emplist 검색
# Controller
package jspexp.a01_mvc;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import jspexp.a13_database.vo.Emp;
/**
* Servlet implementation class A07_EmpController
*/
@WebServlet(name = "empList.do", urlPatterns = { "/empList.do" })
public class A07_EmpController extends HttpServlet {
private static final long serialVersionUID = 1L;
private A08_EmpService service;
public A07_EmpController() {
service = new A08_EmpService();
}
/**
* @see HttpServlet#service(HttpServletRequest request, HttpServletResponse response)
*/
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// post 방식 한글 요청값 처리시 인코딩 필요, java단 안에서 문자열을 비교해야할 때 인코딩
request.setCharacterEncoding("utf-8");
String ename = request.getParameter("ename");
String job = request.getParameter("job");
String frSalS = request.getParameter("frSal");
String toSalS = request.getParameter("toSal");
// 모델 데이터 선언
// 생성자를 통해서 서비스단 객체 생성
request.setAttribute("sch",service.getEmp(ename,job,frSalS,toSalS));
request.setAttribute("empList", service.empList()); // Model은 View단에 넘길 핵심 데이터
String page = "WEB-INF\\a01_mvc\\a07_empList.jsp";
request.getRequestDispatcher(page).forward(request, response);
}
}
# Service
package jspexp.a01_mvc;
import java.util.List;
import com.google.gson.Gson;
import jspexp.a13_database.A02_EmpDao;
import jspexp.a13_database.vo.Emp;
// 주요 핵심 로직 처리 후, 모델로 사용할 핵심 데이터를 리턴
public class A08_EmpService{
private A02_EmpDao dao;
private Emp sch;
public A08_EmpService() {
dao = new A02_EmpDao();
}
// array/object ==> json문자열로 변환
public String empAjax(String ename,String job, String frSalS, String toSalS) {
sch = getEmp(ename, job, frSalS, toSalS);
Gson g = new Gson();
return g.toJson(empList());
}
public Emp getEmp(String ename,String job, String frSalS, String toSalS) {
if(ename==null) ename="";
if(job==null) job="";
if(frSalS==null || frSalS.equals("")) frSalS="0";
if(toSalS==null || toSalS.equals("")) toSalS="9999";
double frSal = Double.parseDouble(frSalS);
double toSal = Double.parseDouble(toSalS);
sch = new Emp(ename,job,frSal,toSal);
return sch;
}
public List<Emp> empList(){
return dao.getEmpSch(sch);
}
}
# View
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"
import="java.util.*"%>
<%@ taglib prefix ="c" uri ="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix = "fmt" uri = "http://java.sun.com/jsp/jstl/fmt"%>
<fmt:requestEncoding value="utf-8"/>
<% request.setCharacterEncoding("utf-8"); %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link href="/a00_com/a01_common.css" rel="stylesheet">
</head>
<body>
<%--WEB-INF 하위에 있는 jsp는 url로 접근할 수 없다 Controller만 접근이 가능 --> 보안성이 높다 --%>
<h2>사원정보</h2>
<form method="post">
사원명 : <input type="text" name="ename" value="${sch.ename}">
직책 : <input type="text" name="job" value="${sch.job}">
급여범위 : <input type="text" name="frSal" value="${sch.frSal}">
~ <input type="text" name="toSal" value="${sch.toSal}">
<input type="submit" value="검색"/> <br>
</form>
<table>
<tr><th>사원번호</th><th>사원명</th><th>직책</th><th>관리자</th><th>입사일자</th><th>연봉</th><th>보너스</th></tr>
<c:forEach var="emp" items="${empList}">
<tr><td>${emp.empno}</td>
<td>${emp.ename}</td>
<td>${emp.job}</td>
<td>${emp.mgr}</td>
<td><fmt:formatDate value="${emp.hiredate}"/> </td>
<td><fmt:formatNumber value="${emp.sal}"/> </td>
<td><fmt:formatNumber value="${emp.comm}"/> </td>
</tr>
</c:forEach>
</table>
</body>
</html>
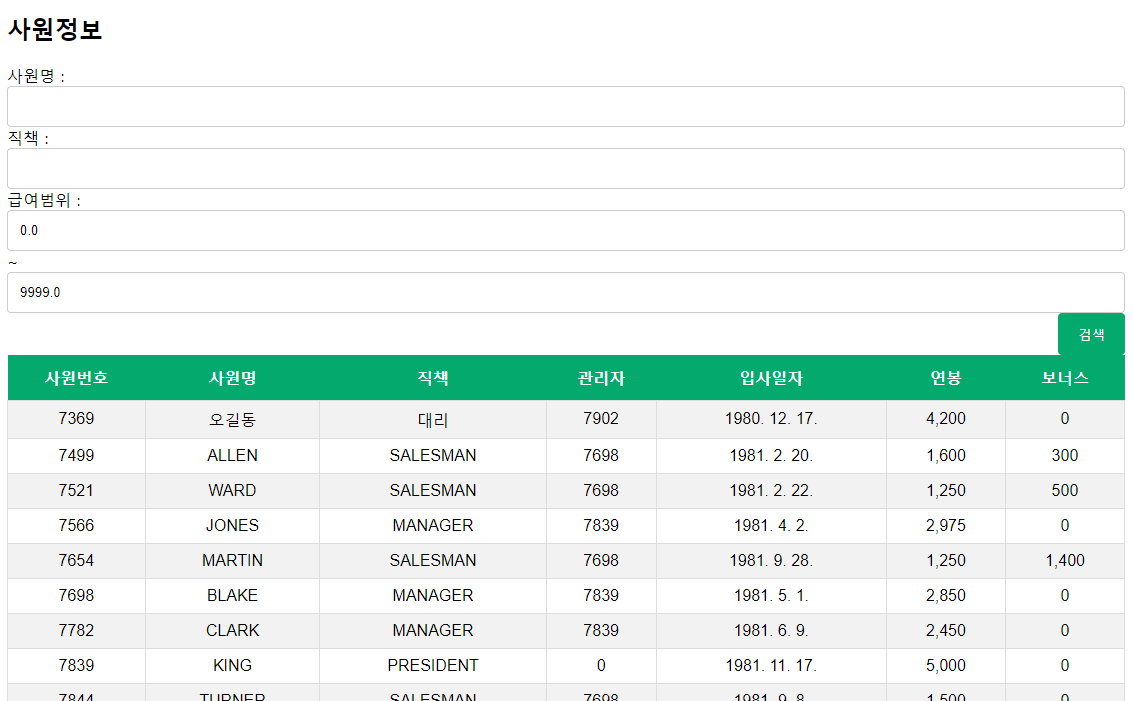