C++ 프로그래밍
Class
#include<iostream>
#include<string.h>
#pragma warning(disable:4996)
using namespace std;
class Human
{
private:
char* name;
int id;
int age;
public:
Human(const char *aname, int aid, int aage)
{
name=new char[strlen(aname)+1];
strcpy(name, aname);
id = aid;
age = aage;
}
~Human()
{
delete[] name;
}
void getData()
{
cout << "이름:" << name << " 학번:" << id << " 나이:" << age << endl;
}
};
int main()
{
Human h("오윤범", 20172537, 26);
h.getData();
Human ary[3] = { Human("가나다",111,10) , Human("라마바",222,20), Human("사아자",333,30) };
ary[0].getData();
ary[1].getData();
ary[2].getData();
return 0;
}
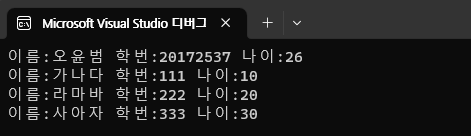
복사생성자
#include<iostream>
using namespace std;
class Myclass
{
int num;
public:
Myclass(int n) :num(n)
{
cout << "생성자 호출" << endl;
}
Myclass(Myclass& other)
{
cout << "복사생성자 호출" << endl;
num = other.num;
}
void getData()
{
cout << num << endl;
}
};
int main()
{
Myclass m1(10);
Myclass m2 = m1;
Myclass m3(m2);
m1.getData();
m2.getData();
m3.getData();
return 0;
}
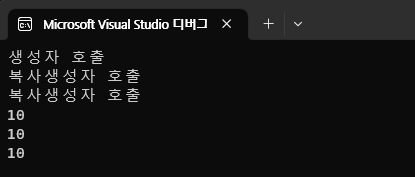
프로그래머스
짝수,홀수 개수
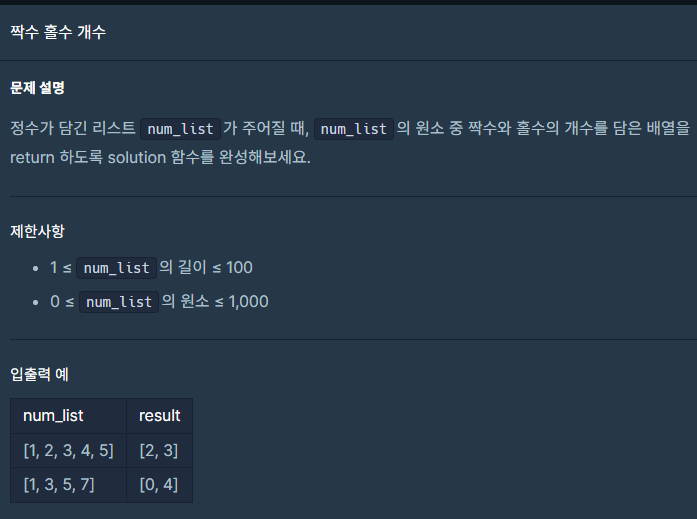
def solution(num_list):
even_cnt,odd_cnt=0,0
for i in range(len(num_list)):
if num_list[i]%2==0:
even_cnt+=1
else:
odd_cnt+=1
answer=[even_cnt,odd_cnt]
return answer
solution([1,2,3,4,5])
문자 반복 출력하기
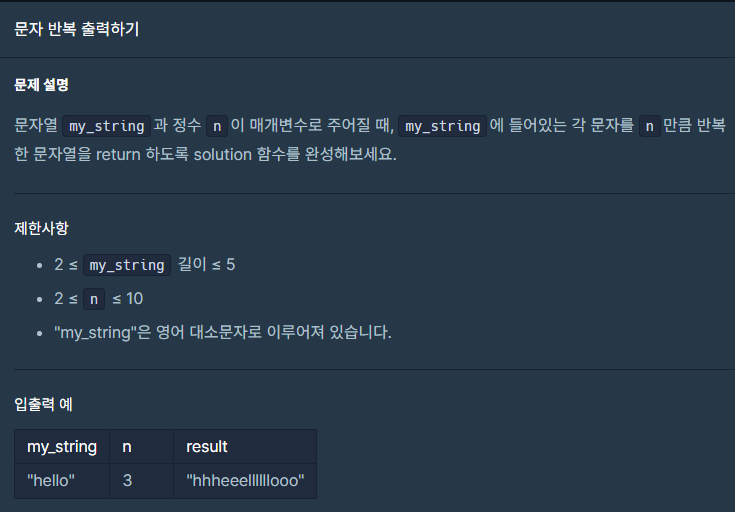
def solution(my_string, n):
answer=''
for i in range(len(my_string)):
answer+=my_string[i]*n
return answer
solution("hello",4)
출력)'hhhheeeelllllllloooo'