문제 1
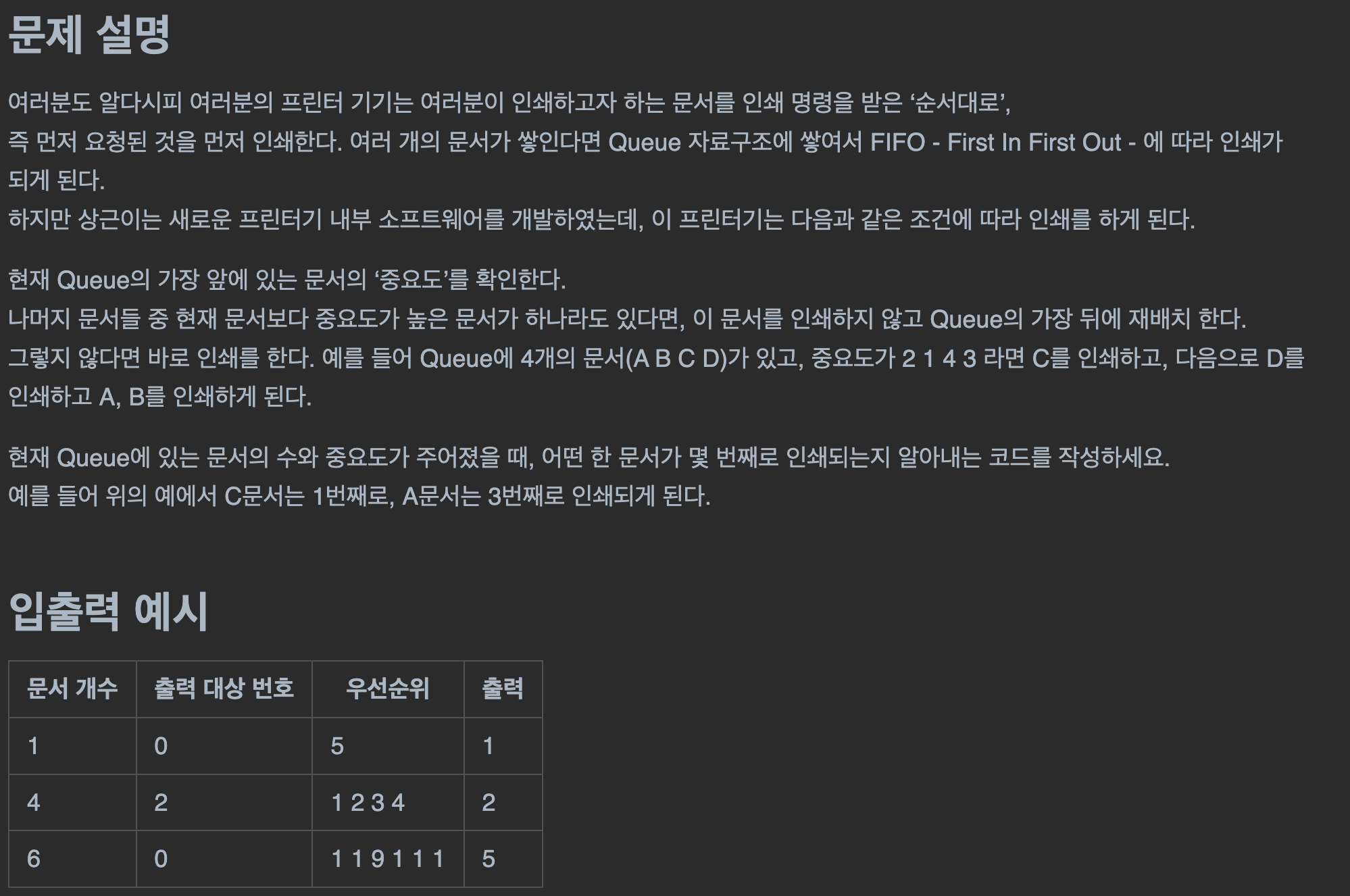
class Doc{
int no;
int priority;
public Doc(int no, int priority) {
this.no = no;
this.priority = priority;
}
}
public class Practice1 {
public static void solution(int docs, int target, int[] priority) {
Queue<Doc> queue = new LinkedList<>();
for(int i = 0; i < docs; i++){
queue.add(new Doc(i, priority[i]));
}
int cnt = 1;
while(true){
Doc cur = queue.poll();
Doc[] highP = queue.stream().filter(x -> x.priority > cur.priority).toArray(Doc[]::new);
if(highP.length > 0){
queue.offer(cur);
}else{
if(cur.no == target){
System.out.println(cnt);
break;
}
cnt++;
}
}
}
public static void main(String[] args) {
int docs = 1;
int target = 0;
int[] priority = {5};
solution(docs, target, priority);
docs = 4;
target = 2;
priority = new int[]{1, 2, 3, 4};
