문제점
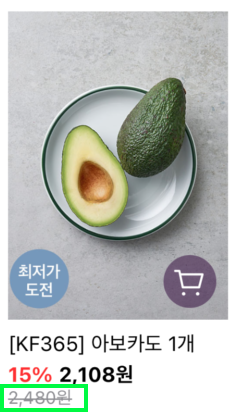
- UILabel에 취소선을 만들어주고 싶다
- 취소선뿐만 아니라 다른 속성도 알아보자
설명
NSMutableAttributedString
을 사용하면 된다
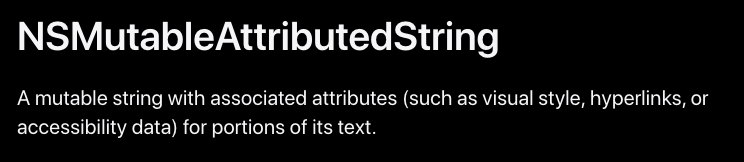
- 이것은 문자 일부에 속성에 따라, 변경 가능한 문자열이다
- 먼저 변경 가능한 문자열을 만들고
- 이 문자열에 속성(어디에 뭘할건지)을 추가한다
- 마지막으로
attributedText
에 이 문자열을 할당하면 된다
- 매번 적기 귀찮으니
UILabel
의 extension
으로 만들어두고 사용하자
- 그렇다면 무슨 종류가 있는지 보자
예시
1. 취소선
extension UILabel {
func strikethrough(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.strikethroughStyle: NSUnderlineStyle.single.rawValue], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
2. 취소선(부분적용)
- 위에 취소선에 파라미터인
at range
부분에 변경하고 싶은 부분만 넣으면 된다
3. 취소선(색상변경)
extension UILabel {
func strikethroughAndChangeLineColor(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([
NSAttributedString.Key.strikethroughStyle: NSUnderlineStyle.single.rawValue,
NSAttributedString.Key.strikethroughColor: UIColor.red
], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
4. 밑줄
extension UILabel {
func underLine(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.underlineStyle: NSUnderlineStyle.single.rawValue], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
5. 텍스트 색상
extension UILabel {
func changeTextColor(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.foregroundColor : UIColor.red], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
6. 텍스트 폰트
extension UILabel {
func changeFont(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
guard let font = UIFont(name: "Bradley Hand", size: 60.0) else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.font : font], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
결과
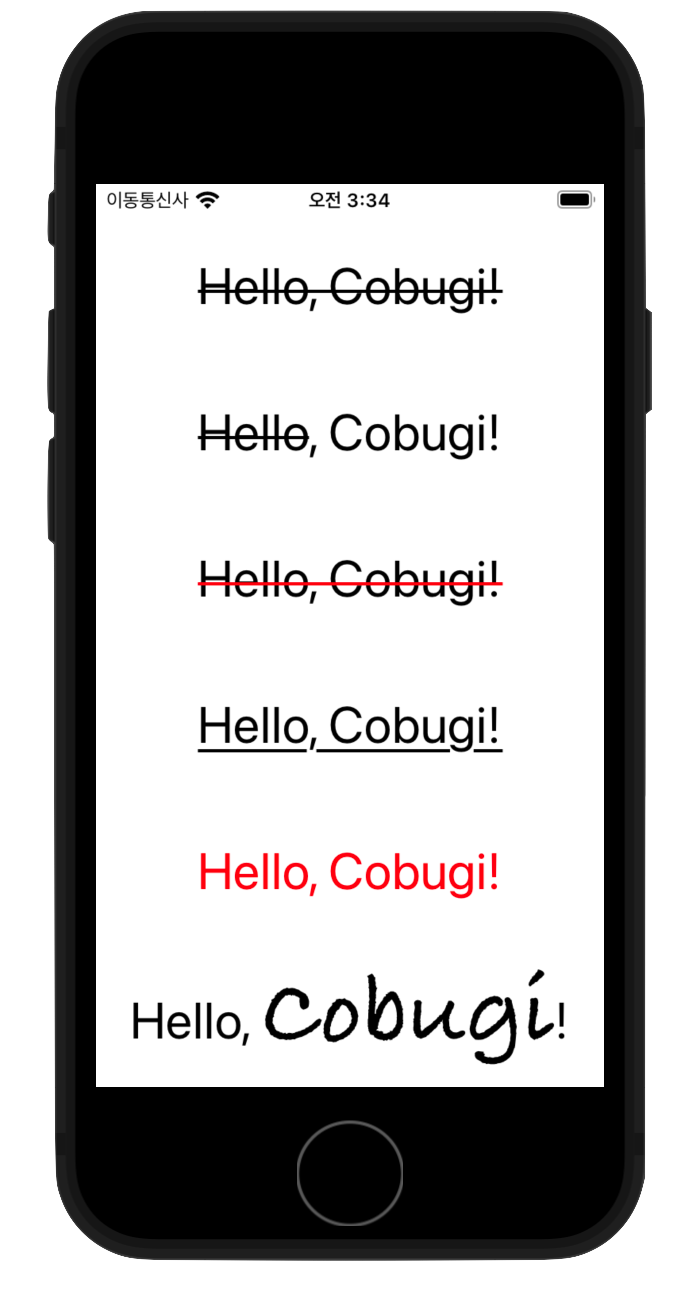
출처
전체 코드
import UIKit
import SnapKit
extension UILabel {
func strikethrough(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.strikethroughStyle: NSUnderlineStyle.single.rawValue], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
func strikethroughAndChangeLineColor(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([
NSAttributedString.Key.strikethroughStyle: NSUnderlineStyle.single.rawValue,
NSAttributedString.Key.strikethroughColor: UIColor.red
], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
func underLine(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.underlineStyle: NSUnderlineStyle.single.rawValue], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
func changeTextColor(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.foregroundColor : UIColor.red], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
func changeFont(from text: String?, at range: String?) {
guard let text = text,
let range = range else { return }
guard let font = UIFont(name: "Bradley Hand", size: 60.0) else { return }
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttributes([NSAttributedString.Key.font : font], range: NSString(string: text).range(of: range))
self.attributedText = attributedString
}
}
class StrikethroughViewController: UIViewController {
private lazy var Label1: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var Label2: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var Label3: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var Label4: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var Label5: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var Label6: UILabel = {
let label = UILabel()
label.textAlignment = .center
label.font = .systemFont(ofSize: 32.0, weight: .regular)
return label
}()
private lazy var stackView: UIStackView = {
let stackView = UIStackView()
stackView.axis = .vertical
stackView.distribution = .fillEqually
stackView.spacing = 4.0
return stackView
}()
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .systemBackground
setupLayout()
let defaultText: String = "Hello, Cobugi!"
Label1.strikethrough(from: defaultText, at: defaultText)
Label2.strikethrough(from: defaultText, at: "Hello")
Label3.strikethroughAndChangeLineColor(from: defaultText, at: defaultText)
Label4.underLine(from: defaultText, at: defaultText)
Label5.changeTextColor(from: defaultText, at: defaultText)
Label6.changeFont(from: defaultText, at: "Cobugi")
}
}
private extension StrikethroughViewController {
func setupLayout() {
view.addSubview(stackView)
[
Label1, Label2, Label3,
Label4, Label5, Label6
].forEach { stackView.addArrangedSubview($0) }
stackView.snp.makeConstraints {
$0.edges.equalTo(view.safeAreaLayoutGuide)
}
}
}