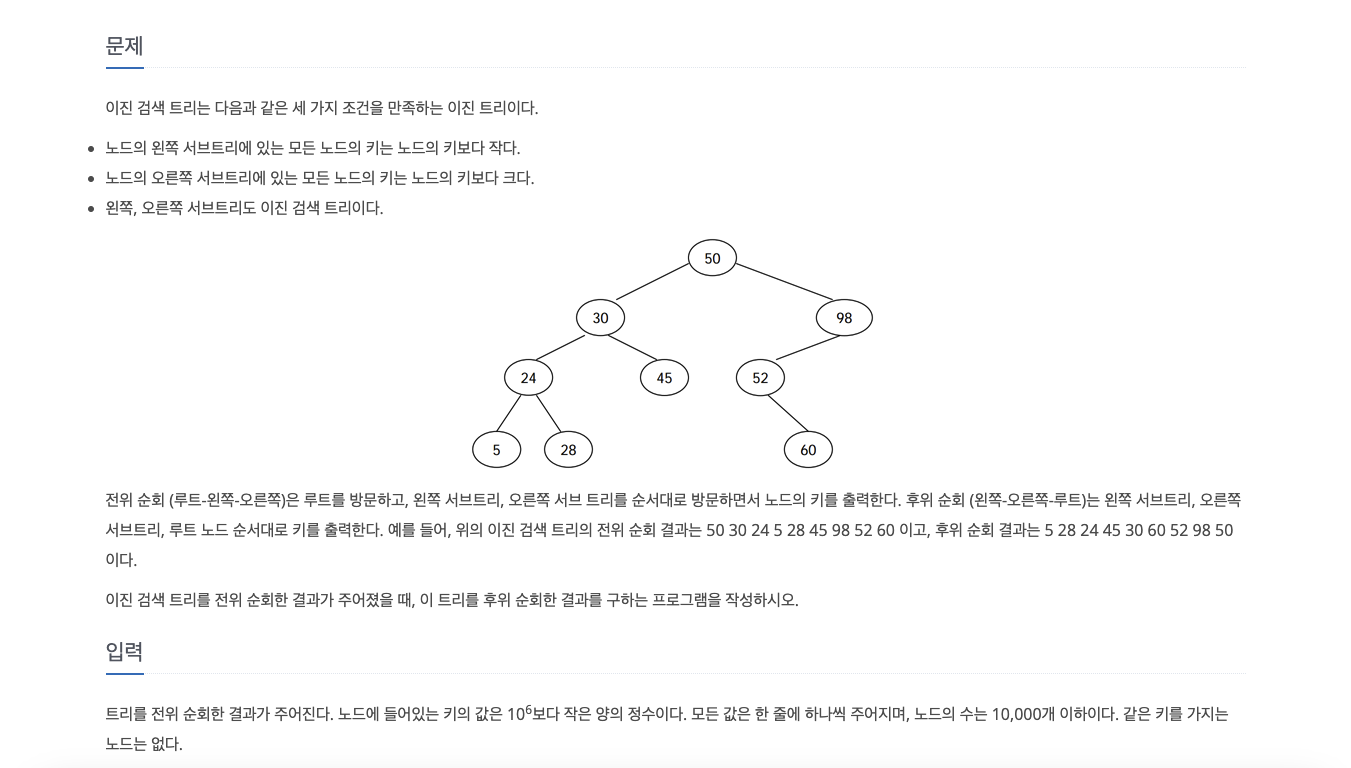
package task.gold.binarysearchtree5639;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static BinaryTree bt = new BinaryTree();
public static void main(String[] args) throws IOException {
String item = "";
while ((item = br.readLine()) != null) {
int i = Integer.parseInt(item);
bt.add(i);
}
bt.search();
}
public static class BinaryTree {
Node RootNode;
public void add(int value) {
if (RootNode == null) {
RootNode = new Node(value);
} else {
RootNode.add(value);
}
}
public void search() {
RootNode.search();
}
}
public static class Node {
int value;
Node left;
Node right;
public Node(int value) {
this.value = value;
}
public void add(int value) {
if (value <= this.value) {
if (this.left == null) {
this.left = new Node(value);
} else {
this.left.add(value);
}
} else {
if (this.right == null) {
this.right = new Node(value);
} else {
this.right.add(value);
}
}
}
public void search() {
if (this.left == null && this.right == null) {
System.out.println(this.value);
return;
}
if (this.left != null) {
this.left.search();
}
if (this.right != null) {
this.right.search();
}
System.out.println(this.value);
}
}
}
- 이진트리 직접 구현
- add 구현해서 전위 순회로 주어지는 값들 새로운 트리에 집어넣기 (재귀 방식)
- search 후위순회로 구현
- 재귀에만 익숙하면 금방 풀 수 있는 문제
- 딱히 할말이 없다