Process Alternative - Threads
Threads
- Thread is a path of execution within a process. A process can contain multiple threads.
Motivation for Threads
- Process is expensive.
- Process creation in fork() model: Duplicate memory space & process context
- Context Switch: requires saving / restoration of process information
- It is harder for independent processes to communicate with each other (requires IPC) and independent memory space.
Advantages of Threads over Processes
- Responsiveness: If process is divided into multiple threads, if one thread finishes execution, its result can be immediately returned.
- Faster context switch: Process context switch requires more overhead from CPU than threads.
- Resource sharing: resources like code, data, and files can be shared among all threads in a process.
- Effective utilization of multiprocessor system:
- Parallelism: threads allow different parts of a program to be executed simultaneously on different processor cores.
Basic Idea:
- Traditional process has a single thread of control
- Only one instruction of whole program is executing at any time.
- Add more threads of control to the same process:
- Multiple parts of the program executing at the same time.
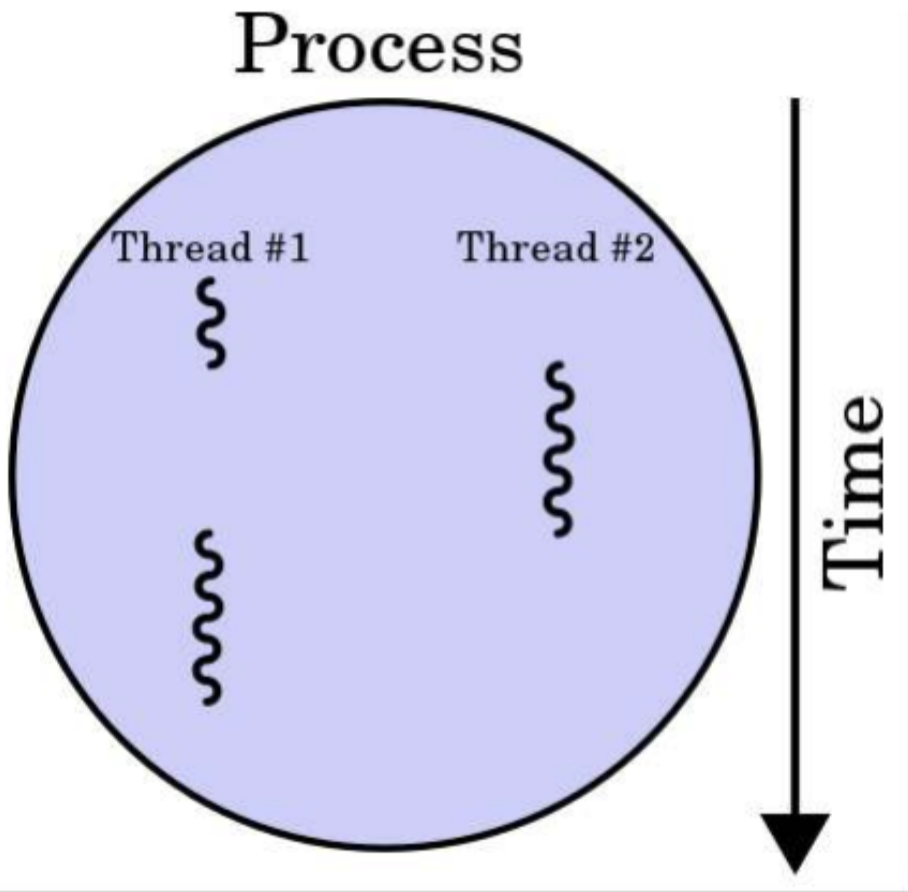
Multithreading Process (aka Light Weight Process)
- Achieve parallelism by dividing a process into multiple threads.
- Browser multiple tabs
- MS word uses a thread to format text and another to input text, and so one.
