Redux 를 개량하여 기존 Redux 보다 더 편리하고 코드가 적다. 또한, Redux 의 구조나 패러다임이 모두 똑같다는 것이 특징이다.
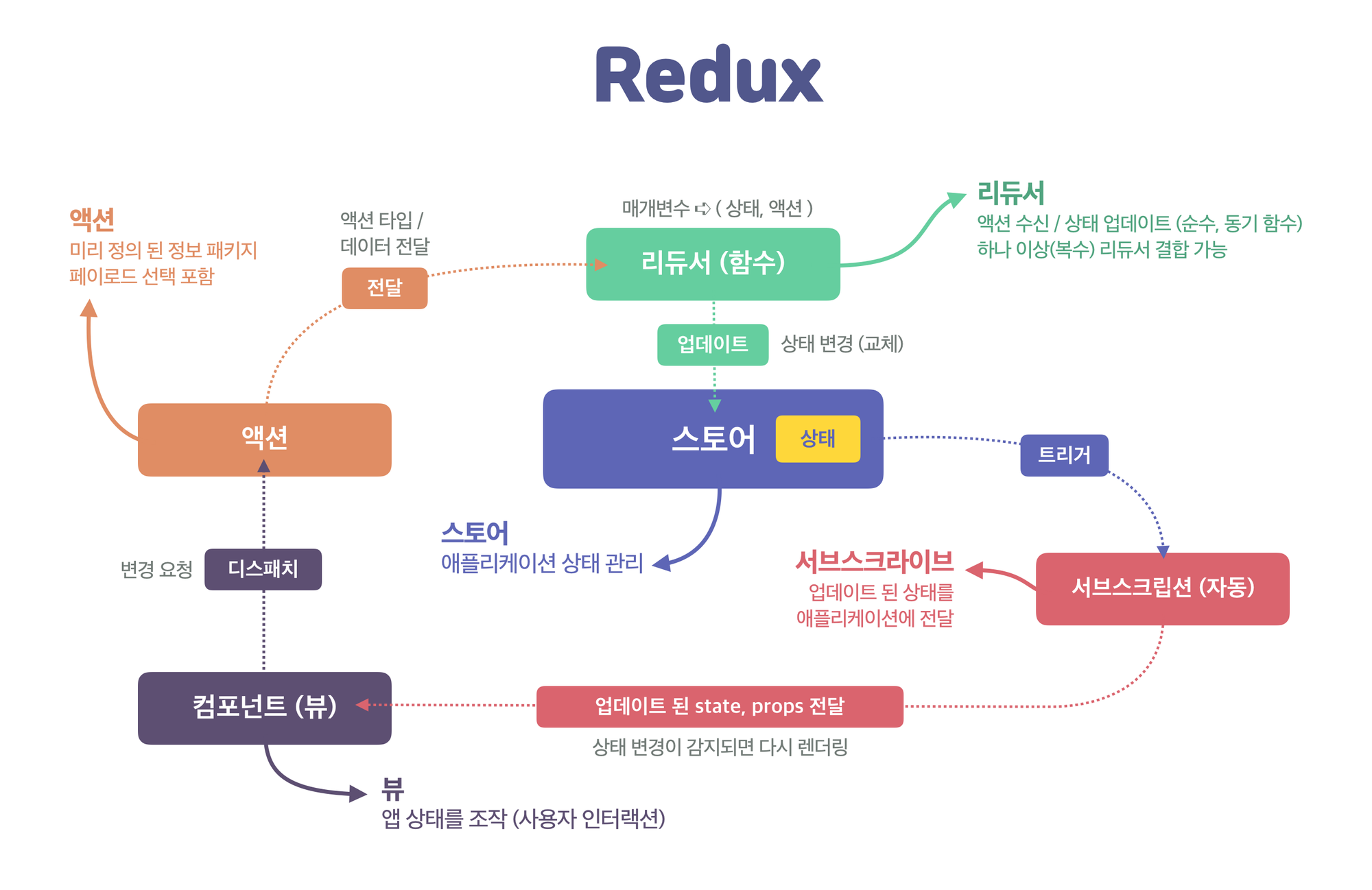
yarn add react-redux @reduxjs/toolkit
Redux를 사용하면 쓸 수 있는 개발툴로 현재 프로젝트의 state 상태라던가, 어떤 액션이 일어났을 때 그 액션이 무엇이고, 그것으로 인해 state가 어떻게 변경되었는지 등 리덕스를 사용하여 개발할 때 아주 편리하게 사용할 수 있다.
💡json-server
DB 와 API 서버를 간단하게 생성해주는 패키지. json-server를 통해서 프론트엔드에서는 백엔드가 하고 있는 작업을 기다리지 않고, 프론트엔드의 로직과 화면을 구현 할 수 있어 효율적으로 협업을 할 수 있다.
yarn add json-server
💡axios
http를 이용해서 서버와 통신하기 위해 사용하는 패키지.
브라우저와 Node.js에서 모두 사용 가능하고, 요청을 보내기 전이나 서버로부터 응답을 받은 후에 특정 코드를 실행할 수 있는 인터셉터 기능을 제공한다. 또한 서버로부터 받은 응답을 자동으로 JSON 으로 변환해주므로, 별도의 파싱 과정 없이 데이터를 쉽게 사용할 수 있다. 기존에 많이 사용하던 fetch 는 브라우저 api이기 때문에 Node.js 서버에서 사용할 수 없다.
yarn add axios
🧵 axios 사용법
import axios from 'axios';
async function fetchTodos() {
const response = await axios.get('https://jsonplaceholder.typicode.com/todos');
console.log(response.data);
}
async function createTodos() {
const response = await axios.post('https://jsonplaceholder.typicode.com/todos', {
title: 'Learn React',
content: 'too hard to learn'
});
console.log(response.data);
}
- axios get
get은 서버의 데이터를 조회할 때 사용
async function fetchTodos() {
const response = await axios.get('https://api.example.com/todos');
console.log(response.data);
}
- axios post
post 는 서버에서 데이터를 추가할 때 사용
async function createTodo() {
const newTodo = { title: 'Learn React', content: 'too hard to learn' };
const response = await axios.post('https://api.example.com/todo', newTodo);
console.log(response.data);
}
- axios delete
delete는 저장되어 있는 데이터를 삭제하고자 요청을 보낼 때 사용
async function deleteUser(todoId) {
const response = await axios.delete(`https://api.example.com/todos/${todoId}`);
console.log(response.data);
}
- axios update (put/patch)
patch 는 어떤 데이터를 수정하고자 서버에 요청을 보낼 때 사용
put 은 데이터 전체를 덮어 씌워서 업데이트하고, patch는 데이터의 일부를 덮어 씌워서 업데이트 함.
async function updateUser(todoId, updatedData) {
const response = await axios.patch(`https://api.example.com/todos/${todoId}`, updatedData);
console.log(response.data);
}
async function updateUser(todoId, updatedData) {
const response = await axios.put(`https://api.example.com/todos/${todoId}`, updatedData);
console.log(response.data);
}