readdir.js
var testFolder = './data';
var fs = require('fs');
fs.readdir(testFolder, function(err, filelist){
console.log(filelist);
})
data 폴더에 있는 세 개의 파일 목록을 보여준다. Node.js는 파일 목록을 배열의 형태로 전달한다는 것을 알 수 있다.
main.js에서 다음 사진에 해당하는 부분을 Node.js가 파일 목록으로 변경할 수 있도록 수정하자.
main.js
var http = require('http');
var fs = require('fs');
var url = require('url');
var app = http.createServer(function(request,response){
var _url = request.url;
var queryData = url.parse(_url, true).query;
var pathname = url.parse(_url, true).pathname;
if(pathname === '/'){
if(queryData.id === undefined){
fs.readdir('./data', function(error, filelist){
var title = 'Welcome';
var description = 'Hello, Node.js';
var list = '<ul>';
var i = 0;
while(i < filelist.length){
list = list + `<li><a href="/?id=${filelist[i]}">${filelist[i]}</a></li>`;
i = i + 1;
}
list = list+'</ul>';
var template = `
<!doctype html>
<html>
<head>
<title>WEB1 - ${title}</title>
<meta charset="utf-8">
</head>
<body>
<h1><a href="/">WEB</a></h1>
${list}
<h2>${title}</h2>
<p>${description}</p>
</body>
</html>
`;
response.writeHead(200);
response.end(template);
})
} else {
fs.readdir('./data', function(error, filelist){
var title = 'Welcome';
var description = 'Hello, Node.js';
var list = '<ul>';
var i = 0;
while(i < filelist.length){
list = list + `<li><a href="/?id=${filelist[i]}">${filelist[i]}</a></li>`;
i = i + 1;
}
list = list+'</ul>';
fs.readFile(`data/${queryData.id}`, 'utf8', function(err, description){
var title = queryData.id;
var template = `
<!doctype html>
<html>
<head>
<title>WEB1 - ${title}</title>
<meta charset="utf-8">
</head>
<body>
<h1><a href="/">WEB</a></h1>
${list}
<h2>${title}</h2>
<p>${description}</p>
</body>
</html>
`;
response.writeHead(200);
response.end(template);
});
});
}
} else {
response.writeHead(404);
response.end('Not found');
}
});
app.listen(3000);
main.js에서 파일 리스트를 직접 입력해주었던 부분을 Node.js가 출력할 수 있도록 변경했다.
이제 파일이 추가되거나 수정되면 따로 코드를 수정할 필요가 없다. Node.js가 알아서 수정된 사항을 반영하여 동작한다!
! 함수 생성
function 함수 이름() {실행하고 싶은 코드}
! 함수 호출
함수 이름();
function.js
f123();
console.log('A');
console.log('Z');
console.log('B');
f123();
console.log('F');
console.log('C');
console.log('P');
console.log('J');
f123();
console.log('U');
console.log('A');
console.log('Z');
console.log('J');
console.log('I');
f123();
function f123(){
console.log(1);
console.log(2);
console.log(3);
console.log(4);
}
f123()이라는 함수를 생성하였다. f123(); 이라는 코드 한 줄로 함수를 호출할 수 있으며, 함수를 정의하는 코드에서 함수를 한 번에 수정할 수 있다.
function2.js
console.log(Math.round(1.6)); //round 함수는 반올림을 해주는 함수이다.
console.log(Math.round(1.4)); //입력값에 따라 값을 출력해준다.
function sum(first, second){
console.log(first+second);
}
sum(2,4); //2와 4를 더해준다.
parameter(매개변수)인 first와 second는 argument(위 코드에서 2와 4)를 입력 받아서 함수에 전달해주는 매개체이다.
내장함수를 출력하기 위해서는 console.log()를 이용해야하지만 직접 만든 함수는 sum(2,4);라고만 코딩해도 결과가 출력된다. 그러나 내장함수는 그 결과를 파일로, 이메일 등 여러 형태로 출력할 수 있다. 즉, 함수의 출력을 전달 받아서 다양한 용도로 사용이 가능하다. 우리가 직접 만든 함수도 광범위하게 사용할 수 있도록 해보자.
function2.js
return을 활용해서 구현했다. return을 만나면 함수는 그 즉시 실행이 종료된다. 즉, return은 '함수의 값을 출력한다'라는 의미와 '함수를 종료시킨다'라는 두 가지 의미를 지닌다.
console.log(Math.round(1.6)); //round 함수는 반올림을 해주는 함수이다. console.log(Math.round(1.4)); //입력값에 따라 값을 출력해준다.
function sum(first, second){
return first+second;
}
sum(2,4); //2와 4를 더해준다.
#### function2.js 실행 결과
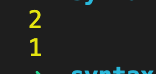
### 함수 이용해서 main.js 코드 정리하기
- 중복되는 코드를 함수를 이용해 정리했다.
> 매개변수를 입력하여 함수를 생성하고 함수를 이용해 코드 양을 줄이고 가독성 있는 코드로 수정했다.
main.js
``` javascript
var http = require('http');
var fs = require('fs');
var url = require('url');
function templateHTML(title, list, body){
return `
<!doctype html>
<html>
<head>
<title>WEB1 - ${title}</title>
<meta charset="utf-8">
</head>
<body>
<h1><a href="/">WEB</a></h1>
${list}
${body}
</body>
</html>
`;
}
function templateList(filelist){
var list = '<ul>';
var i = 0;
while(i < filelist.length){
list = list + `<li><a href="/?id=${filelist[i]}">${filelist[i]}</a></li>`;
i = i + 1;
}
list = list+'</ul>';
return list;
}
var app = http.createServer(function(request,response){
var _url = request.url;
var queryData = url.parse(_url, true).query;
var pathname = url.parse(_url, true).pathname;
if(pathname === '/'){
if(queryData.id === undefined){
fs.readdir('./data', function(error, filelist){
var title = 'Welcome';
var description = 'Hello, Node.js';
var list = templateList(filelist);
var template = templateHTML(title, list, `<h2>${title}</h2>${description}`);
response.writeHead(200);
response.end(template);
})
} else {
fs.readdir('./data', function(error, filelist){
fs.readFile(`data/${queryData.id}`, 'utf8', function(err, description){
var title = queryData.id;
var list = templateList(filelist);
var template = templateHTML(title, list, `<h2>${title}</h2>${description}`);
response.writeHead(200);
response.end(template);
});
});
}
} else {
response.writeHead(404);
response.end('Not found');
}
});
app.listen(3000);
실행 결과는 동일하다. 수정 전과 똑같이 작동하지만 코드의 효율성을 높일 수 있다.