파일업로드
YAML
#server port
server:
port: 9091
servlet:
context-path: /
encoding:
charset: utf-8
enabled: true
error:
path: /error
#spring setting
spring:
output:
ansi:
enabled: always
datasource:
driver-class-name: oracle.jdbc.driver.OracleDriver
url: jdbc:oracle:thin:@localhost:1521:xe
username: "------"
password: "------"
thymeleaf:
cache: false
servlet:
multipart:
max-file-size: 10MB
max-request-size: 100MB
# autoconfigure:
# exclude: org.springframework.boot.autoconfigure.web.ErrorMvcAutoConfiguration
logging:
level:
root: INFO
'[com.jjang051.gallery]': DEBUG
mybatis:
mapper-locations: classpath:mapper/sql/**/*.xml
config-location: classpath:mapper/config/config.xml
file:
path: C:/gallery/upload/
WebConfig
package com.jjang051.gallery.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Value("${file.path}")
private String uploadPath;
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry
.addResourceHandler("/upload/**")
.addResourceLocations("file:///" + uploadPath);
}
}
ServiceImpl
@Service
public class GalleryServiceImpl implements GalleryService {
@Autowired
GalleryDao galleryDao;
@Value("${file.path}")
private String uploadFoler;
@Override
public int insertGallery(GalleryDto galleryDto) {
UUID uuid = UUID.randomUUID();
// form에서 전달된 파일이름
String originalFile = galleryDto.getFile().getOriginalFilename();
// 중복방지용 이름
String renamedFile = uuid + "_" + originalFile;
Path imgFilePath = Paths.get(uploadFoler + renamedFile);
galleryDto.setOriginal(originalFile);
galleryDto.setRenamed(renamedFile);
try {
Files.write(imgFilePath, galleryDto.getFile().getBytes());
} catch (IOException e) {
e.printStackTrace();
}
int result = galleryDao.insertGallery(galleryDto);
return result;
}
@Override
public List<GalleryDto> getAllList() {
List<GalleryDto> galleryList = galleryDao.getAllList();
return galleryList;
}
@Override
public GalleryDto viewGallery(int no) {
GalleryDto galleryDto = galleryDao.viewGallery(no);
return galleryDto;
}
@Override
public int insertReply(ReplyDto replyDto) {
int result = galleryDao.insertReply(replyDto);
return result;
}
@Override
public int deleteReply(ReplyDto replyDto) {
int result = galleryDao.deleteReply(replyDto);
return result;
}
@Override
public List<ReplyDto> getAllReply(int galleryId) {
List<ReplyDto> replyList = galleryDao.getAllReply(galleryId);
return replyList;
}
}
댓글
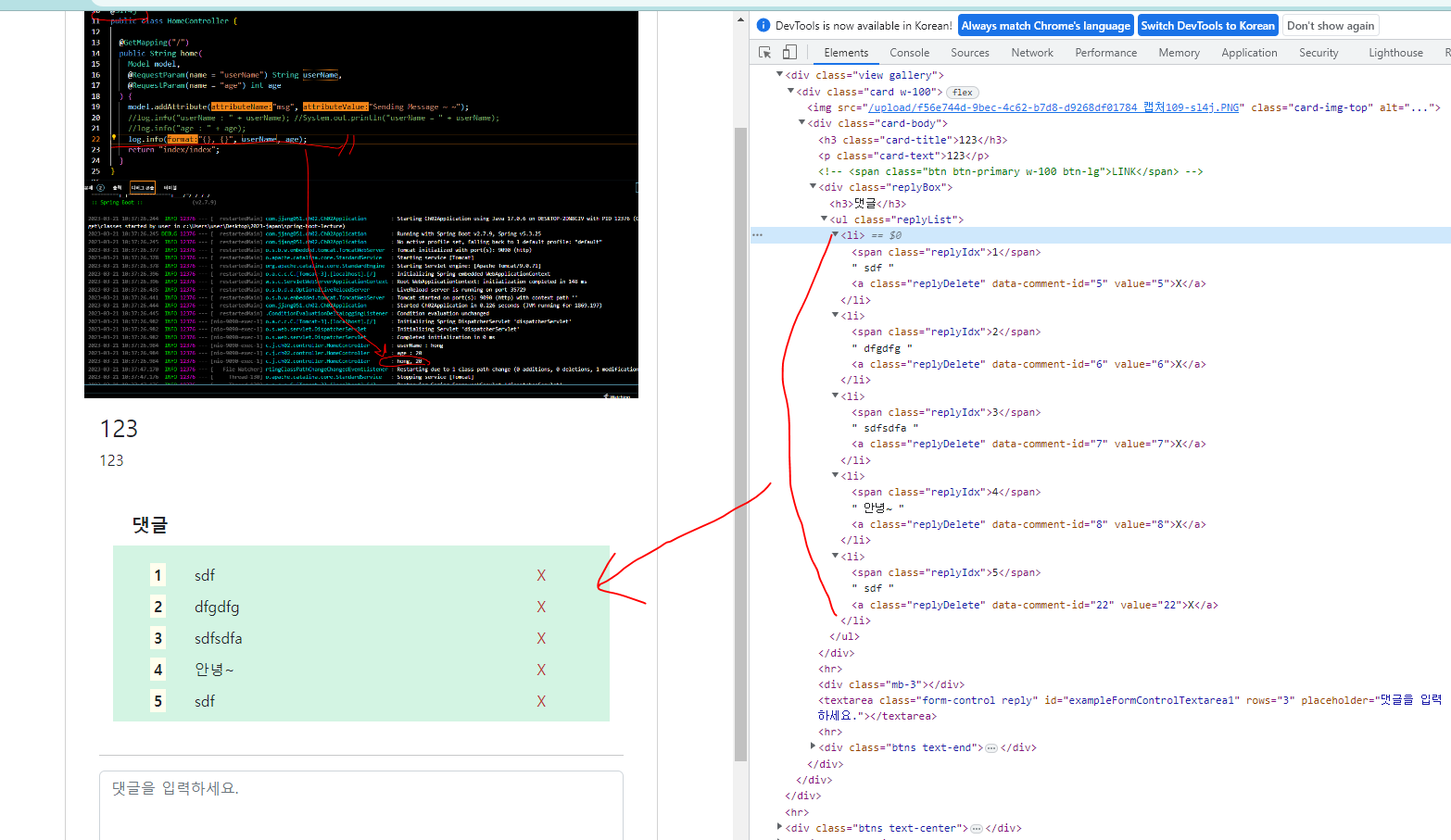
- 댓글로 입력하는 건 ajax로 구현하기 쉬웠는데 삭제는 어려웠다.
