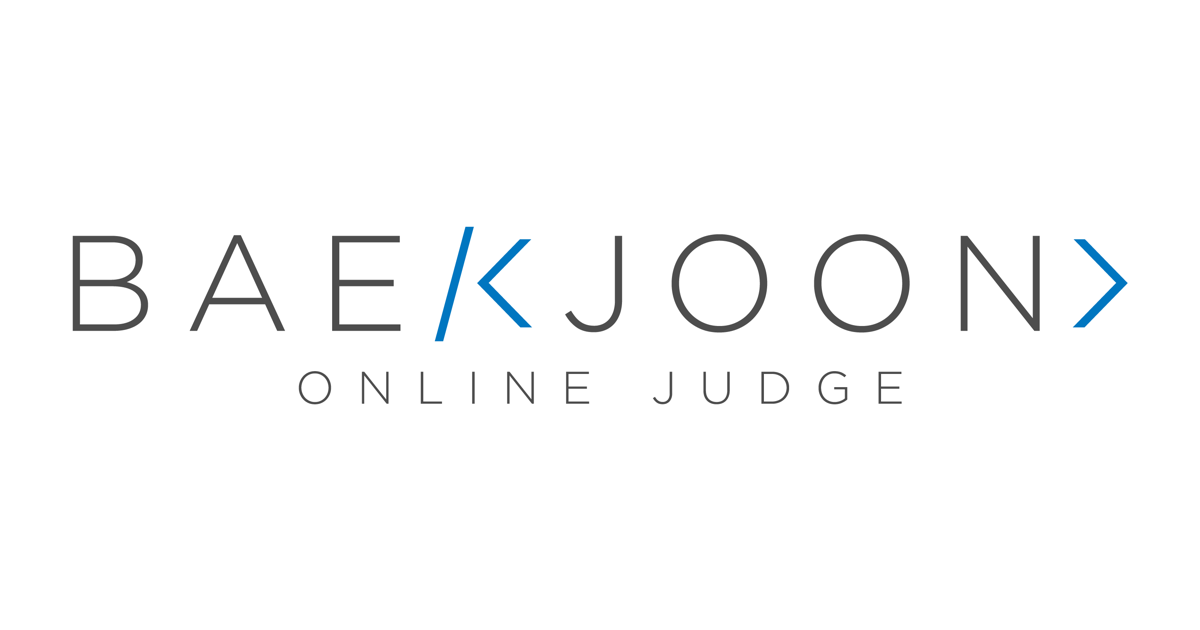
문제
HOW TO SOLVE
- 접미사 배열 : 단순 구현
- 늑대와 양 : 단순 구현
- 두 개의 배열 : 이진탐색 + etc ( 못 품 ㅠㅠ )
- 파티 : 다익스트라 알고리즘
접미사 배열
s = input()
suffixs = []
for i in range(len(s)):
suffixs.append(s[i:])
for suffix in sorted(suffixs):
print(suffix)
늑대와 양
'''
- 늑대가 양이 있는 칸으로 가지 못한다 -> 1출력, 목장의 상태 출력
- 늑대가 양이 있는 칸으로 갈 수 있다 -> 0출력
-> 울타리는 아무렇게나 설치해도 되나?
'''
r, c = map(int, input().split())
_graph = []
dx, dy = [-1, 1, 0, 0], [0, 0, -1, 1]
for _ in range(r):
_graph.append(list(input()))
sheeps = set()
wolves = set()
for i in range(r):
for j in range(c):
if _graph[i][j] == 'S': sheeps.add((i, j))
elif _graph[i][j] == 'W': wolves.add((i, j))
def is_range(x, y):
return 0 <= x < r and 0 <= y < c
def solve():
for x, y in sheeps:
for i in range(4):
nx, ny = x + dx[i], y + dy[i]
if is_range(nx, ny) and _graph[nx][ny] == '.':
_graph[nx][ny] = 'D'
for x, y in wolves:
for i in range(4):
nx, ny = x + dx[i], y + dy[i]
if is_range(nx, ny) and _graph[nx][ny] == 'S':
return False
return True
ret = solve()
if not ret: print(0)
else:
print(1)
for i in range(r):
for j in range(c):
print(_graph[i][j], end='')
print()
두 개의 배열
Not Yet 🤮
파티
'''
- 다익스트라 알고리즘을 이용하여 풀이 -> 특정 시작점에서 다른 모든 노드로 가는 최단 경로를 구해야 함
- 시작점 x라고 가정, x -> i -> x 에 걸리는 최대 시간을 구하면 됨
'''
import sys
import heapq
input = sys.stdin.readline
INF = int(1e9)
n, m, x = map(int, input().split())
graph = [[] for _ in range(n + 1)]
for _ in range(m):
a, b, c = map(int, input().split())
graph[a].append((b, c))
def dijkstra(s, e):
q = []
heapq.heappush(q, (0, s))
dist = [INF] * (n + 1)
dist[s] = 0
while q:
d, cur = heapq.heappop(q)
if dist[cur] < d:
continue
for nxt in graph[cur]:
cost = d + nxt[1]
if cost < dist[nxt[0]]:
dist[nxt[0]] = cost
heapq.heappush(q, (cost, nxt[0]))
return dist[e]
_max = 0
for s in range(1, n + 1):
go = dijkstra(s, x)
come = dijkstra(x, s)
_max = max(_max, go + come)
print(_max)