프로그래머스
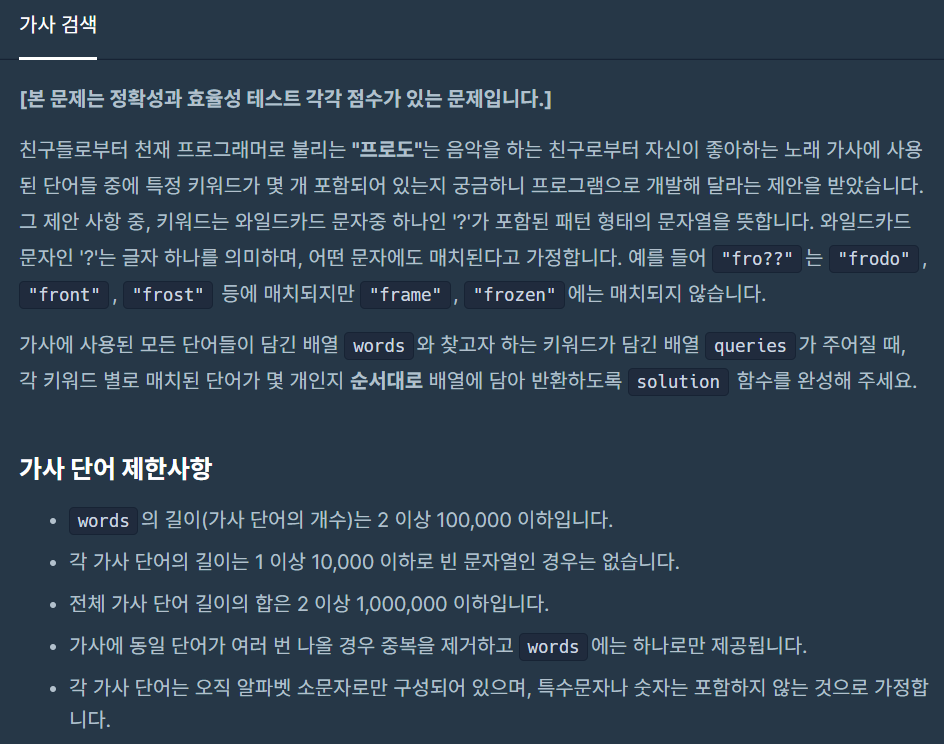
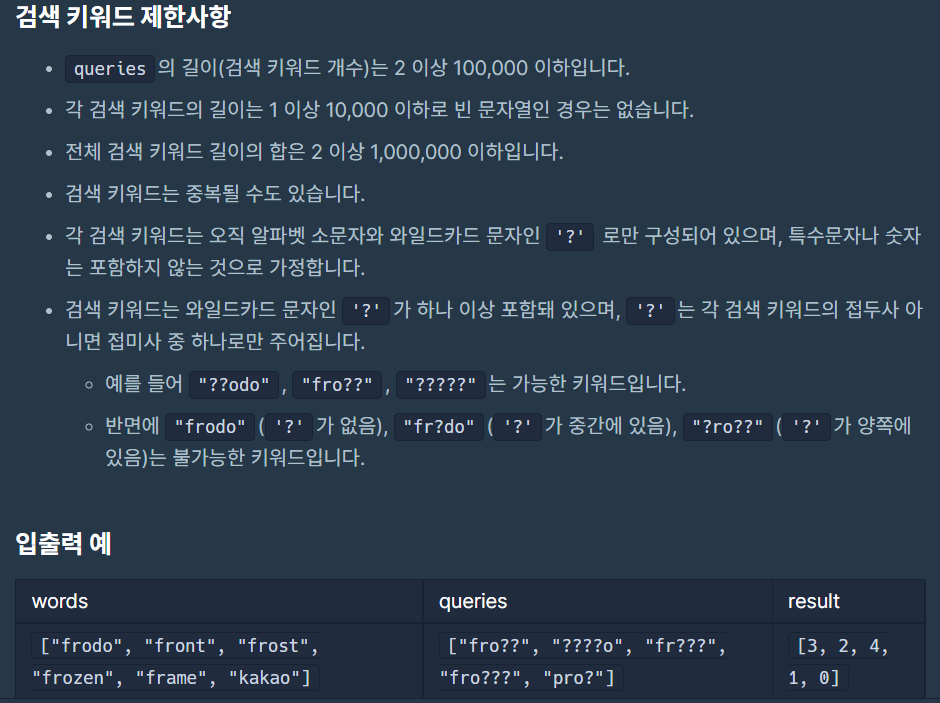
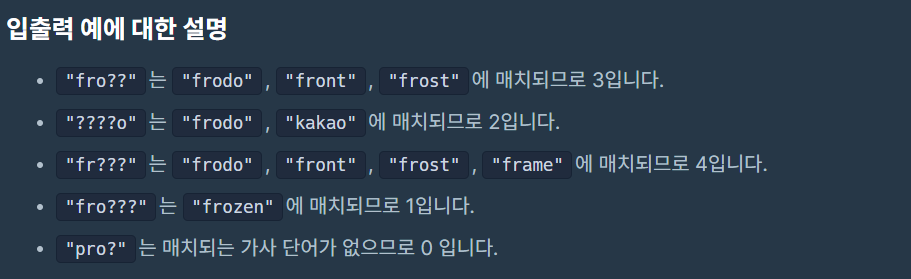
1. 이진 탐색
from bisect import bisect_left, bisect_right
def count_by_range(a, left_value, right_value):
right_index = bisect_right(a, right_value)
left_index = bisect_left(a, left_value)
return right_index - left_index
array = [[] for _ in range(10001)]
reversed_array = [[] for _ in range(10001)]
def solution(words, queries):
answer = []
for word in words:
array[len(word)].append(word)
reversed_array[len(word)].append(word[::-1])
for i in range(10001):
array[i].sort()
reversed_array[i].sort()
for q in queries:
if q[0] != '?':
res = count_by_range(array[len(q)], q.replace('?', 'a'), q.replace('?', 'z'))
else:
res = count_by_range(reversed_array[len(q)], q[::-1].replace('?','a'), q[::-1].replace('?', 'z'))
answer.append(res)
return answer
print(solution(["frodo", "front", "frost", "frozen", "frame", "kakao"], ["fro??", "????o", "fr???", "fro???", "pro?"]))
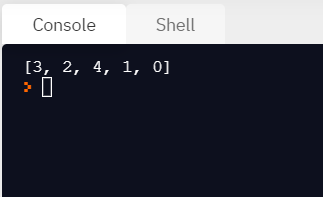
2. C++
#include <bits/stdc++.h>
using namespace std;
int countByRange(vector<string>& v, string leftValue, string rightValue) {
