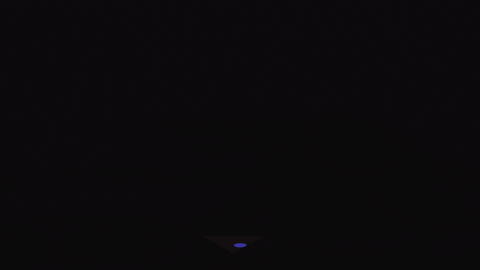
📝 짝지어 제거하기
문제 링크
📌 스택
- 스택 자료 구조를 사용하여 구현할 수 있는 문제입니다.
나의 풀이
function solution(s) {
const stack = [];
for(let i = 0; i < s.length; i++){
if(!stack.length || s[i] !== stack[stack.length - 1]) stack.push(s[i])
else stack.pop();
}
return stack.length ? 0 : 1;
}
- 스택의 마지막 요소와 문자열이 같은 지를 먼저 판별하고 싶으면 다음과 같이 쓸 수 있습니다.
function solution(s) {
const stack = [];
for(let i = 0; i < s.length; i++){
if(stack[stack.length - 1] === s[i]) stack.pop();
else stack.push(s[i]);
}
}
📝 프린터
문제 링크
나의 풀이
function solution(priorities, location) {
let count = 0;
while (true) {
let first = priorities.shift();
if (priorities.filter((el) => first < el).length) {
priorities.push(first);
} else {
count++;
if (location === 0) {
return count;
}
}
location--;
if (location === -1) {
location = priorities.length - 1;
}
}
return answer;
}
다른 풀이
function solution(priorities, location) {
var list = priorities.map((t,i)=>({
my : i === location,
val : t
}));
var count = 0;
while(true){
var cur = list.splice(0,1)[0];
if(list.some(t=> t.val > cur.val )){
list.push(cur);
}
else{
count++;
if(cur.my) return count;
}
}
}
function solution(priorities, location) {
var arr = priorities.map((priority, index) => {
return {
index: index, priority: priority
};
});
var queue = [];
while(arr.length > 0) {
var firstEle = arr.shift();
var hasHighPriority = arr.some(ele => ele.priority > firstEle.priority);
if (hasHighPriority) {
arr.push(firstEle);
} else {
queue.push(firstEle);
}
}
return queue.findIndex(queueEle => queueEle.index === location) + 1;
}
참고 자료
Array.prototype.some()