1. Model 1로 로그인페이지만들기
- Model 1 아키텍처는 JSP와 JavaBeans만 사용하여 웹을 개발하는것
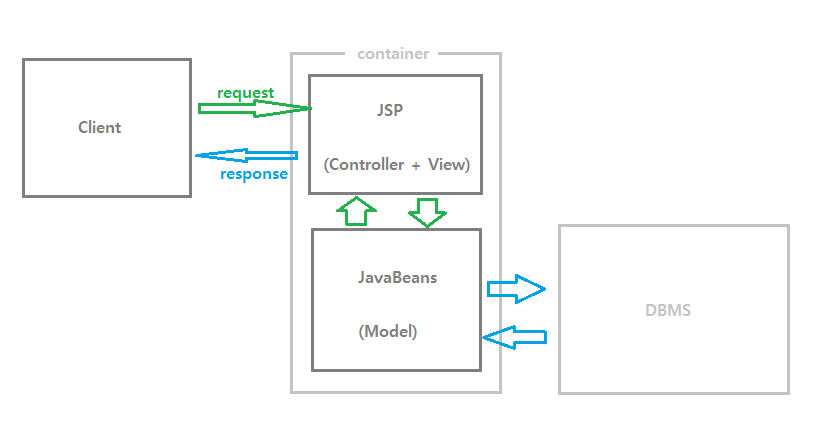
- Model 1 구조는 JSP 파일에서 Controller 기능과 View 기능을 모두 처리한다.
- JSP 파일에 자바코드와 마크업 코드들이 뒤섞여 유지보수가 어렵다.
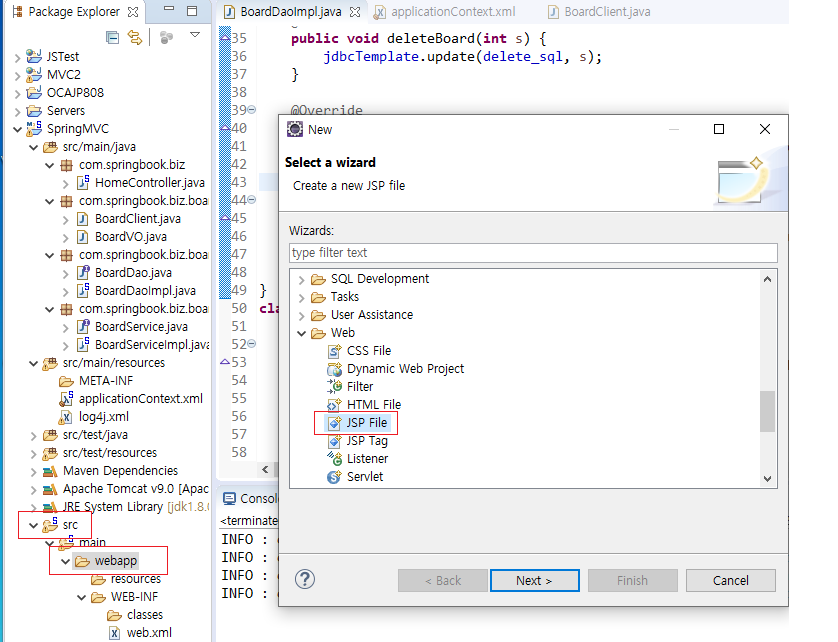
- 로그인정보를 사용자가 입력할 수 있는 틀을 JSP파일에 만들어준다.
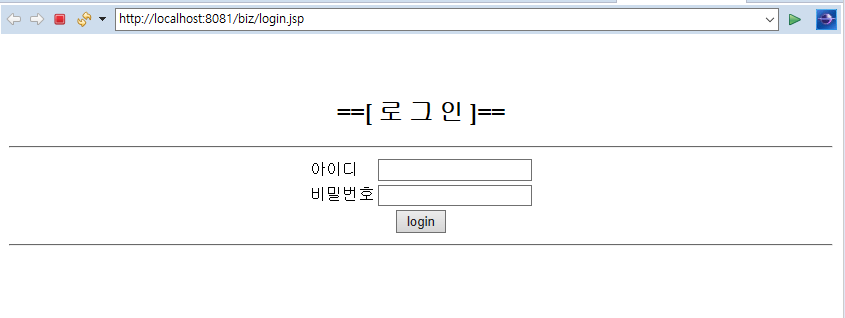
- 톰캣정보 중 경로이름을 프로젝트명과 동일하게 변경할 수 있다.
