용어
- vortex = node
- edge = connection (mostly bi-directional)
- vortex의 plural형 = vertices
함수 구현
import java.util.ArrayList;
import java.util.HashMap;
public class Graphs {
// adjList
private HashMap<String, ArrayList<String>> adjList = new HashMap<>();
public void printGraph() {
System.out.println(adjList);
}
public boolean addVertex(String vertex) {
// hashmap : we can't have duplicates
// so we should have some code to make sure the value we're using for our vertex
// isn't already in our hashmap.
if (adjList.get(vertex) == null) {
adjList.put(vertex, new ArrayList<String>());
return true;
}
return false;
}
public boolean addEdge(String vertex1, String vertex2) {
if(adjList.get(vertex1)!=null && adjList.get(vertex2)!=null) {
adjList.get(vertex1).add(vertex2);
adjList.get(vertex2).add(vertex1);
return true;
}
return false;
}
public boolean removeEdge(String vertex1, String vertex2) {
if(adjList.get(vertex1)!=null && adjList.get(vertex2)!=null) {
adjList.get(vertex1).remove(vertex2);
adjList.get(vertex2).remove(vertex1);
return true;
}
return false;
}
public boolean removeVertex(String vertex) {
if(adjList.get(vertex) == null) return false;
// 해당 vertex가 그래프 안에 없음
for(String otherVertex : adjList.get(vertex)) {
adjList.get(otherVertex).remove(vertex);
}
adjList.remove(vertex);
return true;
}
}
Main 실행 부분
public class Main {
public static void main(String[] args) {
Graphs myGraph = new Graphs();
myGraph.addVertex("A");
myGraph.addVertex("B");
myGraph.addVertex("C");
myGraph.addVertex("D");
myGraph.addEdge("A", "B");
myGraph.addEdge("A", "C");
myGraph.addEdge("B", "D");
myGraph.addEdge("D", "C");
myGraph.addEdge("A", "D");
myGraph.printGraph();
myGraph.removeEdge("A", "B");
myGraph.printGraph();
myGraph.removeEdge("C", "B");
myGraph.printGraph();
myGraph.removeVertex("D");
myGraph.printGraph();
}
}
결과 : 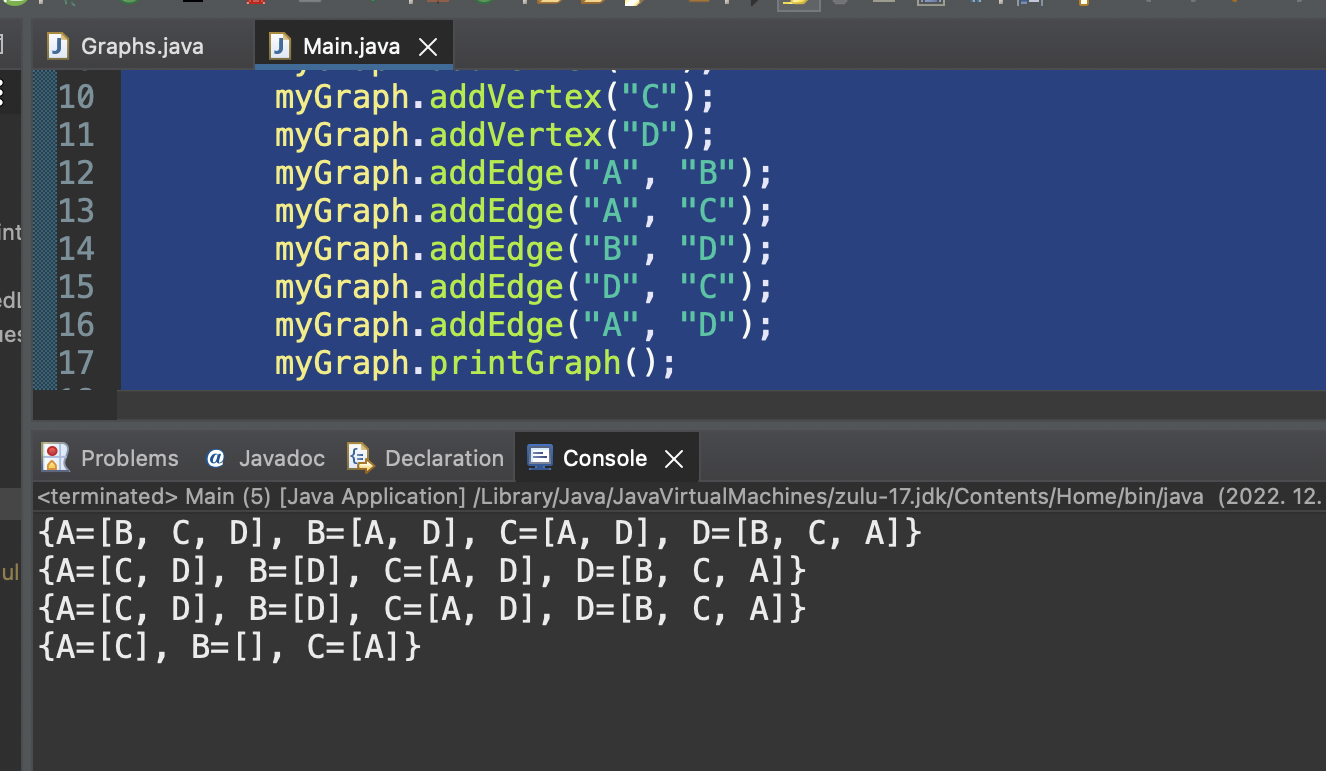