- 왜냐하면 같은 클래스명을 가진 삭제버튼은 여러개인데 (댓글 뒤에 X모양) 그것들이 각각 가져야 하는 value 값 (댓글의 pk)이 서로 다르기 때문
- 그러다가 data- ~~ 형식으로 클래스명 대신에 무언가 속성을 부여하는 방법을 알게 됨
삭제 부분 AJAX
$(document).on("click", ".replyDelete", function() {
const commentNo = $(this).data('comment-id');
console.log(commentNo);
const sendData = {
galleryId:galleryId,
no:commentNo
}
$.ajax({
url:"/deleteReply",
data:sendData,
type:"POST",
success:function(response) {
//console.log(response);
let tempHtml="";
$.each(response,function(idx,item){
tempHtml+=`<li> <span class="replyIdx">${idx+1}</span> ${item.comments}
<a class="replyDelete" data-comment-id="${item.no}" value="${item.no}">X</a></li>`
});
$(".replyBox ul").html(tempHtml);
}
})
});
전체 AJAX (참고)
HTML
<div class="replyBox">
<h3>댓글</h3>
<ul class="replyList">
</ul>
</div>
<hr>
<div class="mb-3"></div>
<textarea class="form-control reply" id="exampleFormControlTextarea1" rows="3"
placeholder="댓글을 입력하세요."></textarea>
<hr>
<div class="btns text-end">
<button class="btn btn-primary" id="btnConfirm">CONFIRM</button>
</div>
</div>
AJAX
<script th:inline="javascript">
const galleryId = [[${galleryDto.no}]];
console.log("gallery ID : " + galleryId);
function loadReply() {
const sendData = {
galleryId:galleryId,
}
$.ajax({
url:"/getReply/" + galleryId,
data:sendData,
type:"POST",
success:function(response) {
console.log(response);
let tempHtml="";
$.each(response,function(idx,item){
tempHtml+=`<li> <span class="replyIdx">${idx+1}</span> ${item.comments}
<a class="replyDelete" data-comment-id="${item.no}" value="${item.no}">X</a></li>`
});
$(".replyBox ul").html(tempHtml);
}
})
}
loadReply();
$("#btnConfirm").on("click",function(){
const sendData = {
galleryId:galleryId,
comments:$(".reply").val()
}
$.ajax({
url:"/insertReply",
data:sendData,
type:"POST",
success:function(response) {
let tempHtml="";
$.each(response,function(idx,item){
tempHtml+=`<li> <span class="replyIdx">${idx+1}</span> ${item.comments}
<a class="replyDelete" data-comment-id="${item.no}" value="${item.no}">X</a></li>`
});
$(".replyBox ul").html(tempHtml);
$("#exampleFormControlTextarea1").val('');
}
})
});
$(document).on("click", ".replyDelete", function() {
const commentNo = $(this).data('comment-id');
console.log(commentNo);
const sendData = {
galleryId:galleryId,
no:commentNo
}
$.ajax({
url:"/deleteReply",
data:sendData,
type:"POST",
success:function(response) {
let tempHtml="";
$.each(response,function(idx,item){
tempHtml+=`<li> <span class="replyIdx">${idx+1}</span> ${item.comments}
<a class="replyDelete" data-comment-id="${item.no}" value="${item.no}">X</a></li>`
});
$(".replyBox ul").html(tempHtml);
}
})
});
</script>
Controller
@GetMapping("/view/{no}")
public String view(@PathVariable("no") int no, Model model) {
GalleryDto galleryDto = galleryService.viewGallery(no);
model.addAttribute("galleryDto", galleryDto);
return "/gallery/view";
}
@PostMapping("/getReply/{no}")
@ResponseBody
public List<ReplyDto> getReply(@PathVariable("no") int no) {
List<ReplyDto> replyList = galleryService.getAllReply(no);
return replyList;
}
@PostMapping("/insertReply")
@ResponseBody
public List<ReplyDto> insertReply(ReplyDto replyDto) {
int result = galleryService.insertReply(replyDto);
List<ReplyDto> replyList = galleryService.getAllReply(
replyDto.getGalleryId()
);
if (result > 0) {
return replyList;
} else {
return replyList;
}
}
@PostMapping("/deleteReply")
@ResponseBody
public List<ReplyDto> deleteReply(ReplyDto replyDto) {
int result = galleryService.deleteReply(replyDto);
List<ReplyDto> replyList = galleryService.getAllReply(
replyDto.getGalleryId()
);
if (result > 0) {
return replyList;
} else {
return replyList;
}
}