📕 문제
📌 링크
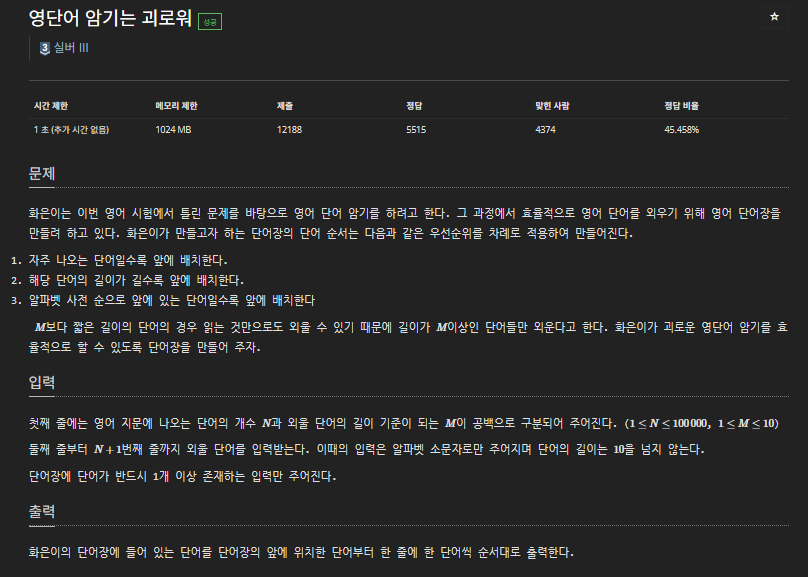
📗 접근 방식
- 딕셔너리 자료구조를 이용하여 단어를 저장한다.
- 단어가 딕셔너리에 저장되어 있지 않다면 딕셔너리에 저장
- 딕셔너리의 해당 단어 요소의 Value를 1증가
- 정렬
- 단어가 호출된 수가 높은것 우선 (OrderByDescending(x => x.Value))
- 단어의 길이가 긴것 우선 (ThenByDescending(x => x.Key.Length).ThenBy(x => x.Key))
- 단어 알파벳 순서 우선 (ThenBy(x => x.Key))
- 정렬시킨 자료구조를 foreach를 사용해 탐색하며 출력
📘 코드
using System.Text;
namespace BOJ_20920
{
class Program
{
static void Main()
{
using StreamReader sr = new StreamReader(new BufferedStream(Console.OpenStandardInput()));
using StreamWriter sw = new StreamWriter(new BufferedStream(Console.OpenStandardOutput()));
Dictionary<string, int> dict = new Dictionary<string, int>();
StringBuilder sb = new StringBuilder();
int[] inputs = Array.ConvertAll(sr.ReadLine().Split(), int.Parse);
int n = inputs[0];
int m = inputs[1];
for (int i = 0; i < n; i++)
{
string s = sr.ReadLine();
if (s.Length < m) continue;
dict.TryAdd(s, 0);
dict[s]++;
}
var sorted = dict.OrderByDescending(x => x.Value).ThenByDescending(x => x.Key.Length).ThenBy(x => x.Key).ToDictionary(x=>x.Key,x=>x.Value);
foreach (var item in sorted)
{
sb.AppendLine(item.Key);
}
sw.Write(sb);
}
}
}
📙 오답노트
- 단어의 개수와 단어 길이를 정렬할 때 오름차순으로 정렬시켰다.
- 단어를 알파벳순으로 정렬시켜야하는데 x.Key로 선언하지 않고 x로 선언하였다.
- StringBuilder를 사용하지 않고 Console을 이용해 출력하였다. (=> 시간초과)
📒 알고리즘 분류
자료 구조
문자열
정렬
해시를 사용한 집합과 맵
트리를 사용한 집합과 맵