- Share:
- memory context (text, data, heap)
- OS context: Process id, other resources like files
- Each thread needs a:
- Identification (Thread ID)
- Register (General Purpose and Special)
- Stack
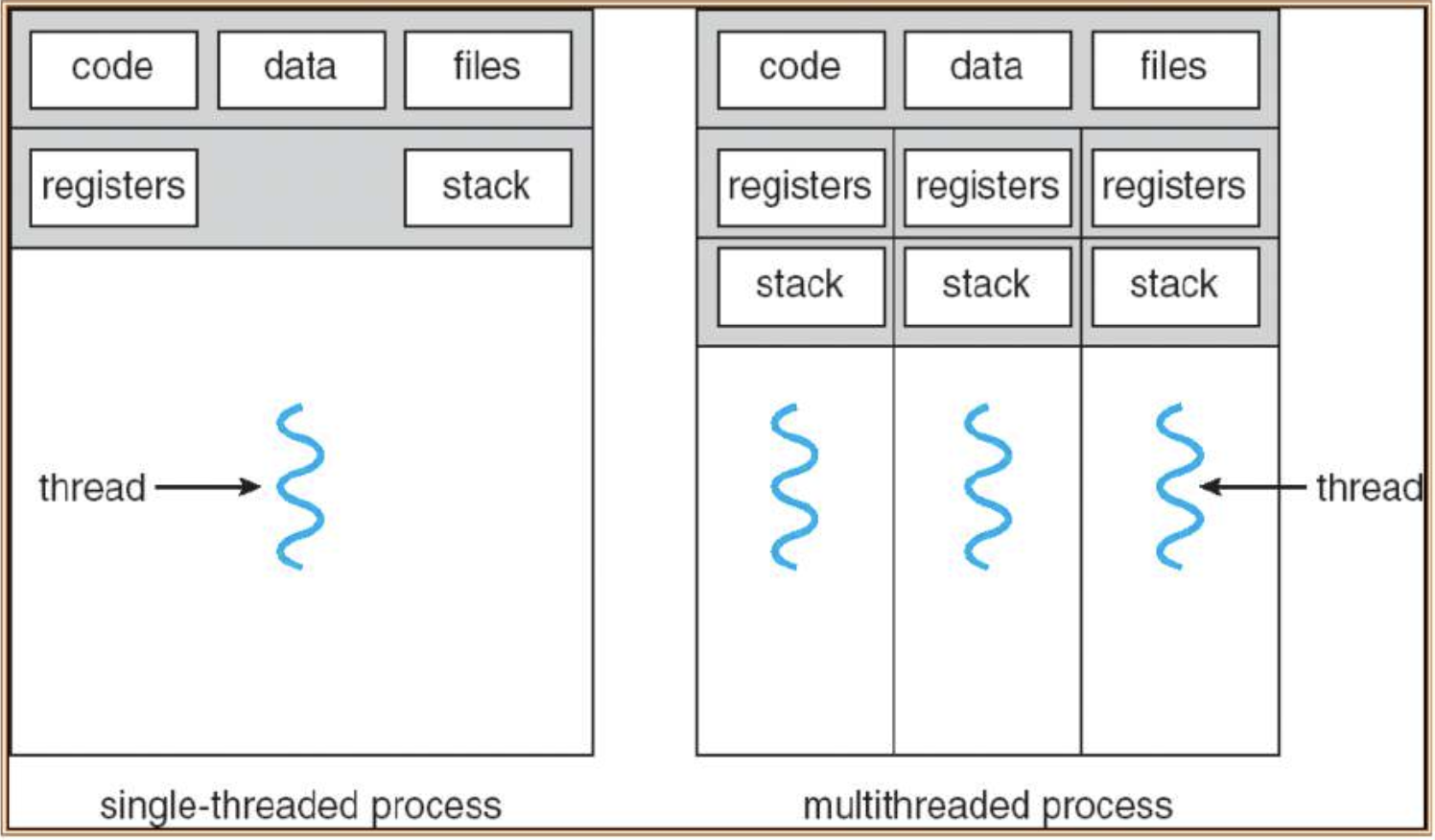
Process Context Switch vs Thread Context Switch
- Process Context Switch involves:
- OS context
- Hardware context
- Memory Context
- Thread context switch involves:
- Hardware context
- Registers, stacks (SP, FP)?
- Thread is much lighter than process
Threads: Problems:
- System call concurrency:
- Parallel execution of multiple threads -> parallel system call is possible -> race condition!