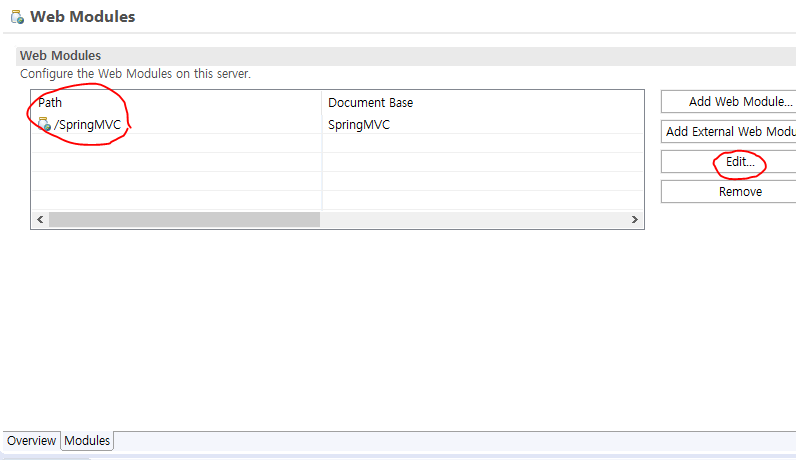
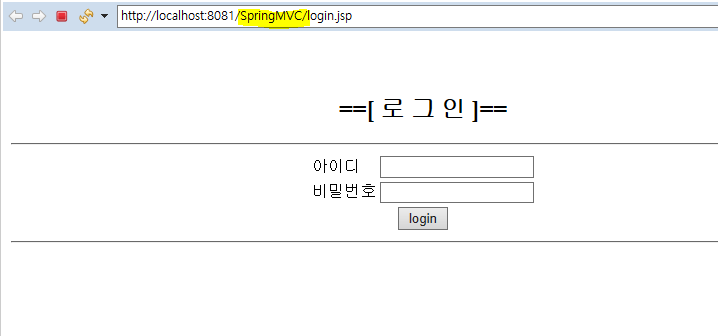
- submit버튼을 누르면 action= login_proc.jsp 로 연결되도록 설정한다.
- login_proc.jsp파일을 설정한다.
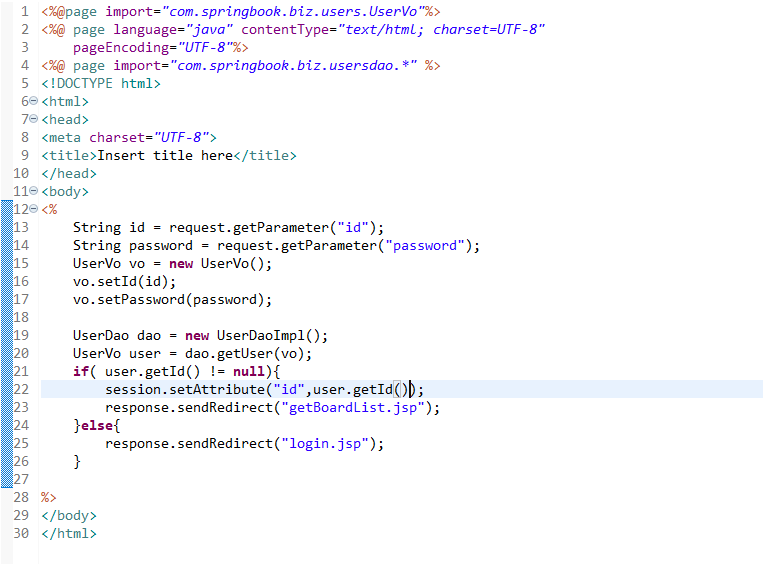
- 다만, MVC 모델1을 사용할때, login_proc.jsp 파일은 applicationContext.xml 파일을 열 수있는 코드를 작성하지 않았기 때문에 DB를 이용할 수 있는 파일을 따로 작성해 주어야한다.