vector<string>::iterator rightIndex = upper_bound(v.begin(), v.end(), rightValue);
vector<string>::iterator leftIndex = lower_bound(v.begin(), v.end(), leftValue);
return rightIndex - leftIndex;
}
string replaceAll(string str, string from, string to){
string res = str;
int pos = 0;
while((pos = res.find(from, pos)) != string::npos)
{
res.replace(pos, from.size(), to);
pos += to.size();
}
return res;
}
vector<string> arr[10001];
vector<string> reversed_arr[10001];
vector<int> solution(vector<string> words, vector<string> queries) {
vector<int> answer;
for (int i = 0; i < words.size(); i++) {
string word = words[i];
arr[word.size()].push_back(word);
reverse(word.begin(), word.end());
reversed_arr[word.size()].push_back(word);
}
for (int i = 0; i < 10001; i++) {
sort(arr[i].begin(), arr[i].end());
sort(reversed_arr[i].begin(), reversed_arr[i].end());
}
for (int i = 0; i < queries.size(); i++) {
string q = queries[i];
int res = 0;
if (q[0] != '?') {
res = countByRange(arr[q.size()], replaceAll(q, "?", "a"), replaceAll(q, "?", "z"));
}
else {
reverse(q.begin(), q.end());
res = countByRange(reversed_arr[q.size()], replaceAll(q, "?", "a"), replaceAll(q, "?", "z"));
}
answer.push_back(res);
}
return answer;
}