C# 코딩테스트
1번
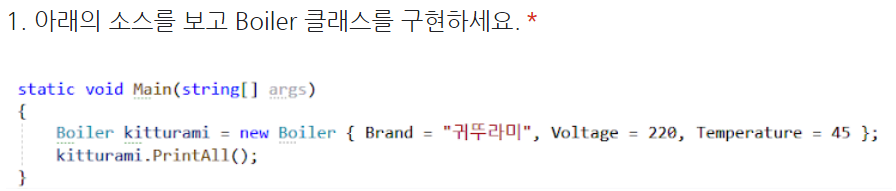
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace N1
{
public class Boiler
{
public string Brand { get; set; }
public byte Voltage { get; set; }
public int Temperature { get; set; }
public void PrintAll()
{
Console.WriteLine($"Brand : {Brand} Voltage : {Voltage} Temperature : {Temperature}");
}
}
internal class Program
{
static void Main(string[] args)
{
Boiler kitturami = new Boiler { Brand = "귀뚜라미", Voltage = 220, Temperature = 45 };
kitturami.PrintAll();
}
}
}
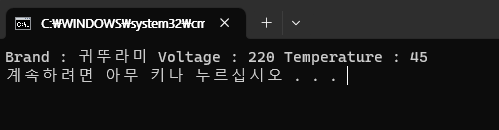
2번
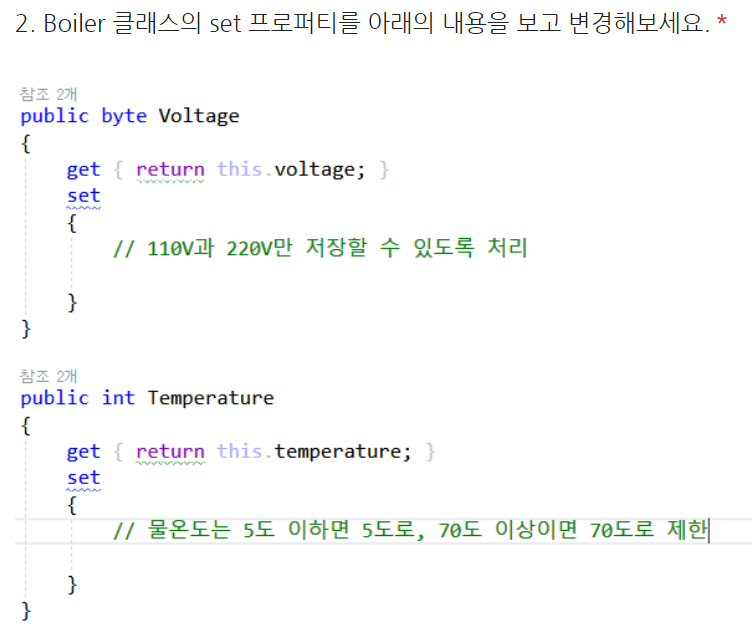
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace N2
{
public class Boiler
{
public string Brand { get; set; }
private byte voltage;
public byte Voltage
{
get { return voltage; }
set
{
if(value==110 || value==220)
{
voltage = value;
}
else
{
Console.WriteLine("Voltage에는 110/220만 입력");
}
}
}
private int temperature;
public int Temperature
{
get { return this.temperature; }
set
{
if (value <= 5)
{
this.temperature = 5;
}
else if (value >= 70)
{
this.temperature = 70;
}
else
{
this.temperature = value;
}
}
}
public void PrintAll()
{
Console.WriteLine($"Brand : {Brand} Voltage : {Voltage} Temperature : {Temperature}");
}
}
internal class Program
{
static void Main(string[] args)
{
Boiler kitturami = new Boiler { Brand = "귀뚜라미", Voltage = 220, Temperature = 45 };
kitturami.PrintAll();
Boiler kitturami1 = new Boiler { Brand = "귀뚜라미", Voltage = 20, Temperature = 3 };
kitturami1.PrintAll();
}
}
}
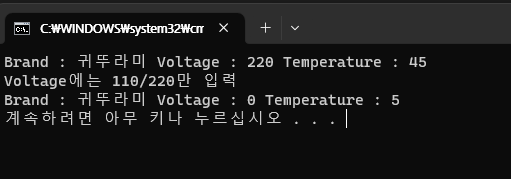
3번
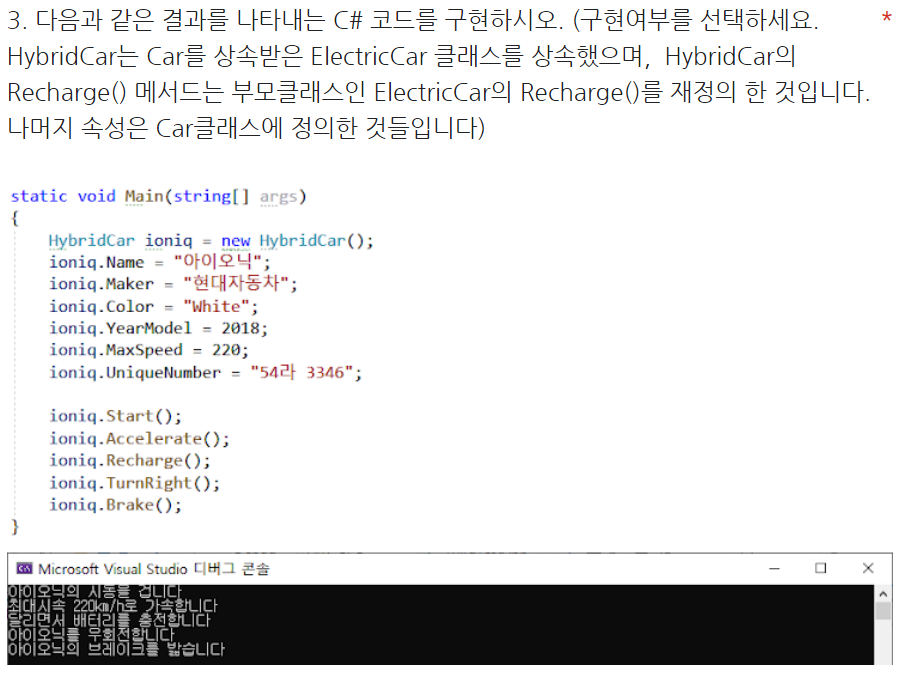
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography.X509Certificates;
