formData.append('key', value);
const saveFileImage = async e => {
try {
const formData = new FormData();
formData.append('image', e.target.files[0]);
const config = {
headers: {
Authorization: ...,
'Content-Type': 'multipart/form-data',
},
};
const response = await axios.post(`api url`, formData, config);
} catch (error) {
}
};
🪴 로컬에 있는 이미지를 업로드하기
import { useRef } from 'react';
const thumbnailInput = useRef();
const saveFileImage...
<input type='file' accept='image/jpg, image/jpeg, image/png' multiple ref={thumbnailInput} onChange={saveFileImage} />
const handleClick = () => {
thumbnailInput.current.click();
};
<button onClick={handleClick}>
썸네일 업로드
<input type='file' accept='image/jpg, image/jpeg, image/png' multiple ref={thumbnailInput} onChange={saveFileImage} style={{ display: 'none' }} />
</button>
const saveFileImage = async e => {
try {
const formData = new FormData();
formData.append('image', e.target.files[0]);
const config = {
headers: {
Authorization: ...,
'Content-Type': 'multipart/form-data',
},
};
const response = await axios.post(`api url`, formData, config);
dispatch(setWriteContent({ type: 'thumbnail', value: `${response.data.imageUrl[0]}` }));
} catch (error) {
}
};
👉 서버에서 받아온 이미지 url을 img 태그 src에 부여하여 이미지 보여주기
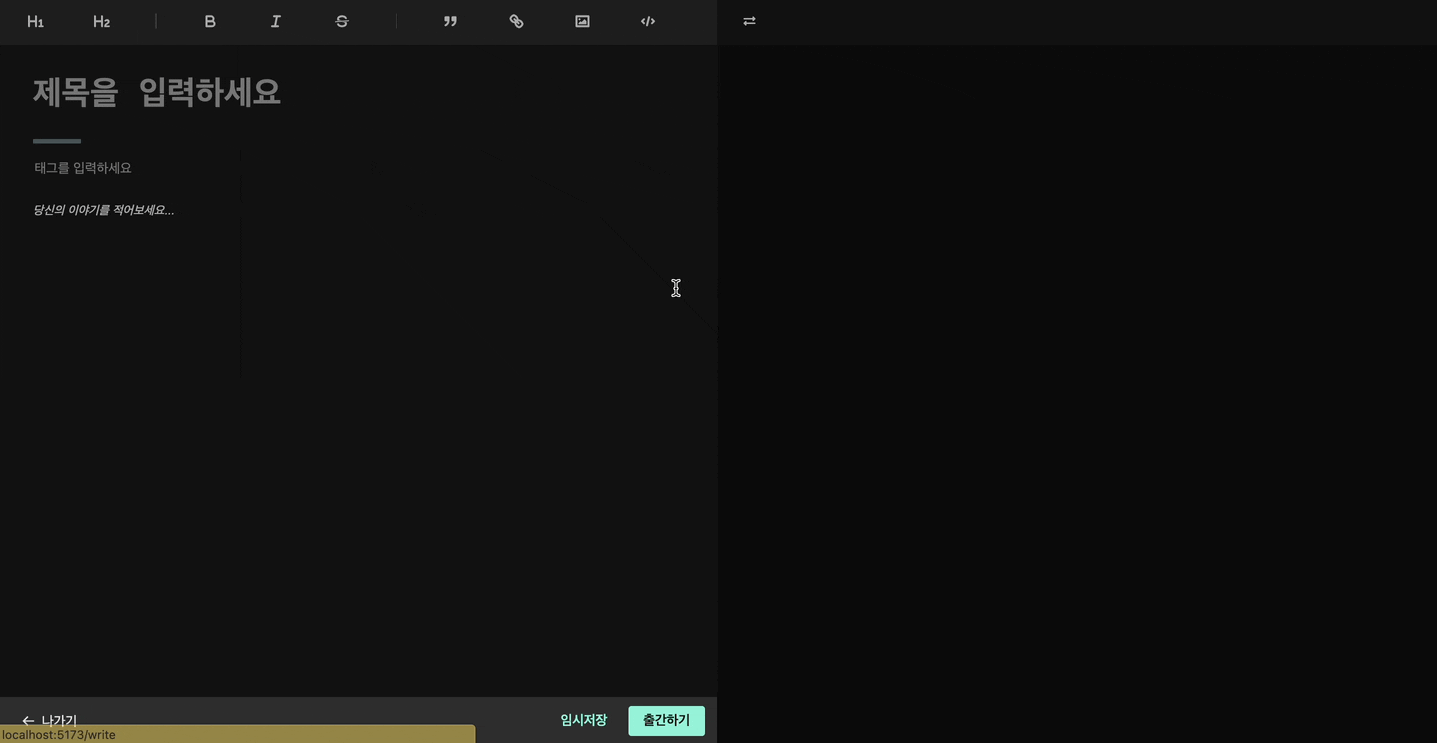
<img src={`서버에 등록된 이미지 주소`} alt='thumbnail' />