package com.springbook.biz.users;
import java.sql.*;
public class JDBCUtill {
private static JDBCUtill db = new JDBCUtill() ;
private JDBCUtill(){}
public static JDBCUtill getInstance(){
return db;
}
public Connection getConnection(){
Connection conn =null;
try {
Class.forName("oracle.jdbc.OracleDriver");
conn = DriverManager.getConnection("jdbc:oracle:thin:@//localhost:1521/xe","system","1234");
} catch( Exception e) {
e.printStackTrace();
}
return conn;
}
public static void close( PreparedStatement pstmt , Connection conn) {
if(pstmt != null ) {
try {
if (!pstmt.isClosed()) pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
} finally {
pstmt =null ;
}
}
if(conn != null ) {
try {
if (!conn.isClosed()) conn.close();
} catch (SQLException e) {
e.printStackTrace();
} finally {
conn =null ;
}
}
}
public static void close(ResultSet rs ,PreparedStatement pstmt , Connection conn) {
if(rs != null ) {
try {
if (!rs.isClosed()) rs.close();
} catch (SQLException e) {
e.printStackTrace();
} finally {
rs =null ;
}
}
if(pstmt != null ) {
try {
if (!pstmt.isClosed()) pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
} finally {
pstmt =null ;
}
}
if(conn != null ) {
try {
if (!conn.isClosed()) conn.close();
} catch (SQLException e) {
e.printStackTrace();
} finally {
conn =null ;
}
}
}
}
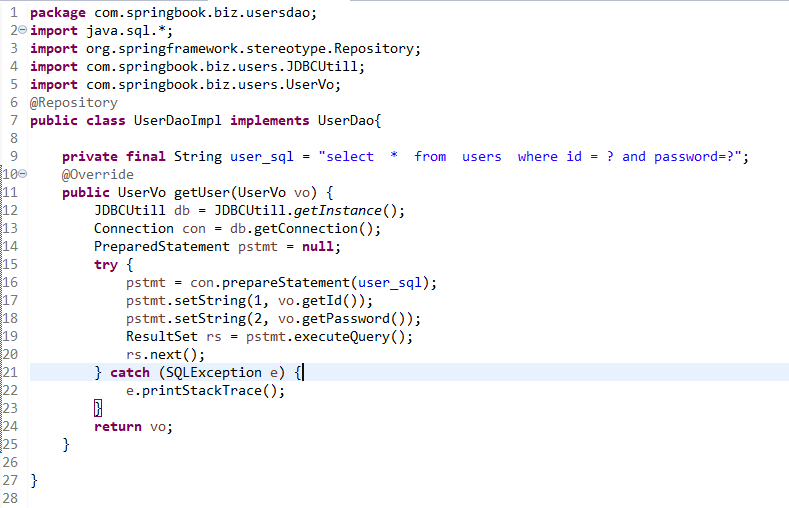
- 예전에 연습했던 패턴을 적용해서 DAO를 수정한다.
- id와 password가 일치하는 결과값이 있는경우 로그인성공으로 getUserList.jsp로 이동한다.
일치하는 결과값이 없는경우 다시 login.jsp로 이동한다.
- 결과화면 (id password 불일치시)
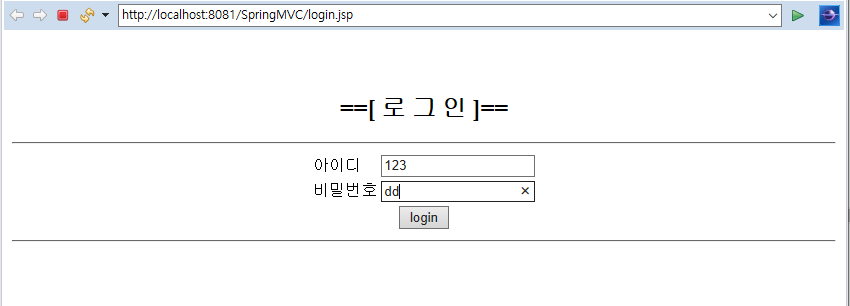
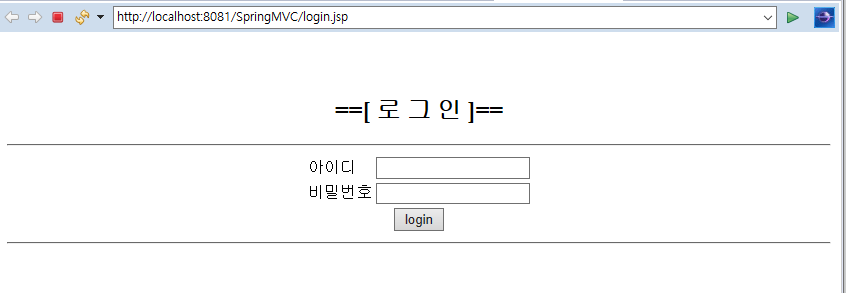
다시 로그인 창으로 돌아간다.
