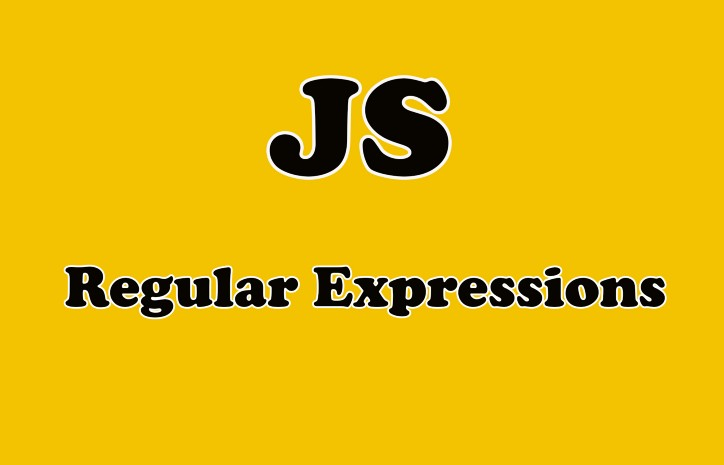
정규 표현식
- 정규 표현식(
regexp
또는 reg
라고 줄여서 사용)은 패턴(pattern)과 선택적으로 사용할 수 있는 플래그(flag)로 구성된다.
- 정규식 객체를 만들 땐 아래와 같이 두 가지 문법이 존재한다.
const regexp1 = new RegExp("pattern", "flags");
const regexp2 = /pattern/;
const regexp3 = /pattern/gmi;
- 슬래시
"/"
는 자바스크립트에게 정규 표현식을 생성하고 있다는 것을 알려준다. 문자열에 따옴표를 쓰는 것과 동일한 역할을 한다.
- 어느 문법을 사용하던 상관 없이 위 예시에서의
regexp
는 내장 클래스 RegExp
의 객체가 된다.
- 두 문법의 중요한 차이점은 짧은 문법
/.../
를 사용하면 문자열 템플릿 리터럴에서 ${...}
를 사용했던 것처럼 중간에 표현식을 넣을 수 없다는 점이다. 즉, 완전히 정적인 표현 방법이다.
- 짧은 문법은 코드를 작성하는 시점에 패턴을 알고 있을 때 유용하다. 대다수가 이런 경우에 속한다. 반면 긴 문법은 상황에 따라 동적으로 생성된 문자열을 가지고 정규 표현식을 만들어야 할 때 주로 사용한다. 관련 예시를 살펴보자.
let tag = prompt("어떤 태그를 찾고 싶나요?", "h2");
let regexp1 = new RegExp(`<${tag}>`);
let regexp2 = new RegExp(`{${'변수명'},${'변수명'}}`);
플래그
- 플래그는 정규 표현식의 옵션으로 검색하려는 문자 패턴에 추가적인 옵션을 넣어 원하는 문자 검색 결과를 반환한다.
- 플래그는 동시에 여러개를 사용할 수 있다.
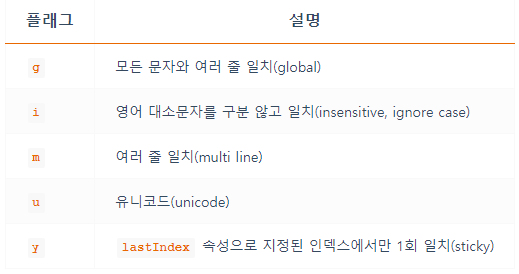
const str = `Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.`;
str.match(/ipsum/);
str.match(/ipsum/i);
str.match(/ipsum/ig);
const strMultyLine = `Lorem Ipsum is simply dummy text of the printing and typesetting industry.
Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book.
It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged.
It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.`;
strMultyLine.match(/$/g);
strMultyLine.match(/$/gm);
패턴
- 표현식의 다양한 특수기호(패턴)는 그 기호의 의미(기능)와 매칭되어 인식되지 않기 때문에 따로 외우지 않으면 의미를 파악할 수가 없다.
