📕 문제
📌 링크
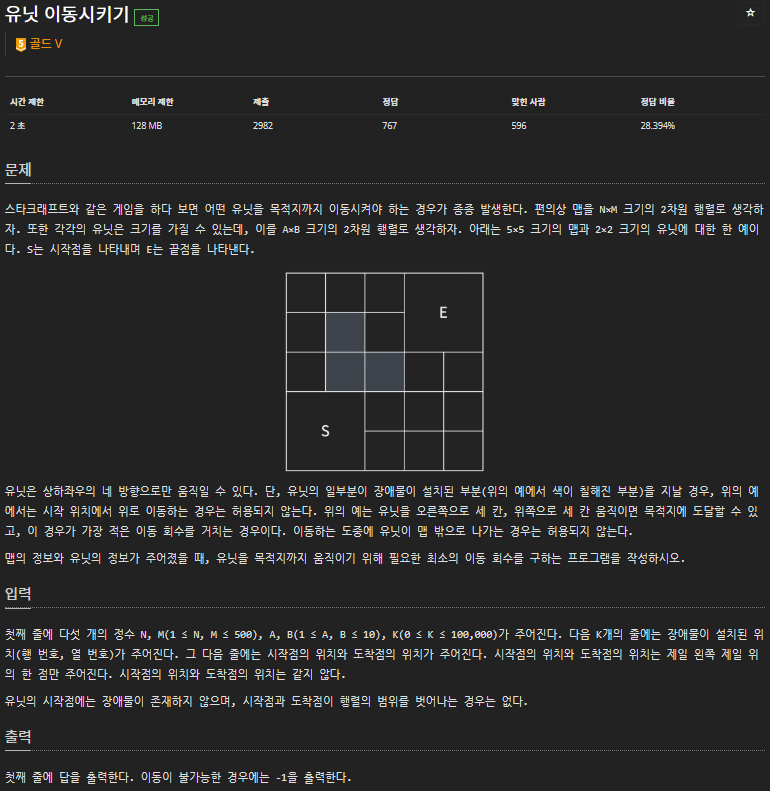
📘 코드
using System;
using System.Collections.Generic;
namespace BOJ
{
class Point
{
public int X { get; set; }
public int Y { get; set; }
public Point(int x, int y)
{
X = x;
Y = y;
}
}
class No_2194
{
static void Main(string[] args)
{
string[] input = Console.ReadLine().Split();
int N = int.Parse(input[0]);
int M = int.Parse(input[1]);
int A = int.Parse(input[2]);
int B = int.Parse(input[3]);
int K = int.Parse(input[4]);
bool[,] obstacleMap = new bool[N + 1, M + 1];
for (int i = 0; i < K; i++)
{
string[] obstacleInput = Console.ReadLine().Split();
int row = int.Parse(obstacleInput[0]);
int col = int.Parse(obstacleInput[1]);
obstacleMap[row, col] = true;
}
string[] startInput = Console.ReadLine().Split();
int startRow = int.Parse(startInput[0]);
int startCol = int.Parse(startInput[1]);
string[] endInput = Console.ReadLine().Split();
int endRow = int.Parse(endInput[0]);
int endCol = int.Parse(endInput[1]);
int[,] visited = new int[N + 1, M + 1];
for (int i = 1; i <= N; i++)
{
for (int j = 1; j <= M; j++)
{
visited[i, j] = -1;
}
}
Queue<Point> queue = new Queue<Point>();
queue.Enqueue(new Point(startRow, startCol));
visited[startRow, startCol] = 0;
int[] dx = { -1, 0, 1, 0 };
int[] dy = { 0, 1, 0, -1 };
while (queue.Count > 0)
{
Point current = queue.Dequeue();
for (int dir = 0; dir < 4; dir++)
{
int nx = current.X + dx[dir];
int ny = current.Y + dy[dir];
if (nx >= 1 && nx + A - 1 <= N && ny >= 1 && ny + B - 1 <= M)
{
bool isValid = true;
for (int i = nx; i < nx + A; i++)
{
for (int j = ny; j < ny + B; j++)
{
if (obstacleMap[i, j])
{
isValid = false;
break;
}
}
if (!isValid)
{
break;
}
}
if (isValid && visited[nx, ny] == -1)
{
visited[nx, ny] = visited[current.X, current.Y] + 1;
queue.Enqueue(new Point(nx, ny));
}
}
}
}
int result = visited[endRow, endCol];
Console.WriteLine(result);
}
}
}
📙 오답노트
📒 알고리즘 분류
개발자로서 배울 점이 많은 글이었습니다. 감사합니다.