웹에서 JSON 데이터 받아오기
기본
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1 id="first">
</h1>
<script>
fetch("https://jsonplaceholder.typicode.com/posts/1")
.then((response) => {
console.log(response);
return response.json();
})
.then((result) => {
console.log(result);
document.querySelector("#first").innerHTML = result.title;
});
</script>
</body>
</html>
랜덤 적용
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button id="randomButton">랜덤</button>
<h1 id="first">
</h1>
<script>
const getData = () => {
const ranPostNum = Math.ceil(Math.random() * 100);
fetch(`https://jsonplaceholder.typicode.com/posts/${ranPostNum}`)
.then((response) => {
return response.json();
})
.then((result) => {
console.log(result);
document.querySelector("#first").innerHTML = "제목 : " + result.title;
console.log("fetch함수 내부")
});
console.log("fetch함수 이후")
}
document.querySelector("#randomButton").addEventListener("click", getData);
</script>
</body>
</html>
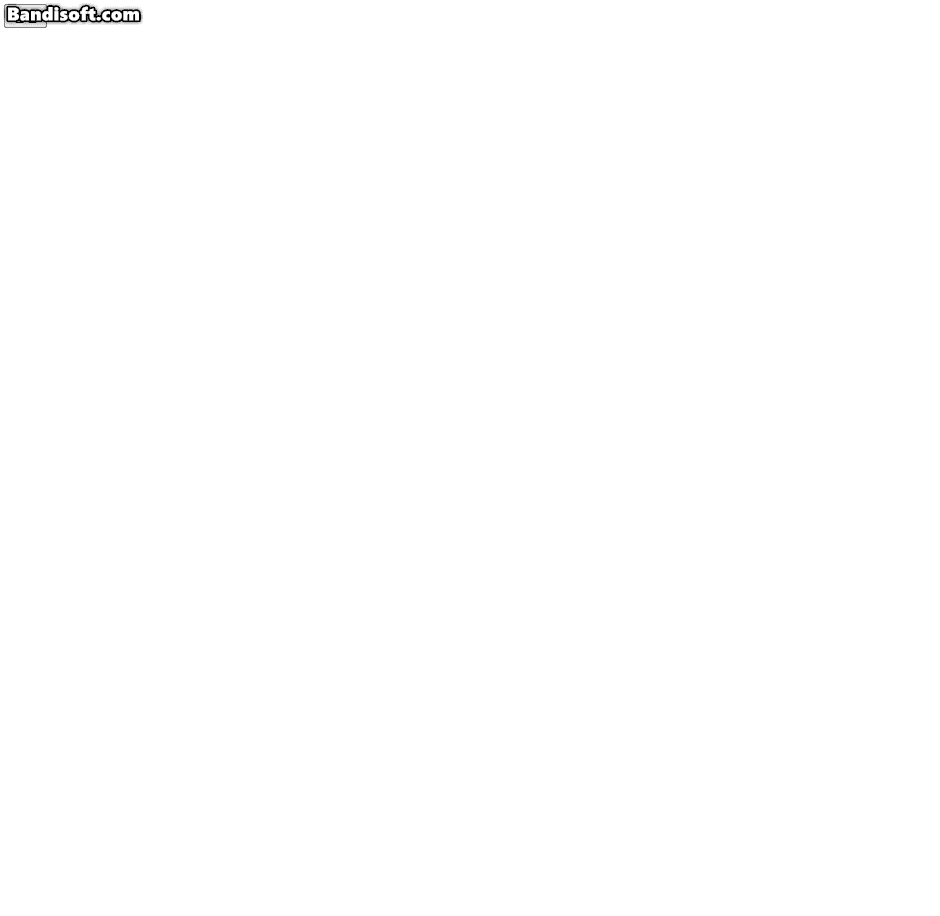
실습
.1