solution(docs, target, priority);
docs = 6;
target = 0;
priority = new int[]{1, 1, 9, 1, 1, 1};
solution(docs, target, priority);
}
}
문제 2
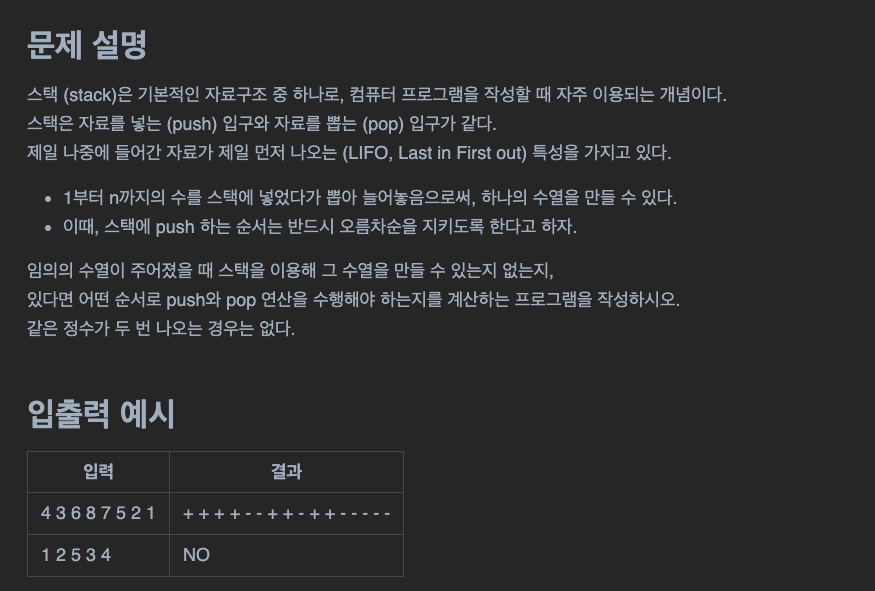
public class Practice2 {
public static void solution(int[] nums) {
Stack<Integer> stack = new Stack<>();
ArrayList<String> result = new ArrayList<>();
int idx = 0;
for (int i = 0; i < nums.length; i++) {
int num = nums[i];
if(num > idx){
for (int j = idx+1; j < num+1; j++) {
stack.push(j);
result.add("+");
}
idx = num;
}else if(stack.peek() != num){
System.out.println("NO");
return;
}
stack.pop();
result.add("-");
}
for(String item : result){
System.out.print(item);
}
}
public static void main(String[] args) {
int[] nums = {4, 3, 6, 8, 7, 5, 2, 1};
solution(nums);
System.out.println();
System.out.println("=====");
nums = new int[]{1, 2, 5, 3, 4};