- Process behavior
- Impact on process operations: fork(), exit(), and exec() (I DONT GET IT)
Thread Models:
User Thread
- Thread is implemented as a user library.
- Runtime system (in the process) will handle thread-related tasks
- Kernel is not aware of the existence of the threads in the process.
Advantages:
- Can be implemented with language-specific libraries - higher portability and compatibility.
- Generally more configurable and flexible.
Disadvantages:
- OS is not aware of threads, scheduling is performed at process level
- This can affect responsiveness of other threads.
- Cannot exploit multiple CPUs.
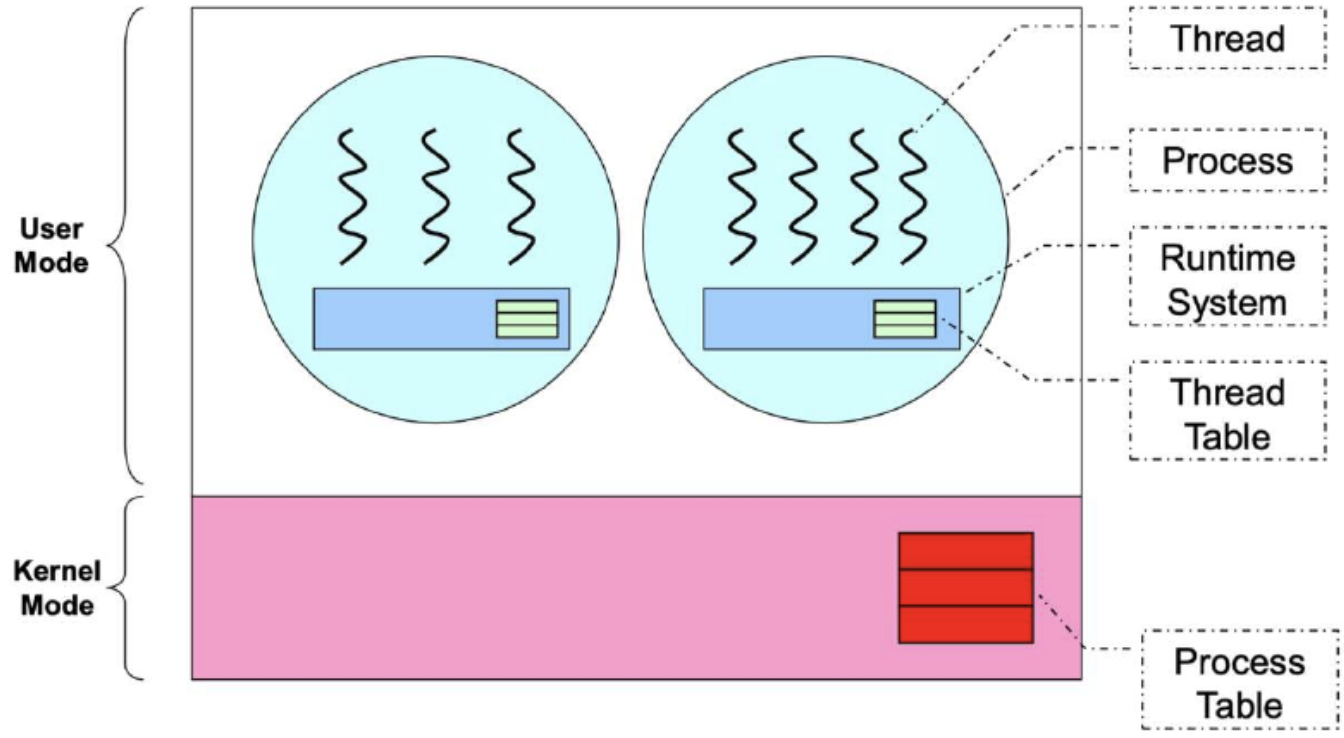
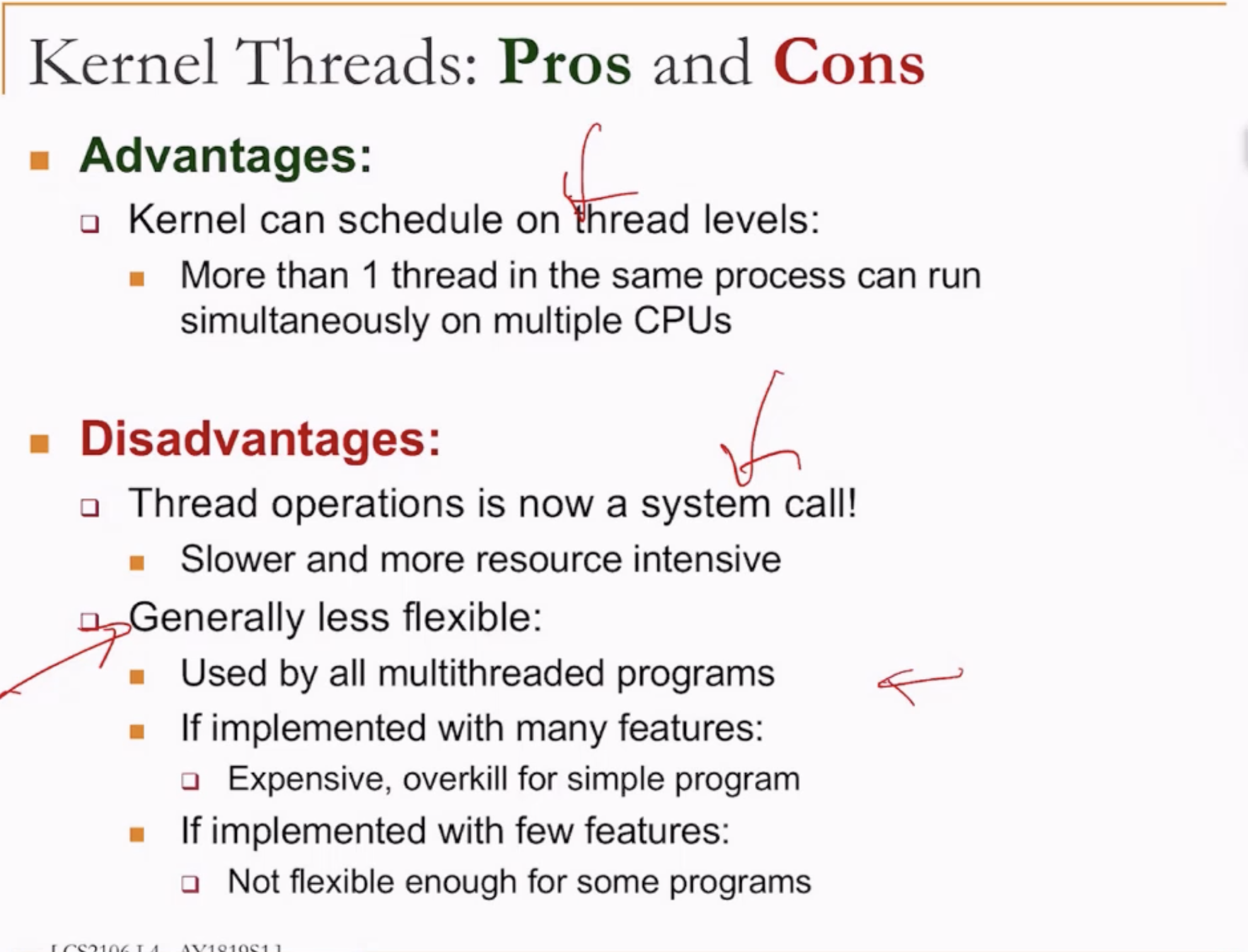
QS:
1. What is multithreading?
2. How is it different from forking and creating lots of processes?
3. Why does each thread require their own set of registers?
4. Why is IPC impossible in forking?
5. Name 3 types of contexts, and how context switching is like in single thread forking and multithread.
6. Why does context switching involve saving and restoring OS context like the file pointers?
7. Why is stack a hardware context?
8. What are SP and FP for?
9. System call concurrency?
10. What happens to the process when a thread calls an exit?
11. Why is the whole process blocked when just one thread is blocked?
12. What about the CPU?
13. Why is switching to kernel mode expensive?
14. What is kernel thread?
15. What are advantages and disadvantages of kernel threads?
16. Why is using kernel threads generally less flexible?
17. What is hybrid thread model?