package com.company;
import java.util.Arrays;
class Solution {
static public void main(String[] args) {
int[] numbers = {1, 5, 2, 6, 3, 7, 4};
int[][] commands = {{2, 5, 3}, {4, 4, 1}, {1, 7, 3}};
int[] rtValArr = {5, 6, 3};
System.out.println(Arrays.equals(solution(numbers, commands), rtValArr));
}
static public int[] solution(int[] numbers, int[][] commands) {
int[] answer = new int[commands.length];
int index = 0;
for (int[] command : commands) {
int arrCutStart = command[0];
int arrCutFinal = command[1];
int answerIndex = command[2];
int[] notSortingArr = new int[arrCutFinal - arrCutStart + 1];
for (int i = (arrCutStart - 1), j = 0; i < arrCutFinal; i++, j++) {
notSortingArr[j] = numbers[i];
}
notSortingArr = sort(notSortingArr,true);
System.out.println(Arrays.toString(notSortingArr));
answer[index] = notSortingArr[answerIndex - 1];
index++;
}
return answer;
}
static private int[] sort(int[] arr, boolean ascending) {
int arrSize = 100;
int[] count = new int[arrSize];
for (int k : arr) {
count[k]++;
}
int[] answer = new int[arr.length];
for (int i = 0 , j = 0; i < arrSize; ) {
if(count[i] != 0){
answer[j] = i;
count[i]--;
j++;
}else{
i++;
}
}
return answer;
}
}
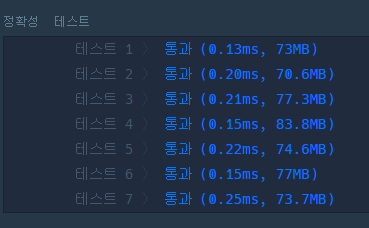