using System.Text;
using System.Threading.Tasks;
namespace N3
{
class car
{
public string Name { get; set; }
public string Maker { get; set; }
public string Color { get; set; }
public int YearMode { get; set; }
public int MaxSpeed { get; set; }
public string UniqueNumber { get; set; }
public void Start()
{
Console.WriteLine($"{this.Name}의 시동을 겁니다");
}
public void Acclerate()
{
Console.WriteLine("절대시속 220km/h로 가속합니다");
}
public void TurnRight()
{
Console.WriteLine($"{this.Name}을 우회전합니다");
}
public void Break()
{
Console.WriteLine($"{this.Name}의 브레이크를 밟습니다");
}
}
class ElectricCar : car
{
public virtual void Recharge()
{
}
}
class HybridCar : ElectricCar
{
public override void Recharge()
{
base.Recharge();
Console.WriteLine("달리면서 배터리를 충전합니다");
}
}
internal class Program
{
static void Main(string[] args)
{
HybridCar ioniq = new HybridCar();
ioniq.Name = "아이오닉";
ioniq.Maker = "현대자동차";
ioniq.Color = "White";
ioniq.YearMode = 2018;
ioniq.MaxSpeed = 220;
ioniq.UniqueNumber = "54라 3346";
ioniq.Start();
ioniq.Acclerate();
ioniq.Recharge();
ioniq.TurnRight();
ioniq.Break();
}
}
}
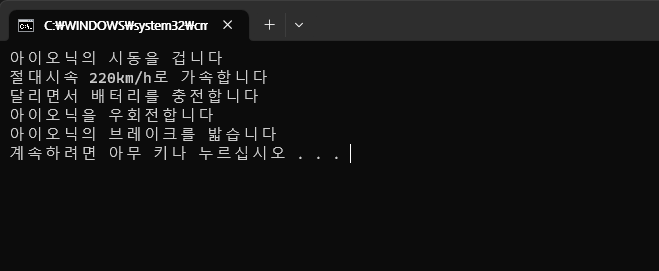
4번
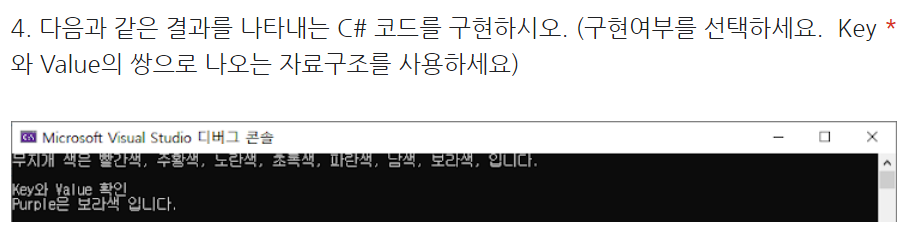
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace N4
{
internal class Program
{
static void Main(string[] args)
{
Dictionary<string, string> colors = new Dictionary<string, string>()
{
{"Red", "빨간색"},
{"Orange", "주황색"},
{"Yellow", "노란색"},
{"Green", "초록색"},
{"Blue", "파란색"},
{"Navy", "남색"},
{"Purple", "보라색"}
};
Console.Write("무지개 색은 ");
foreach (var v in colors.Values)
{
Console.Write(v+", ");
}
Console.WriteLine(" 입니다.");
Console.WriteLine();
Console.WriteLine("Key와 Value 확인");
