서브쿼리
select 컬럼
from 테이블
where 조건 연산자 (select 컬럼
from 테이블
where 조건);
- 메인쿼리 내부에 정의된 쿼리
- 서브쿼리가 먼저 실행되고 이후 메인쿼리가 실행됨
- 작성 위치에 따른 기능
select 단순값
from 가상테이블
where 조건식
사용 예
employee_id 100번과 같은 부서에서 일하는 사람의 정보 조회
-- 100번 사원이 일하는 부서 조회
select first_name, department_id
from employees
where employee_id = '100';
-- 100번 사원의 부서번호는 10으로 가정
-- 그 부서 소속 사람 조회
select employee_id, first_name
from employees
where department_id = '10';
select employee_id, first_name
from employees
where department_id = (select department_id
from employees
where employee_id = '100');
서브쿼리의 종류
단일행 서브쿼리
- 서브쿼리 수행 시 하나의 행만 반환

다중행 서브쿼리
SELECT column, column, ...
FROM table1
WHERE column 연산자 (select column
from table2);
- 서브쿼리 수행 시 여러 행 반환
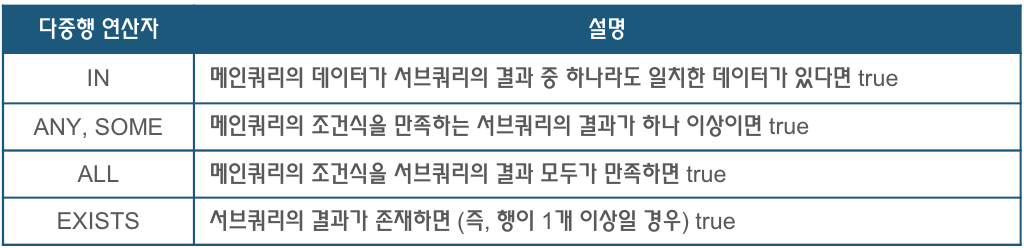
다중컬럼 서브쿼리
select column, column, ....
from table1
where (columnA, columnB) 관계연산자 (select columnA, columnB
from table2)
having절 서브쿼리
select column
from table1
group by column
having 그룹함수 연산자 (select column
from table)
상호연관 서브쿼리
select outer.column1, outer.column2, ...
from table1 outer
where column 연산자 (select column1
from table2
where column = outer.column3);
- 서브쿼리가 메인쿼리를 참조하는 것
- 바깥 쿼리로 실행되는 모든 행을 조회함
- 성능이 나쁘므로 사용시 유의
select first_name, position, salary
from employee e1
where salary >= (select avg(salary)
from employee e2
where e1.employee_id = e2.employee_id)
with절
with
이름1 as ( select 반복구문 ),
이름2 as ( select 반복구문2)
select 컬럼
from 테이블;
- 원하는 테이블(보통 반복되는 구문)을 가상테이블로 저장
- 단독으로 사용 불가하며 수행할 sql문이 함께 와야함
- 중첩하여 사용 가능
WITH
TEST AS (SELECT a.employee_id, b.first_name
FROM a,b),
TEST2 AS (SELECT a.manager_id, c.salary
FROM a,c)
SELECT *
FROM TEST, TEST2
WHERE TEST.employee_id = TEST2.manager_id;