- 결과화면 (id password 일치시)
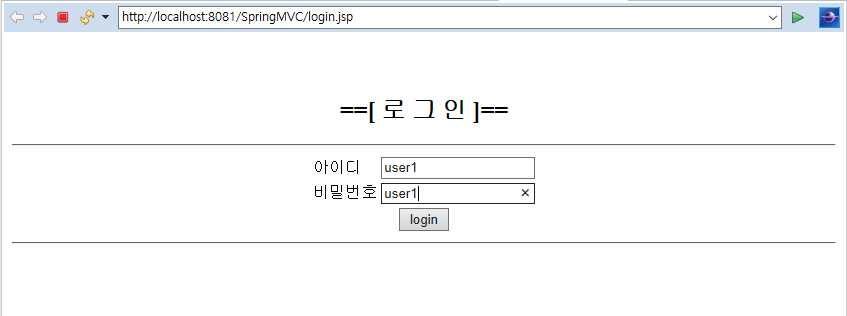
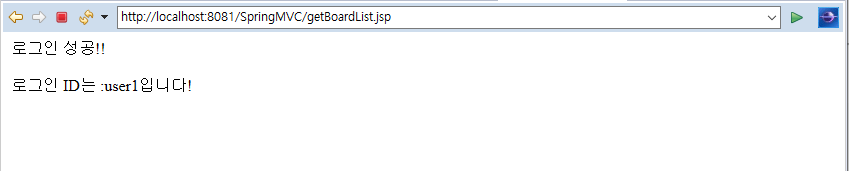
로그인 성공페이지를 확인할 수 있다.
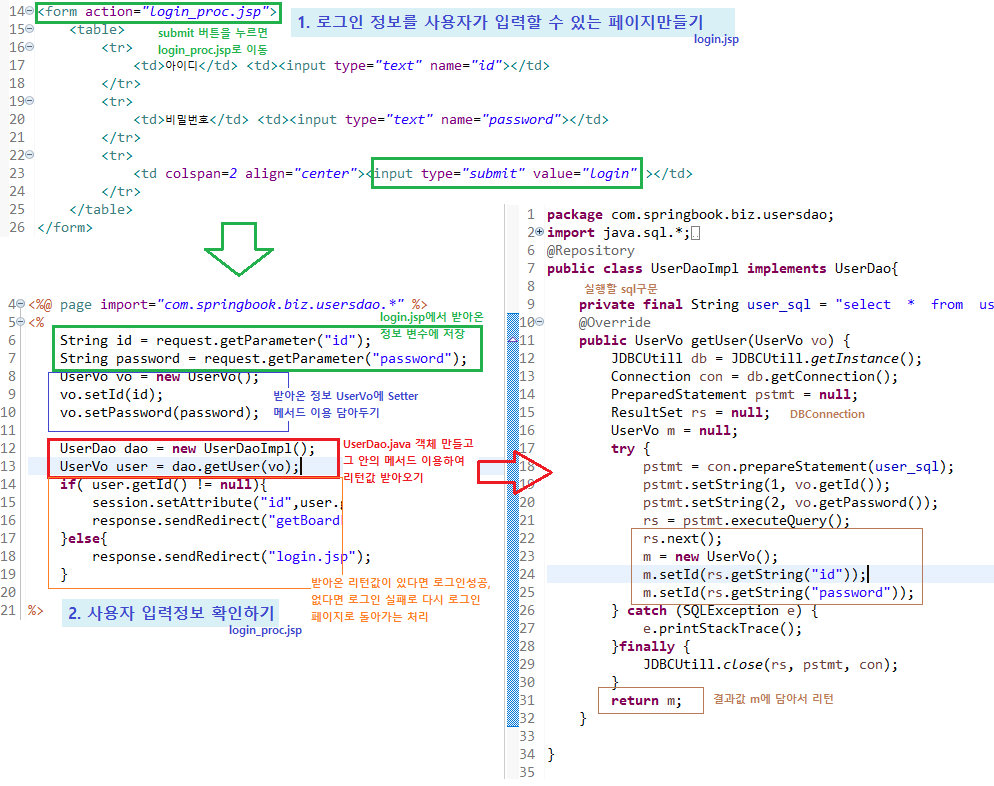
2. Model 1로 목록보기 만들기
- 목록을 출력할 모양을 getBoardList.jsp 파일로 만들어준다.
