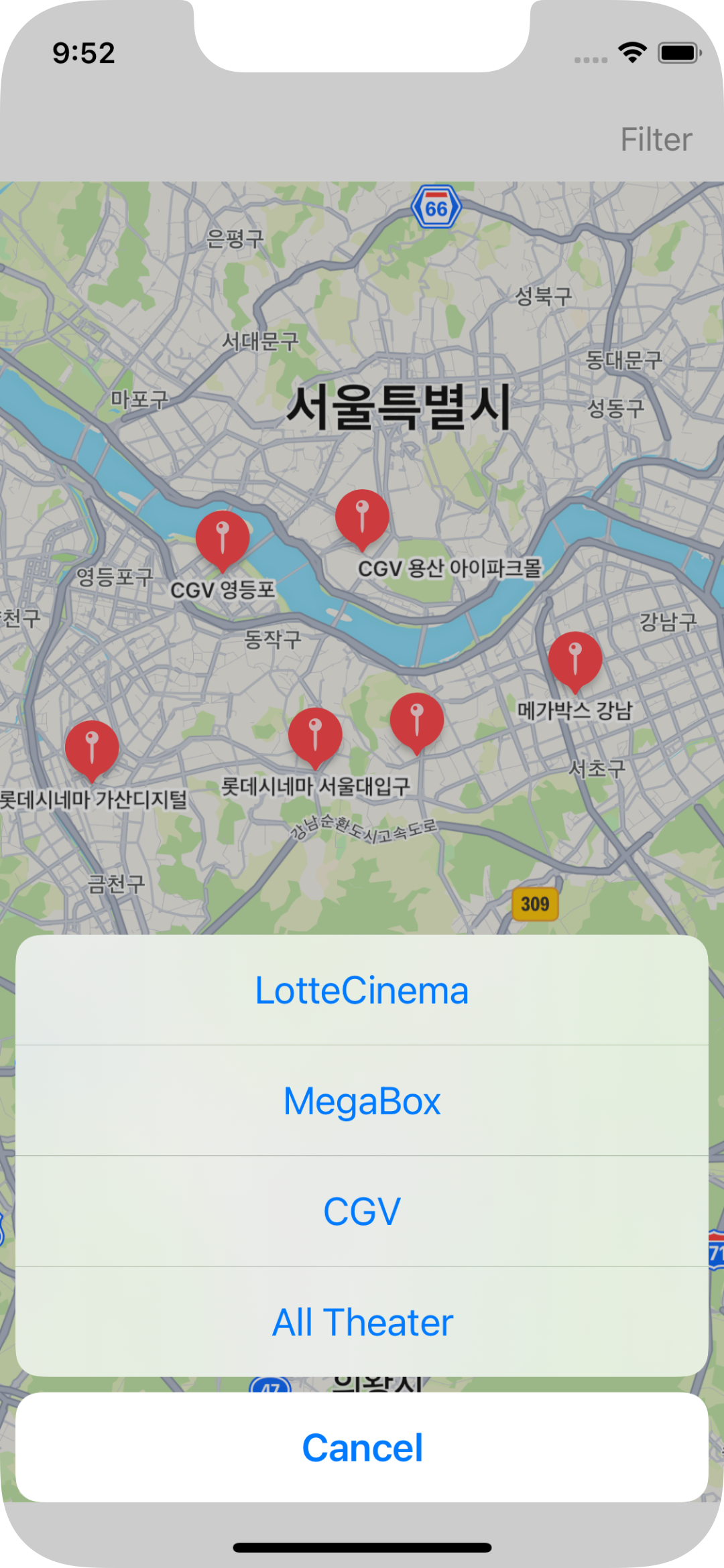
- 단계
1) MapKit, CoreLocation(현재 위치 등을 표현
2) 위치에 대한 담당 객체 CLLocationManager 호출
3) CLLocationManager 프로토콜 선언/연결
4) 위치를 성공적으로 가져온 경우/ 실패한 경우 실행될 코드 작성
5) iOS버전에 따른 분기 처리 / 위치서비스 온=권한요청, 오프=얼럿으로 알려주기
6) 사용자의 위치 '권한' 상태 확인
7) 사용자의 권한 상태가 바뀔때를 알려주기
import UIKit
import MapKit
import CoreLocation
class MapViewController: UIViewController {
@IBOutlet weak var mapView: MKMapView!
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
locationManager.delegate = self
let center = CLLocationCoordinate2D(latitude: 37.517829, longitude: 126.886270)
setRegionAndAnnotation(center: center)
}
func setRegionAndAnnotation(center: CLLocationCoordinate2D){
let region = MKCoordinateRegion(center: center, latitudinalMeters: 1000, longitudinalMeters: 1000)
mapView.setRegion(region, animated: true)
let annotation = MKPointAnnotation()
annotation.coordinate = center
annotation.title = "이곳이 영등포 캠퍼스"
mapView.addAnnotation(annotation)
}
}
extension MapViewController{
func checkUserDeviceLocationServiceAuthorization(){
let authorizationStatus: CLAuthorizationStatus
if #available(iOS 14.0, *){
authorizationStatus = locationManager.authorizationStatus
} else {
authorizationStatus = CLLocationManager.authorizationStatus()
}
if CLLocationManager.locationServicesEnabled(){
checkUserCurrentLocationAuthorization(authorizationStatus)
} else {
print("위치서비스가 꺼져있어 권한을 요청하지 못 합니다.")
}
}
func checkUserCurrentLocationAuthorization(_ authorizationStatus: CLAuthorizationStatus){
switch authorizationStatus {
case .notDetermined:
print("NotDetermined")
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
case .restricted, .denied:
print("Denied, 아이폰 설정으로 유도")
showRequestLocationServiceAlert()
case .authorizedWhenInUse:
print("When In Use")
locationManager.startUpdatingLocation()
default: print("Default")
}
}
func showRequestLocationServiceAlert() {
let requestLocationServiceAlert = UIAlertController(title: "위치정보 이용", message: "위치 서비스를 사용할 수 없습니다. 기기의 '설정>개인정보 보호'에서 위치 서비스를 켜주세요.", preferredStyle: .alert)
let goSetting = UIAlertAction(title: "설정으로 이동", style: .destructive) { _ in
if let appSetting = URL(string: UIApplication.openSettingsURLString){
UIApplication.shared.open(appSetting)
}
}
let cancel = UIAlertAction(title: "취소", style: .default)
requestLocationServiceAlert.addAction(cancel)
requestLocationServiceAlert.addAction(goSetting)
present(requestLocationServiceAlert, animated: true, completion: nil)
}
}
extension MapViewController: CLLocationManagerDelegate{
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
print(#function, locations)
if let coordinate = locations.last?.coordinate{
setRegionAndAnnotation(center: coordinate)
}
locationManager.stopUpdatingLocation()
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
print(#function)
}
func locationManagerDidChangeAuthorization(_ manager: CLLocationManager) {
print(#function)
checkUserDeviceLocationServiceAuthorization()
}
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
}
}
extension MapViewController: MKMapViewDelegate{
func mapView(_ mapView: MKMapView, regionDidChangeAnimated animated: Bool) {
}
}