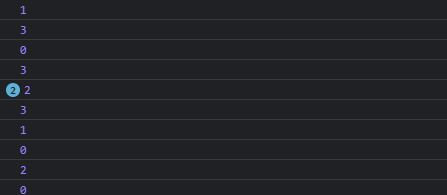
배열 안의 데이터 접근 공식과 결합
btn.addEventListener('click', function(){
var random = Math.floor(Math.random() * 5)
console.log(random);
console.log(colors[random]);
})
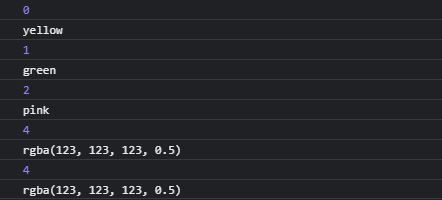
자바스크립트에서 css 적용하기
배경색을 적용할 영역(bg)을 지정
자바스크립트에서 css 적용하기 위해선 bg 객체 안에 있는 style이라는 property에 접근해야 한다.
style에 접근해서 안에 있는 background에 color[radom];를 적용
btn.addEventListener('click', function(){
var random = Math.floor(Math.random() * 5)
bg.style.backgroundColor = colors[random];
})
완성
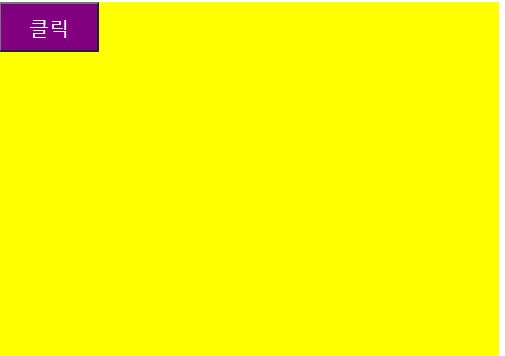