📖 문제
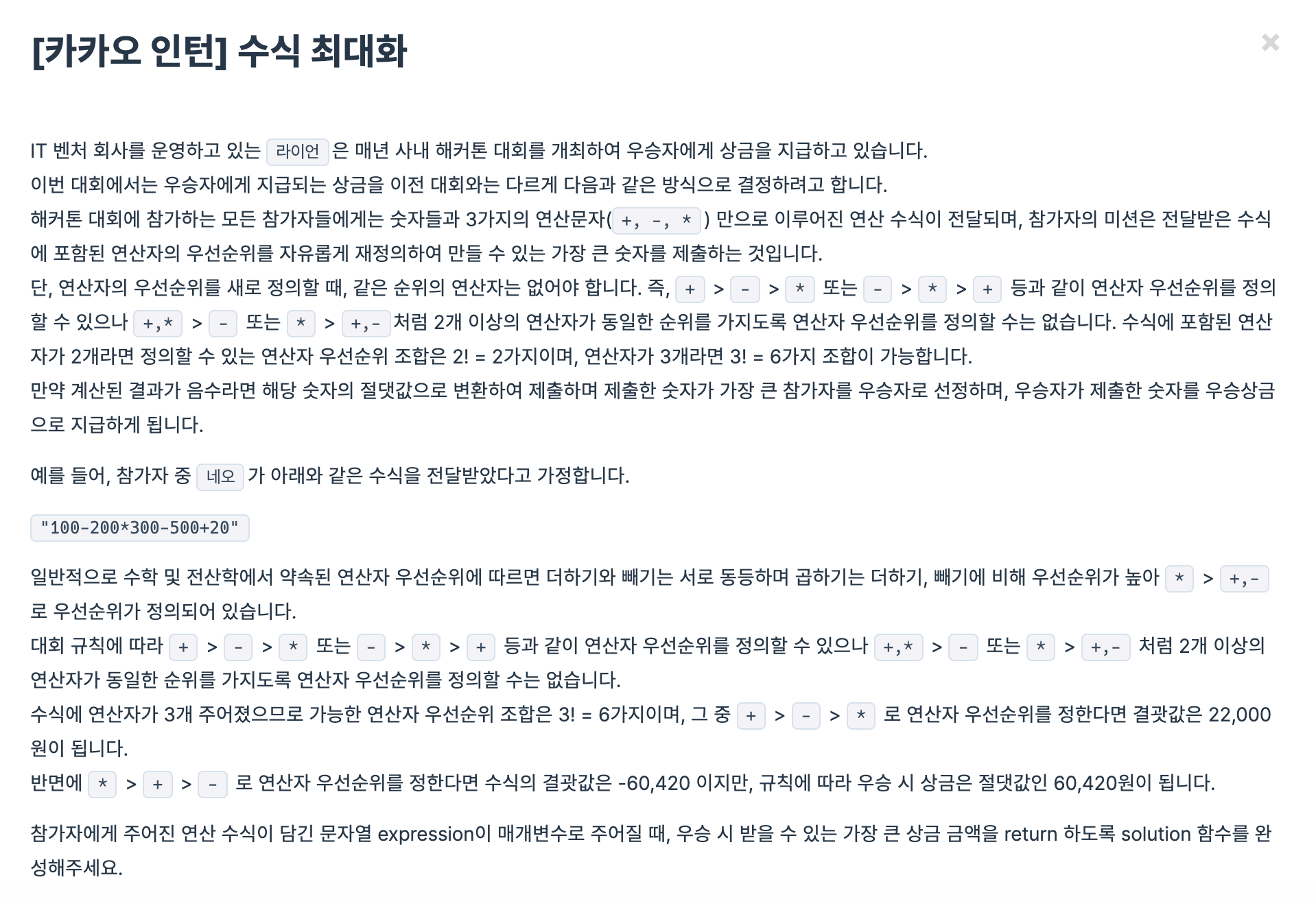
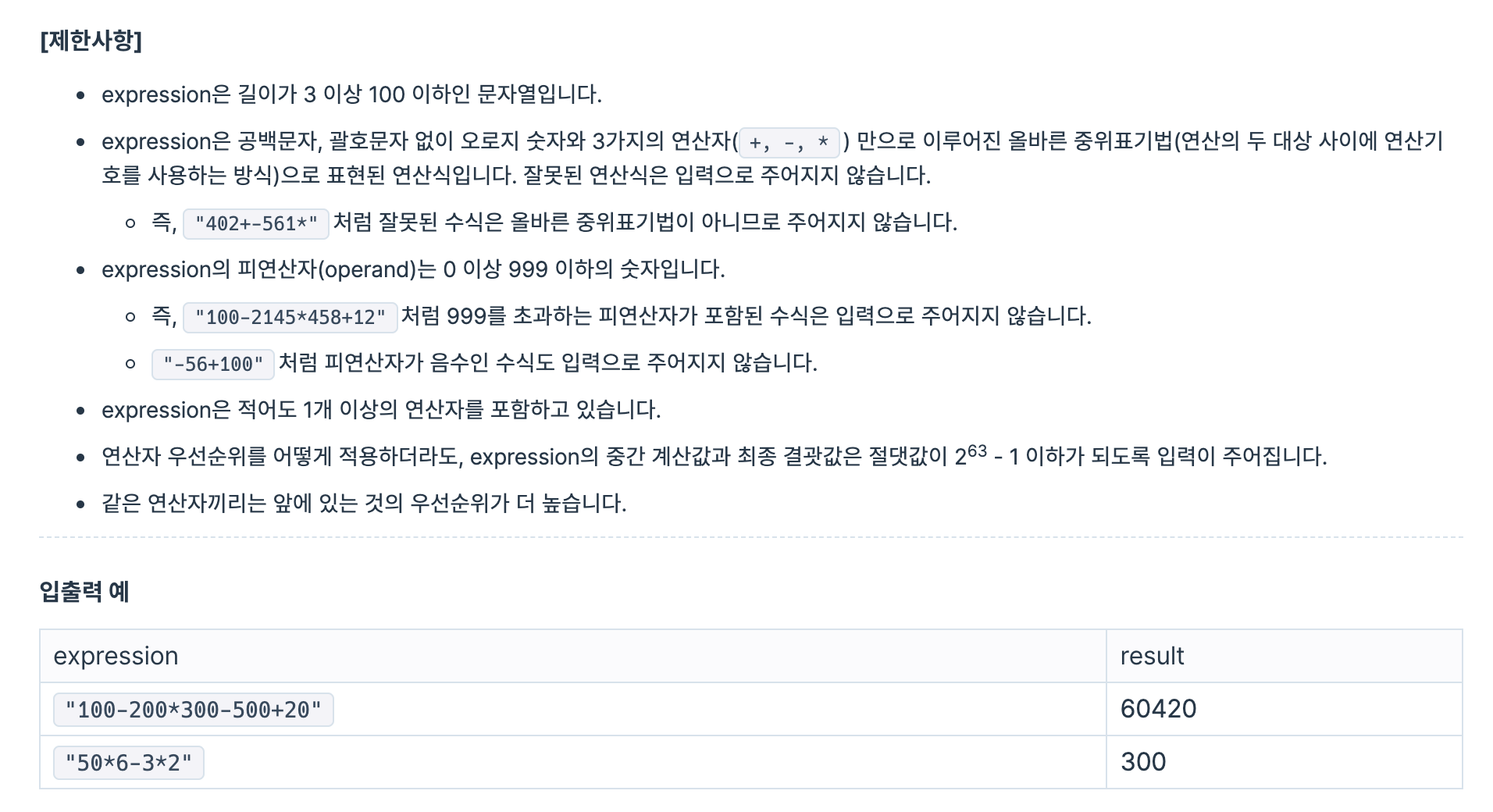
📝 풀이 과정
- 주어진 연산식
expression
에서 연산자와 피연산자들을 각각 다른 리스트에 담는다.
- python의
permutations
모듈을 사용하여 연산자 *, +, -
의 순열을 모두 구한다.
-> 우선순위를 결정(좌측에 있을수록 우선순위가 높다)
- 2번에서 구한 모든 우선순위에 대해 연산식
expression
을 계산하는 함수calculation
을 호출한다. 그리고 그 리턴값을 리스트 result
에 저장
- 리스트
result
에 있는 요소들 중 최댓값이 정답
⌨ 코드
from itertools import permutations as p
import re
def calculation(operators, operands, priority):
priority = list(priority)
operat = operators[:]
operan = operands[:]
for cur_op in priority:
while True:
if cur_op in operat:
op_idx = operat.index(cur_op)
else:
break
if cur_op == '*':
operan[op_idx] = operan[op_idx] * operan[op_idx+1]
elif cur_op == '+':
operan[op_idx] = operan[op_idx] + operan[op_idx+1]
elif cur_op == '-':
operan[op_idx] = operan[op_idx] - operan[op_idx+1]
operan.pop(op_idx+1)
operat.pop(op_idx)
return operan[0] if operan[0] > 0 else -operan[0]
def solution(expression):
result = []
operand = ['+', '-', '*']
operands = list(map(int,re.split('[*+-]', expression)))
operators = list(re.split('[0-9]+', expression))[1:-1]
for priority in p(operand,3):
result.append(calculation(operators, operands, priority))
return max(result)