package com.company;
import java.util.Arrays;
class Solution {
static public void main(String[] args){
int[] numbers = {1, 5, 2, 6, 3, 7, 4};
int[][] commands = {{2, 5, 3},{4, 4, 1},{1, 7, 3}};
int[] rtValArr = {5, 6, 3};
System.out.println(Arrays.equals(solution(numbers, commands), rtValArr));
}
static public int[] solution(int[] numbers, int[][] commands) {
int[] answer = new int[commands.length];
int index = 0;
for (int[] command : commands) {
int arrCutStart = command[0];
int arrCutFinal = command[1];
int answerIndex = command[2];
int[] notSortingArr = new int[arrCutFinal - arrCutStart + 1];
for (int i = (arrCutStart - 1) , j = 0; i < arrCutFinal; i++,j++) {
notSortingArr[j] = numbers[i];
}
sort(notSortingArr,0, notSortingArr.length -1, true);
System.out.println(Arrays.toString(notSortingArr));
answer[index] = notSortingArr[answerIndex - 1];
index++;
}
return answer;
}
static private void sort(int[] arr, int start, int end, boolean ascending) {
if (start >= end) {
return;
}
int pivot = start;
int left = start + 1;
int right = end;
while (left <= right) {
while(left <= end && arr[left] <= arr[pivot])
left = left + 1;
while(right > start && arr[right] >= arr[pivot])
right = right - 1;
if(left > right){
int temp = arr[right];
arr[right] = arr[pivot];
arr[pivot] = temp;
}
else{
int temp = arr[right];
arr[right] = arr[left];
arr[left] = temp;
}
sort(arr, start, right - 1,true);
sort(arr, right + 1, end, true);
}
}
}
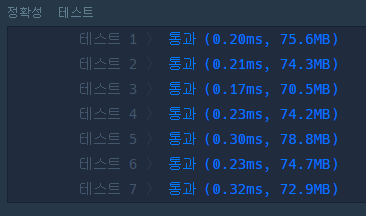