console.log(1 == 1) // true console.log("1" == "1") // true console.log('1' == '2') // false console.log('1' == 1 ) // true
console.log(null == undefined) // true console.log(null === undefined) // false
console.log(ture == 1) //true console.log(false == 0) // true
console.log(true == '1') //true console.log(true ==='1') //false
console.log(1 != 2) // true console.log(1 != 1) // false
-> JS에서는 데이터를 이런식으로도 처리가 가능하다.
<예제>
document.write("<h1>출력할 내용</h1>");
<script> var myage = 19; if( myage == 19){ document.write("<h1>19살 입니다.</h1>"); } if( myage != 20){ document.write("<h1>20살이 아닙니다.</h1>"); } </script>
1)
<script> var point = 75; if( point > 70 && point <= 80 ){ document.write("<h1>C학점 입니다.</h1>") } if( point <= 70 || point > 80 ){ document.write("<h1>범위를 벗어났습니다.</h1>") } </script>
2)
<script> var is_korean = true; if( is_korean ){ document.write("<h1>한국사람입니다.</h1>") } if( is_korean == false ){ document.write("<h1>한국사람이 아닙니다.</h1>") } </script>
3)
<script> var is_korean = true; if( is_korean ){ document.write("<h1>한국사람입니다.</h1>") } if( is_korean == false ){ document.write("<h1>한국사람이 아닙니다.</h1>") } </script>
4)
<script> var myage = 19; if( myage > 19 ){ document.write("<h1>성인입니다.</h1>") } else { document.write("<h1>성인이 아닙니다.</h1>") } </script>
5)
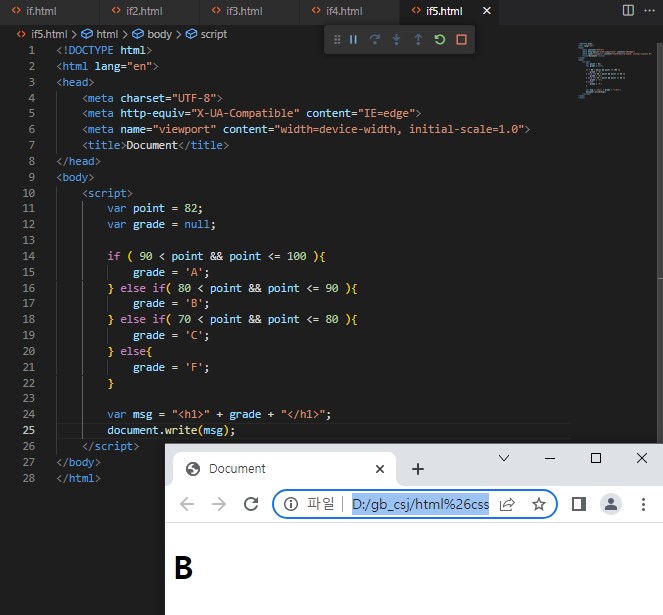<script> var point = 82; var grade = null; if ( 90 < point && point <= 100 ){ grade = 'A'; } else if( 80 < point && point <= 90 ){ grade = 'B'; } else if( 70 < point && point <= 80 ){ grade = 'C'; } else{ grade = 'F'; } var msg = "<h1>" + grade + "</h1>"; document.write(msg);
6)
<script> var grade = 'B'; switch(grade){ case 'A': document.write("<h1>91점~100점 사이</h1>"); break; case 'B': document.write("<h1>81점~90점 사이</h1>"); break; case 'C': document.write("<h1>71점~80점 사이</h1>"); break; default: document.write("<h1>70점 이하</h1>"); break; } </script>
7)
<script> var id = prompt(" 아이디를 입력해 주세요."); if( id == 'user' || id == 'admin' ){ alert('아이디가 일치합니다.'); } else{ alert('아이디가 일치하지 않습니다.'); } </script>
<실습>
<조건에 맞을 때>
<조건에 맞지 않을 때>
var age = 26; var beverage = (age > 21) ? "Beer" : "Juice" ;
🔎condition : 조건문으로 들어갈 표현식
🔎expTrue : 참일 때 실행할 식
🔎expFalse : 거짓일 때 실행할 식
1) for문
i)
ii)
2) while문
i)
ii)
<실습>
1) 1~100까지 중에 짝수의 합과 홀수의 합을 각각 구하여서 document.write로 출력하라
<script> var odd = 0; var even = 0; for( var i = 1; i <= 100; i++ ){ if(i % 2 == 0){ odd += i; } if(i % 2 == 1){ even += i; } } document.write("짝수의 합 : " + even + '<br/>' ); document.write("홀수의 합 : " + odd ); </script>
2) 구구단 2단 ~ 9단까지 출력하라
단
<h1>2단</h1>
결과값
<ul> <li>2 x 1 = 2</li> <li>2 x 2 = 4</li> ... </ul>
<script> for( var i = 2; i < 10; i++){ document.write('<h1>' + '<ul>' + i + '단' + '</ul>' + '</h1>'); for( var j = 1; j < 10; j++){ document.write('<li>' + i + " * " + j + " = " + i * j +'<br/>' + '</li>'); } } </script>
3) 다음과 같은 결과물을 출력하라.
<script> document.write("<table width='80%' border='1'"); for( var i = 0; i < 6; i++){ // 행 document.write("<tr>"); for( var j = 0; j < 7; j++){ var txt = "(" + i + " , " + j + " ) "; document.write("<td>" + txt + "</td>"); } document.write("</tr>"); } document.write("</table"); </script>