1. Thread 동기화 (Synchronized)
- synchronized 생략 시, 무분별하게 값 출력
( display - for문 1번 진행 후, 다른 Thread 값 무분별하게 실행 )
- synchronized 함수를 통해 하나의 Thread가 실행을 완료될 때까지 기다림.
public class threadTest3 {
public static void main(String[] args) throws Exception {
System.out.print(Thread.currentThread().getName() + ":");
System.out.println();
StringPrint sp = new StringPrint();
Thread th1 = new PrintThread("1", sp);
Thread th2 = new PrintThread("2", sp);
Thread th3 = new PrintThread("3", sp);
Thread th4 = new PrintThread("4", sp);
Thread th5 = new PrintThread("5", sp);
th1.start();
th2.start();
th3.start();
th4.start();
th5.start();
}
}
class StringPrint {
synchronized void display(String s) {
for (int i = 1; i <= 5; i++) {
System.out.print(Thread.currentThread().getName() + ":");
System.out.print(s);
System.out.println();
}
System.out.println();
}
}
class PrintThread extends Thread {
private StringPrint sp = new StringPrint();
private String str;
public PrintThread(String s, StringPrint sp) {
str = s;
this.sp = sp;
}
@Override
public void run() {
sp.display(str);
}
}
2. 아래의 네트웍 객체에 대하여 설명하시오.
📌InetAddress
- 호스트(Host)이름과 IP 주소를 보여주는 클래스
- InetAddress 클래스의 객체는 getByName() 함수의 매개변수에 호스트의 이름을 사용하여 생성
InetAddress ip = InetAddress.getByName("OOO.cafe24.com")
- getHostName(); - 호스트명 반환
- getHostAddress(); - IP 주소 반환
📌 URLConnection
📌 Socket
📌SeverSocket
3. 아래를 실행해보고, balance 가 마이너스 금액이 찍히는 이유를 적고, 코드를 수정해 보시오.
class Ex13_12 {
public static void main(String args[]) {
Runnable r = new RunnableEx12();
new Thread(r).start();
new Thread(r).start();
}
}
class Account {
private int balance = 1000;
public int getBalance() {
return balance;
}
public void withdraw(int money){
if(balance >= money) {
try { Thread.sleep(1000);} catch(InterruptedException e) {}
balance -= money;
}
}
}
class RunnableEx12 implements Runnable {
Account acc = new Account();
public void run() {
while(acc.getBalance() > 0) {
int money = (int)(Math.random() * 3 + 1) * 100;
acc.withdraw(money);
System.out.println("balance:"+acc.getBalance());
}
}
}
- 마이너스가 나오는 원인
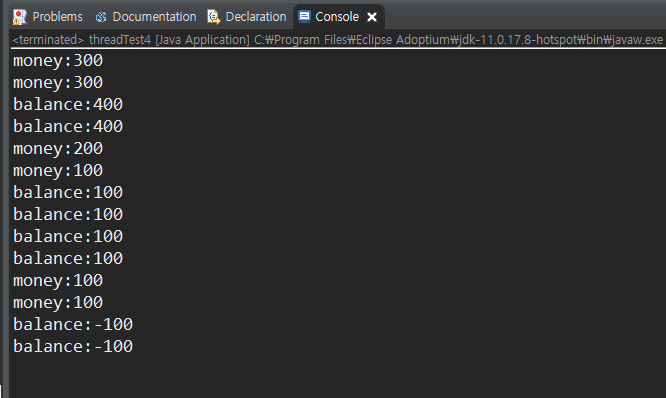
- 동기화가 되어있지 않아서 함수가 무분별하게 적용되어 오류 발생
- Account 클래스의 withdraw 메소드에 synchronized를 넣어 무분별한 값 계산을 막는다.