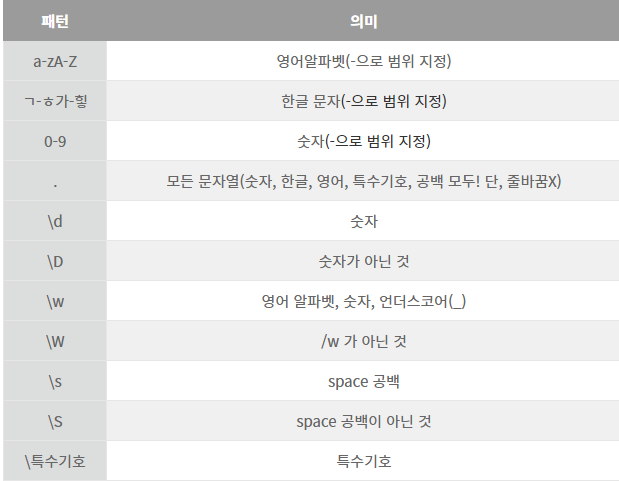
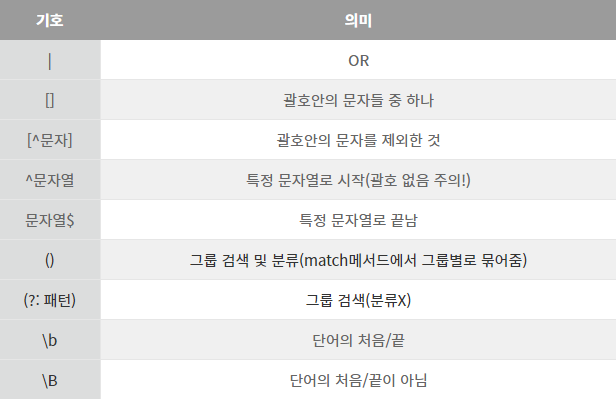
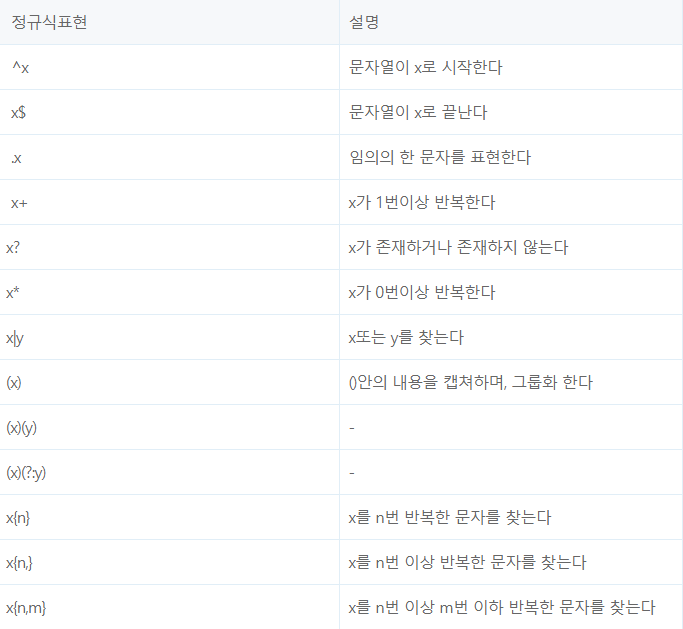
메소드
- 정규표현식에는 다양한 메소드들이 존재한다.
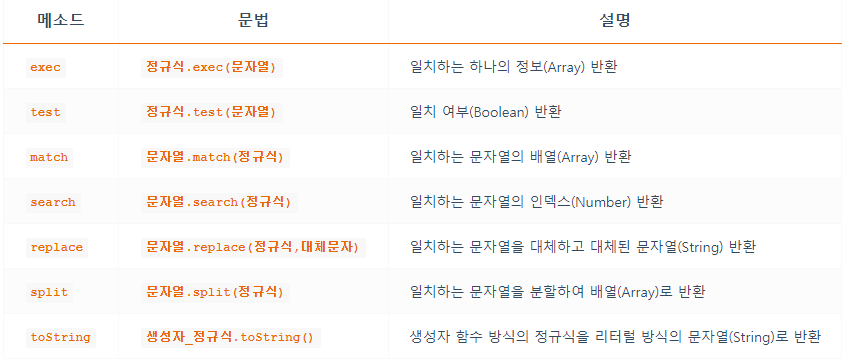
const str = `Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.`;
console.log(/ipsum/.exec(str));
console.log(/ipsum/i.exec(str));
console.log(/ipsum/i.test(str));
console.log(str.match(/ipsum/));
console.log(str.match(/ipsum/i));
console.log(str.search(/ipsum/i));
console.log(str.replace(/ipsum/i, 'Hoon'));
console.log(str.split(/ipsum/i));
참고자료