string key = "Purple";
string value = colors[key];
Console.WriteLine($"{key}은 {value} 입니다.");
}
}
}
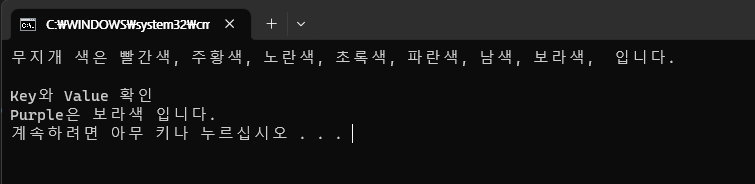
5번

using N5;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace N5
{
public interface IAnimal
{
int Age { get; set; }
String Name { get; set; }
void Eat();
void Sleep();
void Sound();
}
public class Dog : IAnimal
{
public string Name { get; set; }
public int Age { get; set; }
public void Eat()
{
Console.WriteLine($"{this.Name}이/가 먹습니다");
}
public void Sleep()
{
Console.WriteLine($"{this.Name}이/가 잡니다");
}
public void Sound()
{
Console.WriteLine($"{this.Name}이/가 짖습니다 멍멍");
}
}
public class Cat : IAnimal
{
public string Name { get; set; }
public int Age { get; set; }
public void Eat()
{
Console.WriteLine($"{this.Name}이/가 먹습니다");
}
public void Sleep()
{
Console.WriteLine($"{this.Name}이/가 잡니다");
}
public void Sound()
{
Console.WriteLine($"{this.Name}이/가 웁니다 야옹");
}
}
public class Horse : IAnimal
{
public string Name { get; set; }
public int Age { get; set; }
public void Eat()
{
Console.WriteLine($"{this.Name}이/가 먹습니다");
}
public void Sleep()
{
Console.WriteLine($"{this.Name}이/가 잡니다");
}
public void Sound()
{
Console.WriteLine($"{this.Name}이/가 웁니다 이히힝");
}
}
}
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("-- 강아지 객체 생성 --");
Dog dog = new Dog { Name = "우리집강아지", Age = 1 };
dog.Sleep();
dog.Eat();
dog.Sound();
Console.WriteLine("\n-- 고양이 객체 생성 --");
Cat cat = new Cat { Name = "우리집고양이", Age = 2 };
cat.Sleep();
cat.Eat();
cat.Sound();
Console.WriteLine("\n-- 말 객체 생성 --");
Horse horse = new Horse { Name = "우리집말", Age = 3 };
horse.Sleep();
horse.Eat();
horse.Sound();
}
}
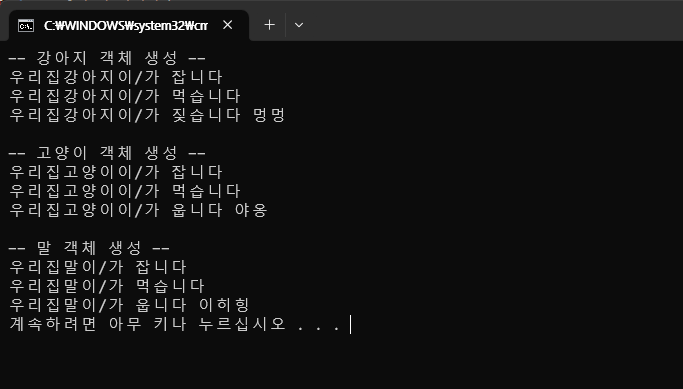