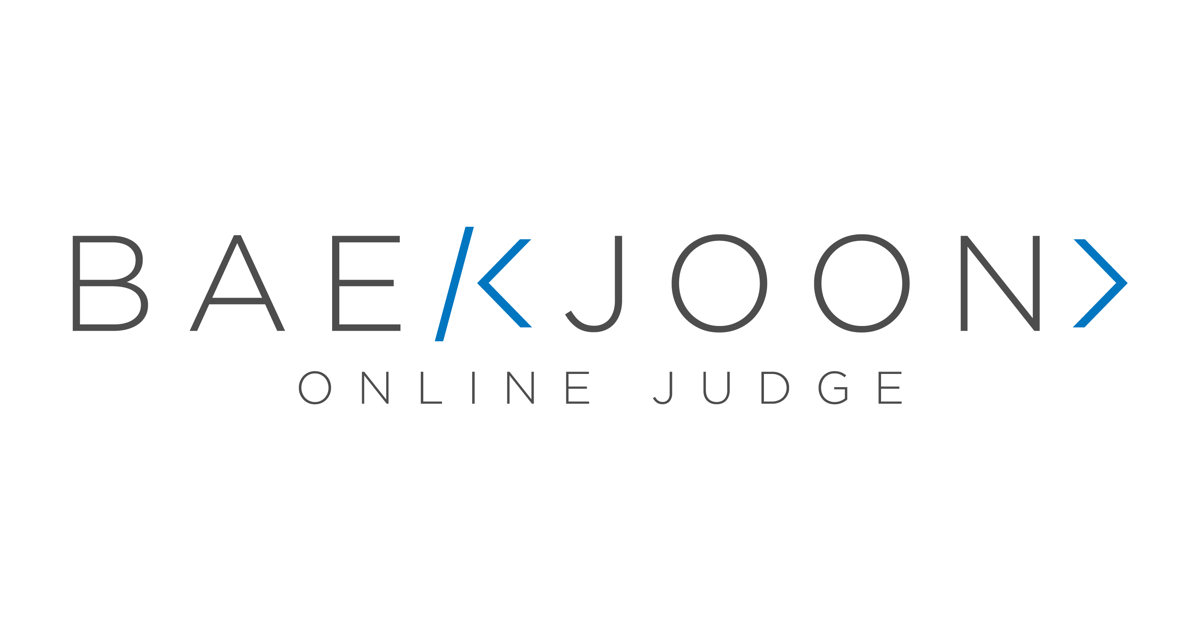
문제
HOW TO SOLVE
- 피보나치 함수 : DP
- 두 스티커 : 단순 구현
- 미세먼지 안녕!: 단순 구현
- 프렉탈 평면 : 재귀 ( 못 품 ㅠ )
피보나치 함수
'''
- 재귀 -> TLE
'''
def fibo(n):
if n == 0: return (1, 0)
dp = [(0, 0) for _ in range(n + 1)]
dp[0] = (1, 0)
dp[1] = (0, 1)
for i in range(2, n + 1):
dp[i] = (dp[i - 1][0] + dp[i - 2][0], dp[i - 1][1] + dp[i - 2][1])
return dp[n]
t = int(input())
for _ in range(t):
n = int(input())
one, zero = fibo(n)
print(one, zero)
두 스티커
'''
- 스티커는 90도 회전 가능 -> 가로와 세로가 바뀐다는 뜻
- 스티커가 모눈종이를 벗어나면 안됨
- 스티커는 겹치면 안됨
- 총 2개의 스티커를 붙여야 함
- 스티커를 못붙이면 0을 출력
'''
h, w = map(int, input().split())
n = int(input())
stickers = [list(map(int, input().split())) for _ in range(n)]
_max = 0
for i in range(n - 1):
for j in range(i + 1, n):
h1, w1 = stickers[i]
h2, w2 = stickers[j]
if (w1 + w2 <= w and max(h1, h2) <= h) or (h1 + h2 <= h and max(w1, w2) <= w):
_max = max(_max, h1 * w1 + h2 * w2)
if (h1 + w2 <= w and max(w1, h2) <= h) or (w1 + h2 <= h and max(h1, w2) <= w):
_max = max(_max, h1 * w1 + h2 * w2)
if (w1 + h2 <= w and max(h1, w2) <= h) or (h1 + w2 <= h and max(w1, h2) <= w):
_max = max(_max, h1 * w1 + h2 * w2)
if (h1 + h2 <= w and max(w1, w2) <= h) or (w1 + w2 <= h and max(h1, h2) <= w):
_max = max(_max, h1 * w1 + h2 * w2)
print(_max)
미세먼지 안녕!
from collections import deque
def spread():
dx, dy = (-1, 1, 0, 0), (0, 0, -1, 1)
tmp = [[0] * c for _ in range(r)]
for x in range(r):
for y in range(c):
if graph[x][y] == 0 or graph[x][y] == -1: continue
d = graph[x][y] // 5
for i in range(4):
nx, ny = x + dx[i], y + dy[i]
if 0 <= nx < r and 0 <= ny < c and graph[nx][ny] != -1:
tmp[nx][ny] += d
tmp[x][y] -= d
for x in range(r):
for y in range(c):
graph[x][y] += tmp[x][y]
def air_top():
dx, dy = (0, -1, 0, 1), (1, 0, -1, 0)
x, y, d = up, 1, 0
prev = 0
while 1:
nx, ny = x + dx[d], y + dy[d]
if x == up and y == 0: break
if not 0 <= nx < r or not 0 <= ny < c:
d += 1
continue
graph[x][y], prev = prev, graph[x][y]
x, y = nx, ny
def air_bottom():
dx, dy = (0, 1, 0, -1), (1, 0, -1, 0)
x, y, d = down, 1, 0
prev = 0
while 1:
nx, ny = x + dx[d], y + dy[d]
if x == down and y == 0: break
if not 0 <= nx < r or not 0 <= ny < c:
d += 1
continue
graph[x][y], prev = prev, graph[x][y]
x, y = nx, ny
r, c, t = map(int, input().split())
graph = []
for _ in range(r):
data = list(map(int, input().split()))
graph.append(data)
up, down = 0, 0
for i in range(r):
if graph[i][0] == -1:
up, down = i, i + 1
break
for _ in range(t):
spread()
air_top()
air_bottom()
_sum = 0
for i in range(r):
_sum += sum(graph[i])
_sum += 2
print(_sum)
프렉탈 평면
🤮