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul id="titleList">
</ul>
<script>
fetch("https://jsonplaceholder.typicode.com/posts")
.then((response) => response.json())
.then((result) => {
console.log(result);
for (const iterator of result) {
document.querySelector("#titleList").insertAdjacentHTML(
"beforeend",
`<li>${iterator.id}. ${iterator.title}</li>`
);
};
});
</script>
</body>
</html>
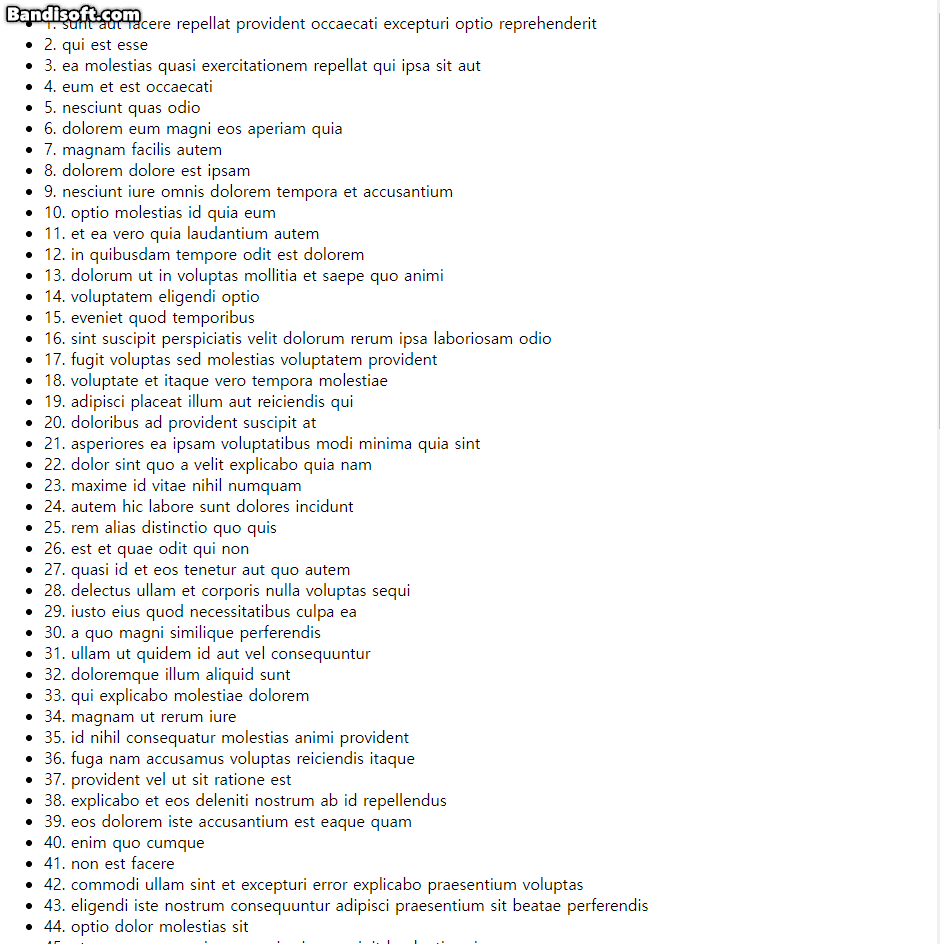
.2
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul id="movieRank">
</ul>
<script>
fetch("http://kobis.or.kr/kobisopenapi/webservice/rest/boxoffice/searchDailyBoxOfficeList.json?key=f5eef3421c602c6cb7ea224104795888&targetDt=20230707")
.then((response) => response.json())
.then((result) => {
console.log(result.boxOfficeResult.dailyBoxOfficeList);
for (const iterator of result.boxOfficeResult.dailyBoxOfficeList) {
document.querySelector("#movieRank").insertAdjacentHTML(
"beforeend",
`<li>${iterator.rank}위. ${iterator.movieNm}</li>`
);
};
})
</script>
</body>
</html>
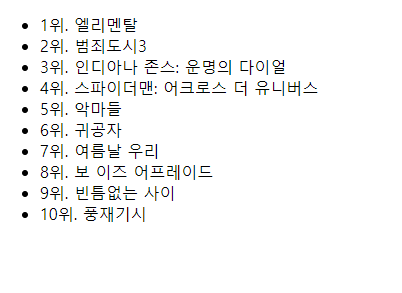