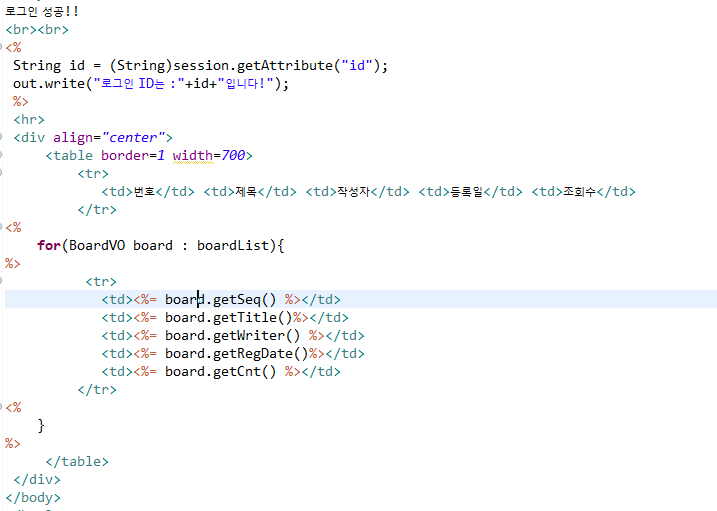
- 이때 for문으로 dao를 통해 넘겨받은 결과 List를 반복문출력한다.
- daoImpl을 작성하는데 이때도 마찬가지로 JDBCTemplate을 이용할 수 없기때문에
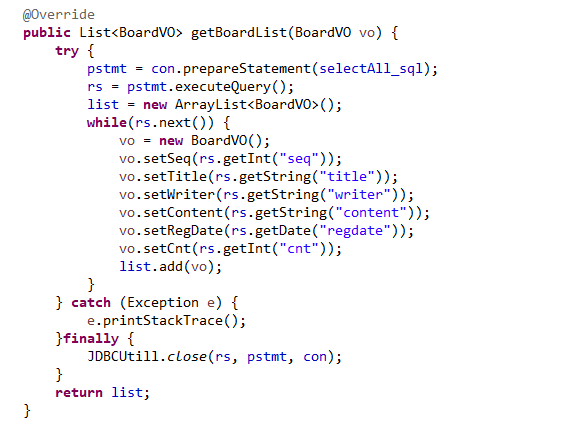
이런식으로 DB연결 - >쿼리실행 -> 값리턴
순으로 진행시켜준다.
- 로그인페이지에서 로그인을 성공하면 아래와 같은 결과화면이 출력된다.
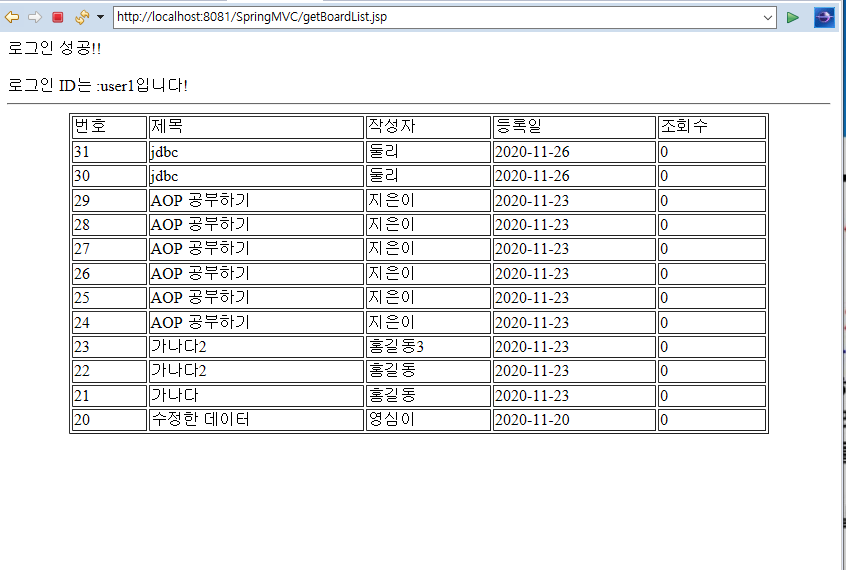
3. Model 1로 상세보기 만들기
- getBoardList에서 seq번호에 링크를 걸고 클릭하면 getBoard.jsp로 넘어가도록 설정한다.
