1. 서블릿
- 서블릿은 톰캣 같은 웹 애플리케이션 서버를 직접 설치하고,그 위에 서블릿 코드를 클래스 파일로 빌드해서 올린 다음, 톰캣 서버를 실행하면 되지만, 번거롭기 때문에 톱캣 서버를 내장하고 있는 스프링부트를 이용해 서블릿코드 실행
1-1. 웹 애플리케이션 서버의 요청 응답 구조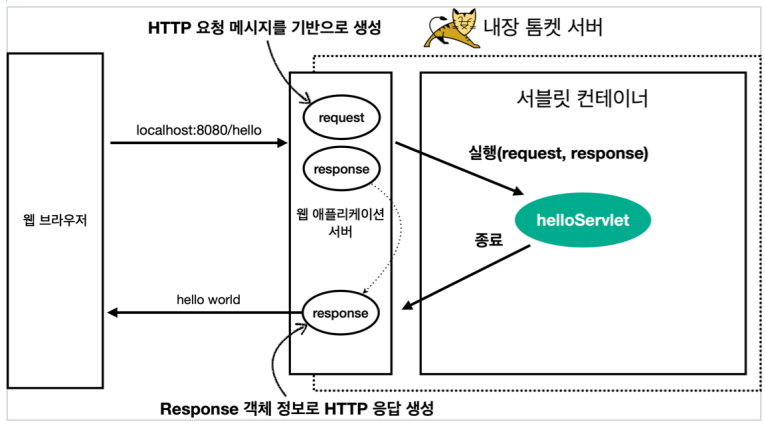
1-2. 스프링 부트 서블릿 환경 구성
@ServletComponentScan
@SpringBootApplication
public class ServletApplication {
public static void main(String[] args) {
SpringApplication.run(ServletApplication.class, args);
}
}
@ServletComponentScan
: 스프링이 자동으로 현재 내 패키지를 포함한 하위 패키지를 뒤져서 서블릿 자동 동륵시킴
1-3. 서블릿 등록하기
@WebServlet(name = "helloServlet", urlPatterns = "/hello")
public class HelloServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
response.setContentType("text/plain");
response.setCharacterEncoding("utf-8");
response.getWriter().write("hello " + username);
}
}
1-4. welcome page추가
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<ul>
<li><a href="basic.html">서블릿 basic</a></li>
</ul>
</body>
</html>
2. HttpServletRequest 및 HTTP 요청 데이터
2-1. HttpServletRequest 역할
- HTTP 요청 메시지를 파싱한 결과를 HttpServletRequest 객체에 담아서 제공(start-line, header 정보 등)
2-2. 방법1) GET - 쿼리 파라미터
- 메시지 바디 없이, URL의 쿼리 파라미터를 사용해서 데이터를 전달
- 검색, 필터, 페이징등에서 많이 사용
- 파라미터 전송 가능
- 동일한 파라미터 전송 가능
- 단일 파라미터:
request.getParameter()
- 복수 파라미터:
request.getParameterValues()
- 중복일 때 request.getParameter()을 사용하면 첫 번째 값 반환
2-3. 방법2) POST - HTML Form
- get query parameter에 있는 데이터 꺼내기
- post러 html form보낼 때, 내부에 있는 데이터 꺼낼 수 있음
- 주로 회원 가입, 상품 주문 등에서 사용하는 방식
- content-type(필수 지정): application/x-www-form-urlencoded
- 메시지 바디에 쿼리 파리미터 형식으로 데이터를 전달
request.getParameter()
사용해 데이터 조회
2-4. 방법3) HTTP message body에 데이터를 직접 담기
[ API 메시지 바디 - 단순 텍스트 ]
- HTTP message body에 내가 원하는 데이터 직접 담아 전송
- 데이터 형식은 주로 JSON 사용
- POST, PUT, PATCH
- InputStream을 이용해 HTTP message body의 데이터를 직접 읽을 수 있음
[ API 메시지 바디 - JSON ]
- content-type: application/json
- message body: {"username": "hello", "age": 20}
- JSON 결과를 파싱해서 사용할 수 있는 자바 객체로 변환하려면 Jackson, Gson 같은 JSON 변환 라이브러리를 추가해서 사용(ObjectMapper)
3. HttpServletResponse 및 HTTP 응답 데이터
3-1. HttpServletResponse 역할
- HTTP 응답 메시지 생성(HTTP 응답코드 지정, 헤더 생성, 바디 생성)
- 편의 기능 제공(content, 쿠키, redirect)
3-2. 단순 텍스트, HTML
- HTTP 응답으로 HTML을 반환할 때는 content-type을 text/html 로 지정
response.setContentType("text/html");
3-3. API JSON
- HTTP 응답으로 JSON을 반환할 때는 content-type을 application/json 로 지정
response.setHeader("content-type", "application/json");