01. 순서도로 복잡한 문제를 단순하게 만들기
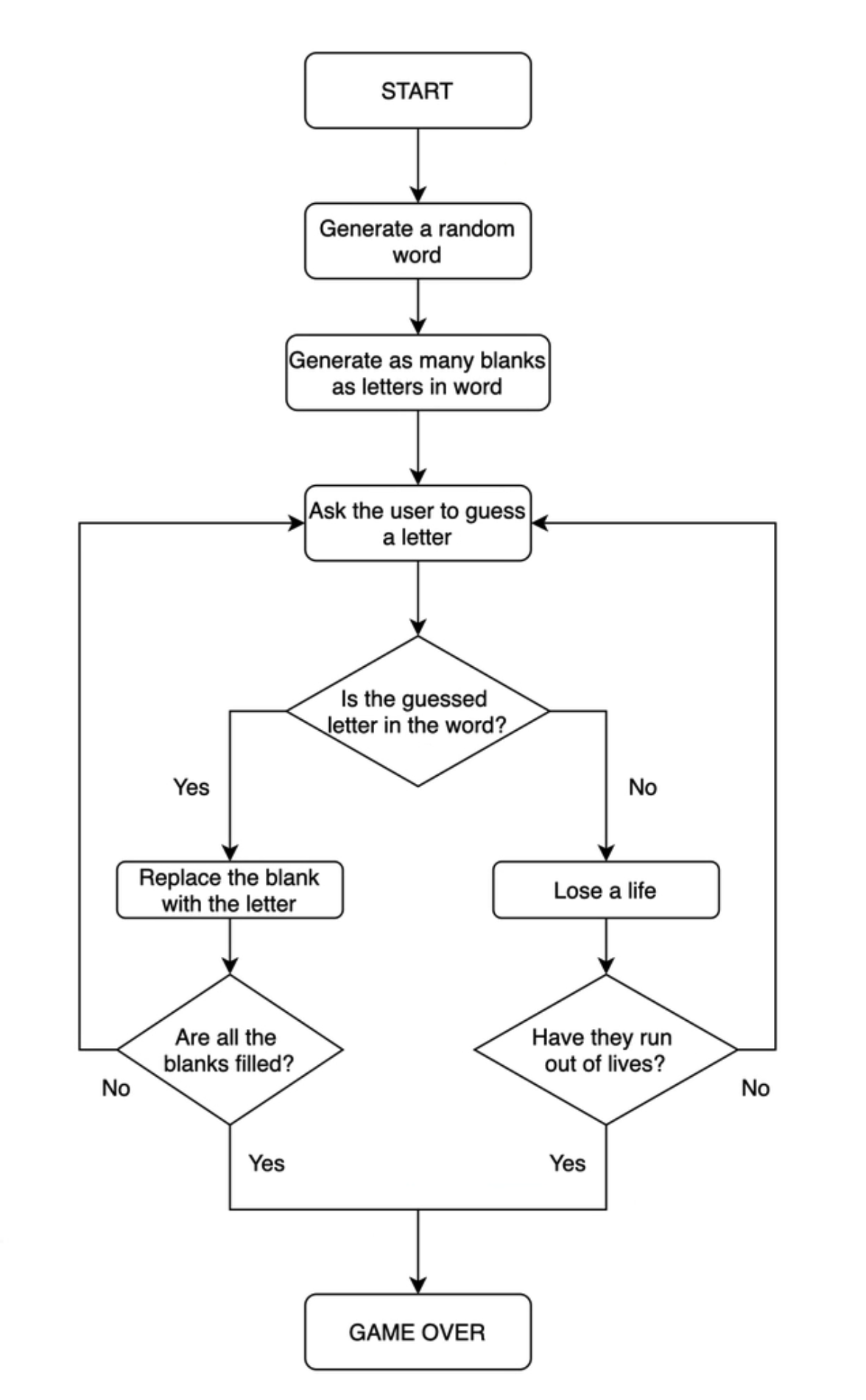
02. 무작위 단어를 고르고 정답 확인하기
import random
chosen_word = random.choice(word_list)
guess = input("Guess a letter : ").lower()
for letter in chosen_word:
if letter == guess:
print("True")
else:
print("False")
03. 빈칸을 맞춘 글자로 바꾸기
import random
word_list = ["aardvark", "baboon", "camel"]
chosen_word = random.choice(word_list)
print(f'Pssst, the solution is {chosen_word}.')
display = []
word_length = len(chosen_word)
for _ in range(word_length):
display.append("_")
guess = input("Guess a letter: ").lower()
for position in range(word_length):
letter = chosen_word[position]
if letter == guess :
display[position] +=letter
print(display)
04. 플레이어가 이겼는지 확인하기
import random
word_list = ["aardvark", "baboon", "camel"]
chosen_word = random.choice(word_list)
word_length = len(chosen_word)
print(f'Pssst, the solution is {chosen_word}.')
display = []
for _ in range(word_length):
display += "_"
end_of_game = False
while not end_of_game:
guess = input("Guess a letter: ").lower()
for position in range(word_length):
letter = chosen_word[position]
print(f"Current position: {position}\n Current letter: {letter}\n Guessed letter: {guess}")
if letter == guess:
display[position] = letter
print(display)
if "_" not in display:
end_of_game = True
print("You win.")
05. 플레이어의 남은 목숨 세기
import random
stages = ['''
+---+
| |
O |
/|\ |
/ \ |
|
=========
''', '''
+---+
| |
O |
/|\ |
/ |
|
=========
''', '''
+---+
| |
O |
/|\ |
|
|
=========
''', '''
+---+
| |
O |
/| |
|
|
=========''', '''
+---+
| |
O |
| |
|
|
=========
''', '''
+---+
| |
O |
|
|
|
=========
''', '''
+---+
| |
|
|
|
|
=========
''']
end_of_game = False
word_list = ["ardvark", "baboon", "camel"]
chosen_word = random.choice(word_list)
word_length = len(chosen_word)
lives = 6
print(f'Pssst, the solution is {chosen_word}.')
display = []
for _ in range(word_length):
display += "_"
while not end_of_game:
guess = input("Guess a letter: ").lower()
for position in range(word_length):
letter = chosen_word[position]
if letter == guess:
display[position] = letter
if guess not in chosen_word:
lives -= 1
if lives == 0:
end_of_game = True
print("You Lose")
print(f"{' '.join(display)}")
if "_" not in display:
end_of_game = True
print("You win.")
print(stages[lives])
06. 유저 경험 개선시키기
import random
from hangman_art import stages, logo
from hangman_words import word_list
from replit import clear
print(logo)
game_is_finished = False
lives = len(stages) - 1
chosen_word = random.choice(word_list)
word_length = len(chosen_word)
display = []
for _ in range(word_length):
display += "_"
while not game_is_finished:
guess = input("Guess a letter: ").lower()
clear()
if guess in display:
print(f"You've already guessed {guess}")
for position in range(word_length):
letter = chosen_word[position]
if letter == guess:
display[position] = letter
print(f"{' '.join(display)}")
if guess not in chosen_word:
print(f"You guessed {guess}, that's not in the word. You lose a life.")
lives -= 1
if lives == 0:
game_is_finished = True
print("You lose.")
if not "_" in display:
game_is_finished = True
print("You win.")
print(stages[lives])