백준
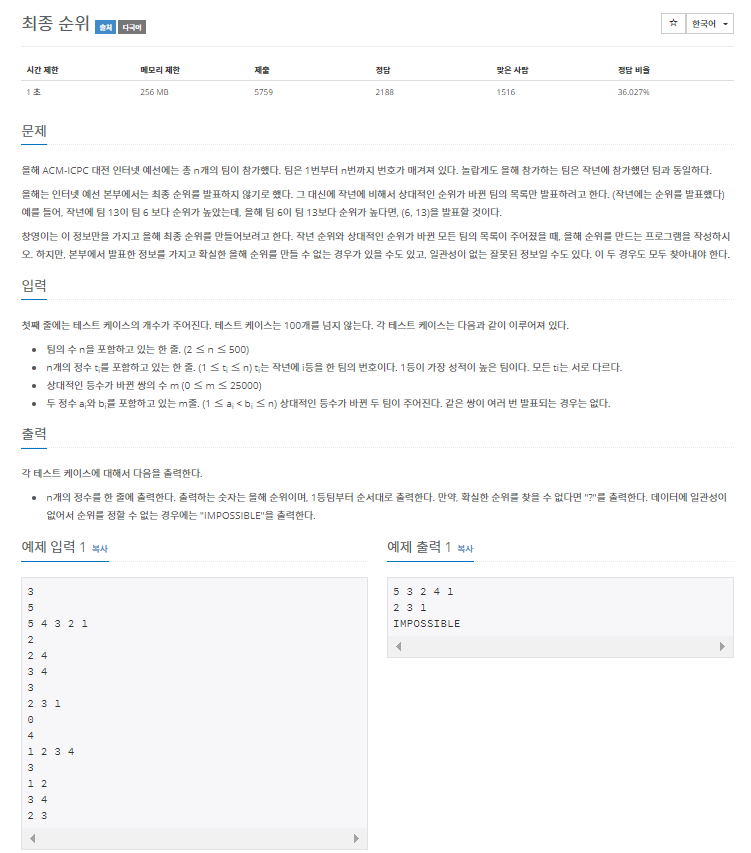
1. 위상 정렬
from collections import deque
for t in range(int(input())):
n = int(input())
indegree = [0] * (n + 1)
graph = [[False] * (n + 1) for i in range(n + 1)]
data = list(map(int, input().split())
)
for i in range(n):
for j in range(i + 1, n):
graph[data[i]][data[j]] = True
indegree[data[j]] += 1
m = int(input())
for i in range(m):
a, b = map(int, input().split())
if graph[a][b]:
graph[a][b] = False
graph[b][a] = True
indegree[a] += 1
indegree[b] -= 1
else:
graph[a][b] = True
graph[b][a] = False
indegree[a] -= 1
indegree[b] += 1
result = []
q = deque()
for i in range(1, n + 1):
if indegree[i] == 0:
q.append(i)
certain = True
cycle = False
for i in range(n):
if len(q) == 0:
cycle = True
break
if len(q) >= 2:
certain = False
break
now = q.popleft()
result.append(now)
for j in range(1, n + 1):
if graph[now][j]:
indegree[j] -= 1
if indegree[j] == 0:
q.append(j)
if cycle:
print("IMPOSSIBLE")
elif not certain:
print("?")
else:
for i in result:
print(i, end=' ')
print()
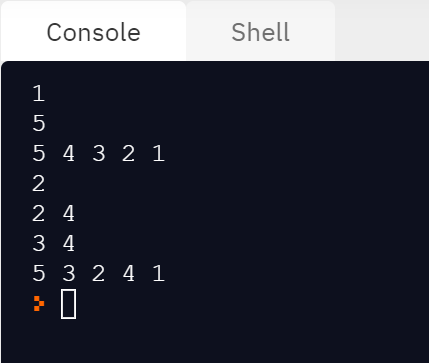
2. C++
#include <bits/stdc++.h>
using namespace std;
int testCase, n, m;
int indegree[501];
bool graph[501][501];
int main(void) {
cin >> testCase;
for (int tc = 0; tc < testCase; tc++) {
fill(indegree, indegree + 501, 0);
for (int i = 0; i < 501; i++) {
fill(graph[i], graph[i] + 501, false);
}
cin >> n;
vector<int> v;
for (int i = 0; i < n; i++) {
int x;
cin >> x;
v.push_back(x);
}
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
graph[v[i]][v[j]] = true;
indegree[v[j]] += 1;
}
}
cin >> m;
for (int i = 0; i < m; i++) {
int a, b;
cin >> a >> b;
if (graph[a][b]) {
graph[a][b] = false;
graph[b][a] = true;
indegree[a] += 1;
indegree[b] -= 1;
}
else {
graph[a][b] = true;
graph[b][a] = false;
indegree[a] -= 1;
indegree[b] += 1;
}
}
vector<int> result;
queue<int> q;
for (int i = 1; i <= n; i++) {
if (indegree[i] == 0) {
q.push(i);
}
}
bool certain = true;
bool cycle = false;
for (int i = 0; i < n; i++) {
if (q.size() == 0) {
cycle = true;
break;
}
if (q.size() >= 2) {
certain = false;
break;
}
int now = q.front();
q.pop();
result.push_back(now);
for (int j = 1; j <= n; j++) {
if (graph[now][j]) {
indegree[j] -= 1;
if (indegree[j] == 0) {
q.push(j);
}
}
}
}
if (cycle) cout << "IMPOSSIBLE" << '\n';
else if (!certain) cout << "?" << '\n';
else {
for (int i = 0; i < result.size(); i++) {
cout << result[i] << ' ';
}
cout << '\n';
}
}