package package10;
public class Tire {
public int maxRotation;
public int accumulatedRotation;
public String location;
public Tire(int maxRotation, String location) {
this.maxRotation = maxRotation;
this.location = location;
}
public boolean roll() {
accumulatedRotation++;
if(accumulatedRotation < maxRotation) {
System.out.println(location + " 타이어 수명이 " + (maxRotation - accumulatedRotation) + "만큼 남았습니다.");
return true; // 함수를 강제 종료시킴
} else {
System.out.println(location + " 타이어를 교체하세요.");
return false;
}
}
}
package package10;
public class Car {
Tire frontLeftTire = new Tire(6, "앞왼쪽"); // 1
Tire frontRightTire = new Tire(2, "앞오른쪽"); // 2
Tire backLeftTire = new Tire(3, "뒤왼쪽"); // 3
Tire backRightTire = new Tire(4, "뒤오른쪽"); // 4
public int run() {
System.out.println("차가 달립니다.");
if(frontLeftTire.roll() == false) {
stop();
return 1;
}
if(frontRightTire.roll() == false) {
stop();
return 2;
}
if(backLeftTire.roll() == false) {
stop();
return 3;
}
if(backRightTire.roll() == false) {
stop();
return 4;
}
return 0;
}
void stop() {
System.out.println("멈추세요.");
}
}
package package10;
public class CarEx {
public static void main(String[] args) {
// TODO Auto-generated method stub
int number = 5;
Car car = new Car();
boolean stop = false;
while(!stop) { // 무한 반복
int num = car.run(); // 타이어의 위치
switch(num) {
case 1:
System.out.println("앞 왼쪽 타이어를 교체");
car.frontLeftTire = new KumhoTire(15, "앞왼쪽");
// 객체 다향성
break;
case 2:
System.out.println("앞 오른쪽 타이어를 교체");
car.frontRightTire = new HankookTire(15, "앞오른쪽");
break;
case 3:
System.out.println("뒤 왼쪽 타이어를 교체");
car.backLeftTire = new HankookTire(15, "뒤왼쪽");
break;
case 4:
System.out.println("뒤 오른쪽 타이어를 교체");
car.backRightTire = new KumhoTire(15, "뒤오른쪽");
break;
}
number--;
if(number == 0) {
stop = true;
}
}
}
}
package package10;
public class KumhoTire extends Tire {
public KumhoTire(int maxRotation, String location) {
super(maxRotation, location);
}
@Override
public boolean roll() {
accumulatedRotation++;
if(accumulatedRotation < maxRotation) {
System.out.println(location + " 금호 타이어 수명이 " + (maxRotation - accumulatedRotation) + "만큼 남았습니다.");
return true; // 함수를 강제 종료시킴
} else {
System.out.println(location + " 금호 타이어를 교체하세요.");
return false;
}
}
}
package package10;
public class HankookTire extends Tire {
public HankookTire(int maxRotation, String location) {
super(maxRotation, location);
}
@Override
public boolean roll() {
accumulatedRotation++;
if(accumulatedRotation < maxRotation) {
System.out.println(location + " 한국 타이어 수명이 " + (maxRotation - accumulatedRotation) + "만큼 남았습니다.");
return true; // 함수를 강제 종료시킴
} else {
System.out.println(location + " 한국 타이어를 교체하세요.");
return false;
}
}
}
- 실행결과
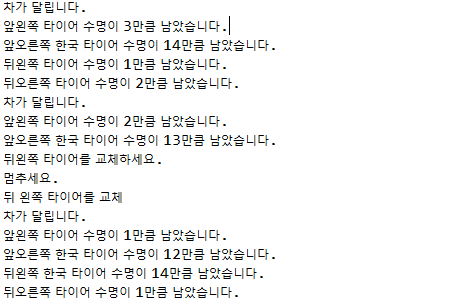