type ReturnedActions<T> = {
[P in keyof T]: T[P] extends (...a: any) => infer R ? {
type: P,
payload: R
} : never
}[keyof T]
type AllActions = ReturnedActions<Actions>
Playground link
Edit If Actions only contains functions, you can also use the distributive nature of ReturnType to get the return type of all functions in Actions:
type AllActions = ReturnType<Actions[keyof Actions]>;
type ReturnedActions<T> = {
[P in keyof T]: T[P] extends (...args: any) => infer R ? {
type:P,
payload:R
}:never
}[keyof T]
class Test {
constructor(){}
hello():void{
console.log('hello')
}
num():number{
return 1
}
}
type TestAction = ReturnType<Test[keyof Test]>
type Ret = ReturnedActions<Test>
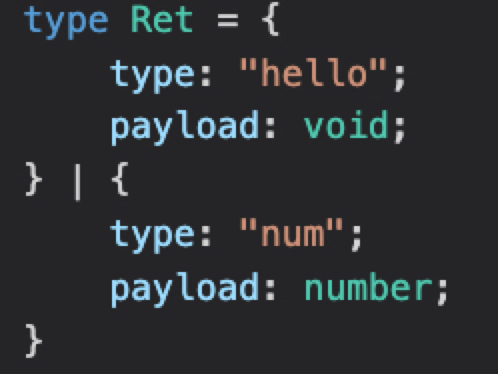
class Drill {
constructor() {}
consoleHi(): string {
return 'Hi';
}
}
type DrillAction = ReturnType<Drill[keyof Drill]>;