1. 외부 데이터 사용
01. 외부 데이터 다루는 방식
- 앱이 원격 서비스에게 데이터를 요청
- 원격 서비스는 요청을 수신하고 요청된 데이터를 돌려보낸다
- 앱이 그 데이터를 받는다
- 앱은 받은 데이터를 가공해 사용자에게 보여준다
02. 웹 요청에 관한 기초
- 인터넷은 서로 연결된 엄청난 수의 컴퓨터, 즉 서버들로 구성되어 있다
- 모든 통신과정은 HTTP라는 프로토콜 때문에 가능하다
- HTTP는 브라우저, 또는 그런 종류의 클라이언트와 인터넷의 서버가 통신할 수 있는 공통의 언어를 제공한다
- 사용자를 대신해 브라우저가 HTTP를 활용해 만드는 요청을 HTTP Request(요청)이라고 하며, 그 요청은 새 페이지가 로딩될 때까지 유지된다
- HTTP 요청 예시
- 위의 예시처럼 페이지를 다시 로딩하지 않고 비동기식 요청과 데이터를 처리하고 수행하는 기술을 AJAX라고 하며, 이는 비동기식 자바스크립트와 XML의 줄임말이다
- 자바스크립트에서 HTTP 요청을 보내거나 받는 책임을 지는 객체는 기묘한 이름의 XMLHttpRequest다
- 서버로 요청을 전송
- 요청의 상태 확인
- 응답을 수신하고 파싱
- 요청의 상태와 상호작용할 수 있게하는, readystatechange라는 이벤트 리스닝 진행
03. 리액트 타임
-1. 시작하기
- create-react-app ipaddress
- public 폴더랑 src 폴더 안의 모든 파일을 삭제하고 깨끗이 시작합시다
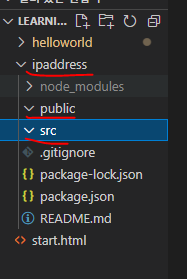
- public / index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>IP Address</title>
</head>
<body>
<div id="container"></div>
</body>
</html>
import React from "react";
import ReactDOM from "react-dom";
import "./index.css";
import IPAddressContainer from "./IPAddressContainer";
var destination = document.querySelector("#container");
ReactDOM.render(
<div>
<IPAddressContainer />
</div>,
destination
);
body {
background-color: #FFCC00;
}
-2. IP 주소 가져오기
- src / IPAddressContainer.js
import React, {Component} from 'react';
class IPAddressContainer extends Component {
render() {
return(
<p>Nothing yet!</p>
)
}
}
export default IPAddressContainer;
import React, { Component } from "react";
import "./IPAddress.css";
var xhr;
class IPAddressContainer extends Component {
constructor(props) {
super(props);
this.state = {
ip_address: "...",
};
this.processRequest = this.processRequest.bind(this);
}
componentDidMount() {
xhr = new XMLHttpRequest();
xhr.open("GET", "http://ipinfo.io/json", true);
xhr.send();
xhr.addEventListener("readystatechange", this.processRequest, false);
}
processRequest() {
if (xhr.readyState === 4 && xhr.status === 200) {
var response = JSON.parse(xhr.responseText);
this.setState({
ip_address: response.ip,
});
}
}
render() {
return (
<div>
<h1>{this.state.ip_address}</h1>
<p>( This is your IP address...probaly :P )</p>
</div>
);
}
}
export default IPAddressContainer;
- async / await로 갖고 왓어요! 동일한 비동기 방식이며 최근에 가장 많이 사용되는 비동기 방식 입니다
import React, { Component } from "react";
import "./IPAddress.css";
var xhr;
class IPAddressContainer extends Component {
constructor(props) {
super(props);
this.state = {
ip_address: "...",
};
this.processRequest = this.processRequest.bind(this);
}
componentDidMount() {
this.processRequest();
}
async processRequest() {
const ipData = await fetch("https://geolocation-db.com/json/");
const locationIp = await ipData.json();
this.setState({
ip_address: locationIp.IPv4,
});
}
render() {
return (
<div>
<h1>{this.state.ip_address}</h1>
<p>( This is your IP address...probaly :P )</p>
</div>
);
}
}
export default IPAddressContainer;
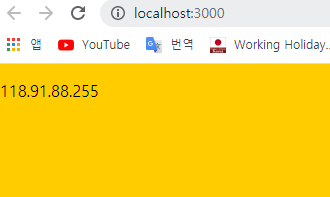
-3. 스타일 입히기
h1{
font-family: sans-serif;
text-align: center;
padding-top: 140px;
font-size: 60px;
margin: -15px;
}
p {
font-family: sans-serif;
color: #907400;
text-align: center;
}
- src / IPAddressContainer.js
import React, { Component } from "react";
import "./IPAddress.css";
var xhr;
class IPAddressContainer extends Component {
constructor(props) {
super(props);
this.state = {
ip_address: "...",
};
this.processRequest = this.processRequest.bind(this);
}
componentDidMount() {
// xhr = new XMLHttpRequest();
// xhr.open("GET", "https://ipinfo.io/json", true);
// xhr.send();
// xhr.addEventListener("readystatechange", this.processRequest, false);
this.processRequest();
}
async processRequest() {
const ipData = await fetch("https://geolocation-db.com/json/");
const locationIp = await ipData.json();
this.setState({
ip_address: locationIp.IPv4,
});
// if (xhr.readyState === 4 && xhr.status === 200) {
// var response = JSON.parse(xhr.responseText);
// this.setState({
// ip_address: response.ip,
// });
// }
}
render() {
return (
<div>
<h1>{this.state.ip_address}</h1>
<p>( This is your IP address...probaly :P )</p>
</div>
);
}
}
export default IPAddressContainer;
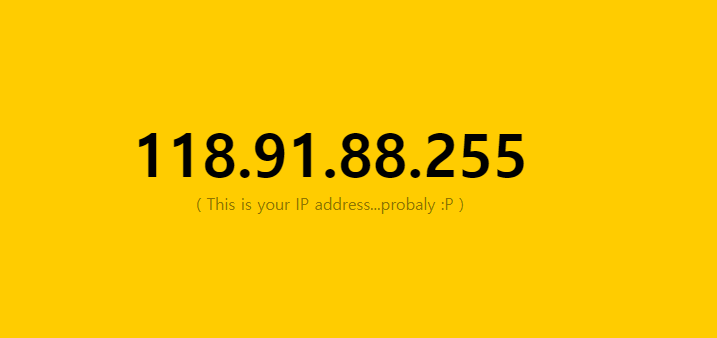