이때, 링크에 seq도 함께 넘긴다.
<td><a href="getBoard.jsp?seq=<%= board.getSeq() %>"><%= board.getSeq() %></a></td>
- getBoardList에서 넘긴 seq값을 getBoard에서 받아 변수에 저장한다.
int seq = Integer.parseInt(request.getParameter("seq"));
- BoardDaoImpl에 getBoard 메서드를 만든다.
@Override
public BoardVO getBoard(BoardVO vo) {
try {
pstmt = con.prepareStatement(selectOne_sql);
pstmt.setInt(1, vo.getSeq());
rs = pstmt.executeQuery();
rs.next();
vo = new BoardVO();
vo.setSeq(rs.getInt("seq"));
vo.setTitle(rs.getString("title"));
vo.setWriter(rs.getString("writer"));
vo.setContent(rs.getString("content"));
vo.setRegDate(rs.getDate("regdate"));
vo.setCnt(rs.getInt("cnt"));
} catch (Exception e) {
e.printStackTrace();
}finally {
JDBCUtill.close(rs, pstmt, con);
}
return vo;
}
- dao메서드의 실행결과값을 getBoard JSP파일에서 받아 변수 값을 화면에 출력한다.
<%@page import="java.sql.Date"%>
<%@page import="com.springbook.biz.boarddao.BoardDaoImpl"%>
<%@page import="com.springbook.biz.boarddao.BoardDao"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="com.springbook.biz.board.*" %>
<%
BoardVO vo = new BoardVO();
BoardDao dao = new BoardDaoImpl();
int seq = Integer.parseInt(request.getParameter("seq"));
vo.setSeq(seq);
BoardVO board = dao.getBoard(vo);
String title = board.getTitle();
String writer = board.getWriter();
String content = board.getContent();
Date regdate = board.getRegDate();
int cnt = board.getCnt();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>상세보기</title>
</head>
<body>
<div align="center">
<br><br>
<h2>회원상세보기</h2>
<hr>
<table border=1 width=500>
<tr>
<td>번호</td> <td><input type="text" value="<%=seq %>" name="seq"></td>
</tr>
<tr>
<td>제목</td> <td><input type="text" value="<%=title %>" name="title"></td>
</tr>
<tr>
<td>작성자</td> <td><input type="text" value="<%=writer %>" name="writer"></td>
</tr>
<tr>
<td>글내용</td> <td><input type="text" value="<%=content %>" name="content"></td>
</tr>
<tr>
<td>등록일</td> <td><input type="text" value="<%=regdate %>" name="regdate"></td>
</tr>
<tr>
<td>조회수</td> <td><input type="text" value="<%=cnt %>" name="cnt"></td>
</tr>
<tr>
<td colspan=2 align="center"><input type="submit" value="수정하기"></td>
</tr>
</table>
</div>
</body>
</html>
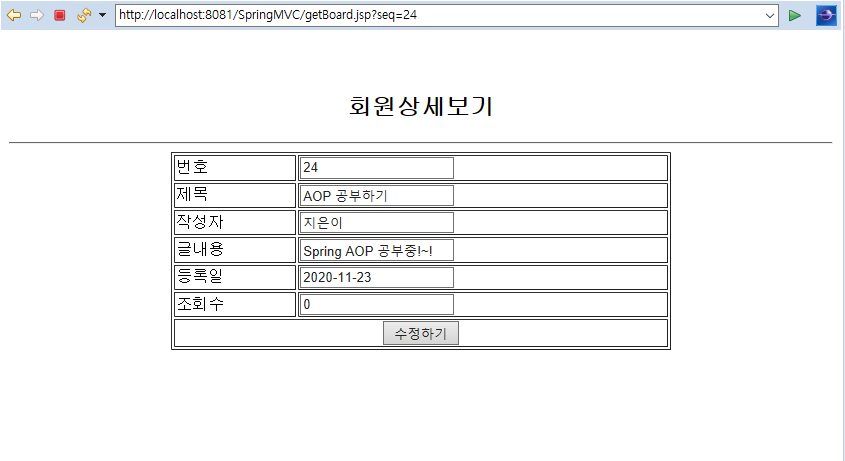