solution(nums);
}
}
문제 3
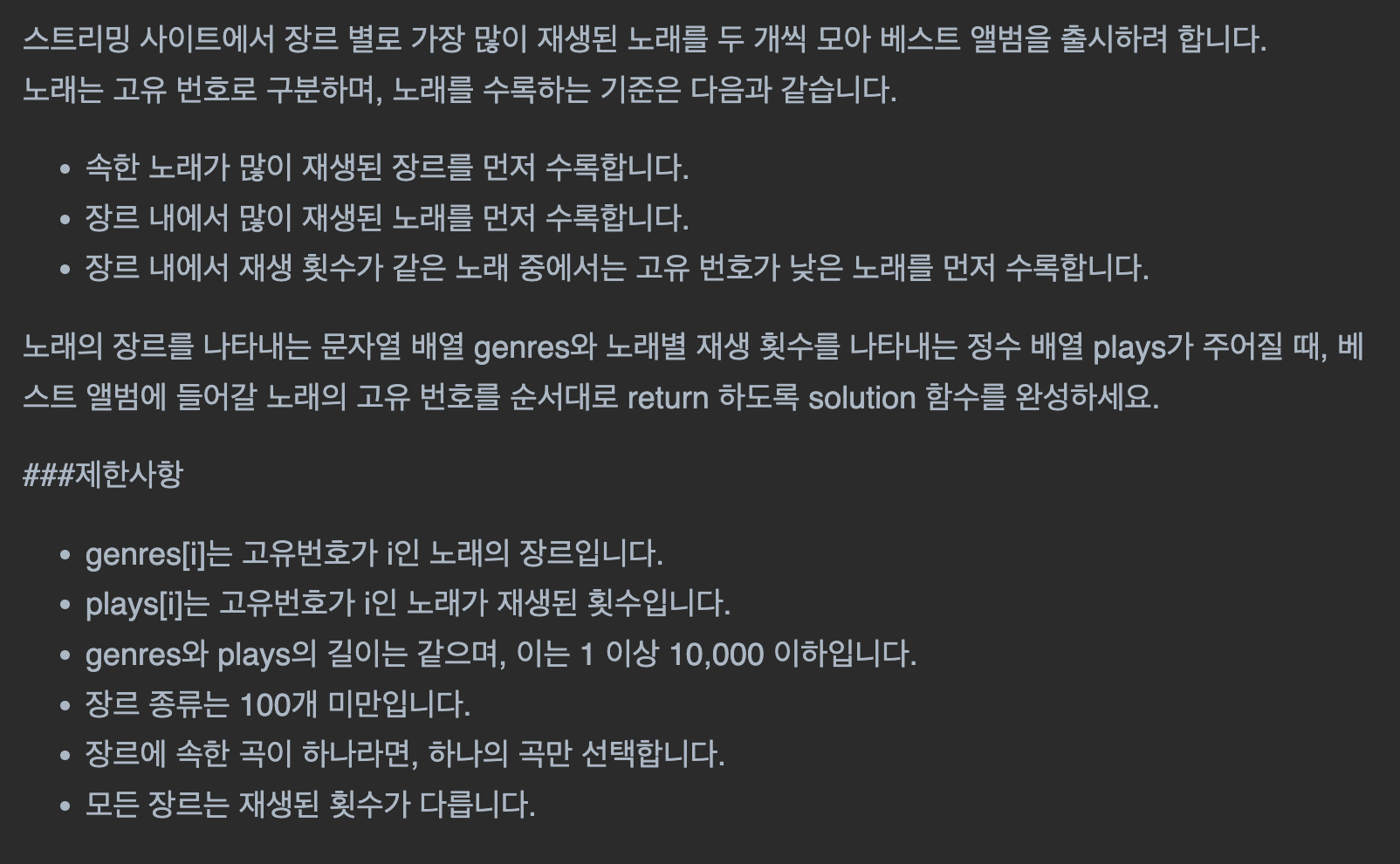
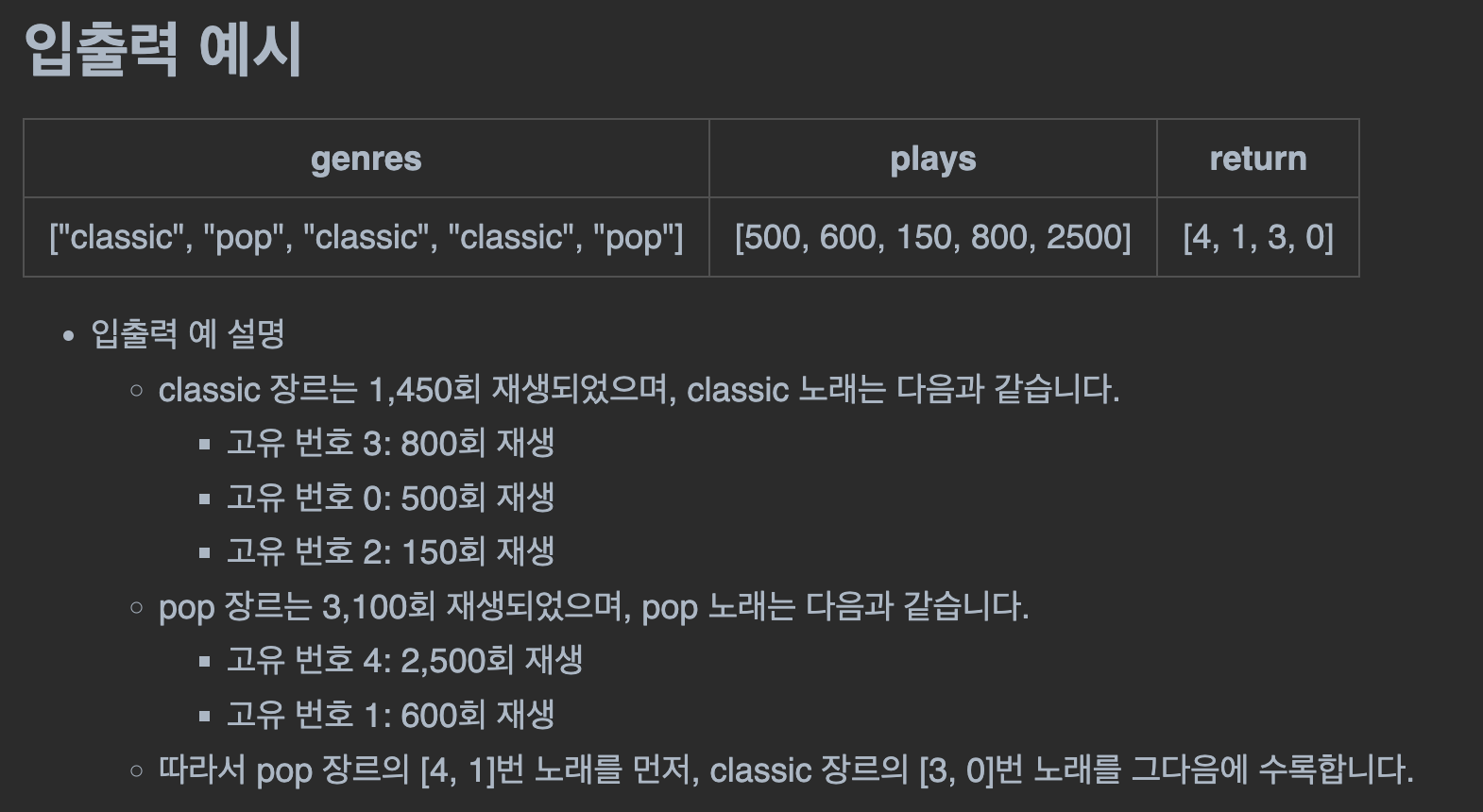
class Song{
int no;
int play;
public Song(int no, int play) {
this.no = no;
this.play = play;
}
}
public class Practice3 {
public static void solution(String[] genres, int[] plays) {
Hashtable<String, ArrayList<Song>> ht = new Hashtable<>();
for(int i = 0; i < genres.length;i++){
if(ht.containsKey(genres[i])){
ArrayList<Song> cur = ht.get(genres[i]);
int idx = -1;
for (int j = 0; j < cur.size(); j++) {
if(plays[i] > cur.get(j).play || plays[i] == cur.get(j).play
&& i < cur.get(j).no){
idx = j;
break;
}
}
if(idx == -1){
cur.add(new Song(i, plays[i]));
}else{
cur.add(idx, new Song(i, plays[i]));
}
ht.put(genres[i], cur);
} else{
Song s = new Song(i, plays[i]);
ht.put(genres[i], new ArrayList<>(Arrays.asList(s)));
}
}
Hashtable<String, Integer> htPlay = new Hashtable<>();
for(String item : ht.keySet()){
int sum = 0;
ArrayList<Song> cur = ht.get(item);
for (int i = 0; i < cur.size(); i++) {
sum += cur.get(i).play;
}
htPlay.put(item, sum);
}
ArrayList<Map.Entry<String, Integer>> htPlaySort = new ArrayList<>(htPlay.entrySet());
htPlaySort.sort((x1, x2) -> x2.getValue() - x1.getValue());
for(Map.Entry<String, Integer>item : htPlaySort){
ArrayList<Song> songs = ht.get(item.getKey());
for (int i = 0; i < songs.size(); i++) {
System.out.print(songs.get(i).no + " ");
if(i == 1){
break;
}
}
}
System.out.println();
}
public static void main(String[] args) {
String[] genres = {"classic", "pop", "classic", "classic", "pop"};
int[] plays = {500, 600, 150, 800, 2500};
solution(genres, plays);
}
}
문제 4
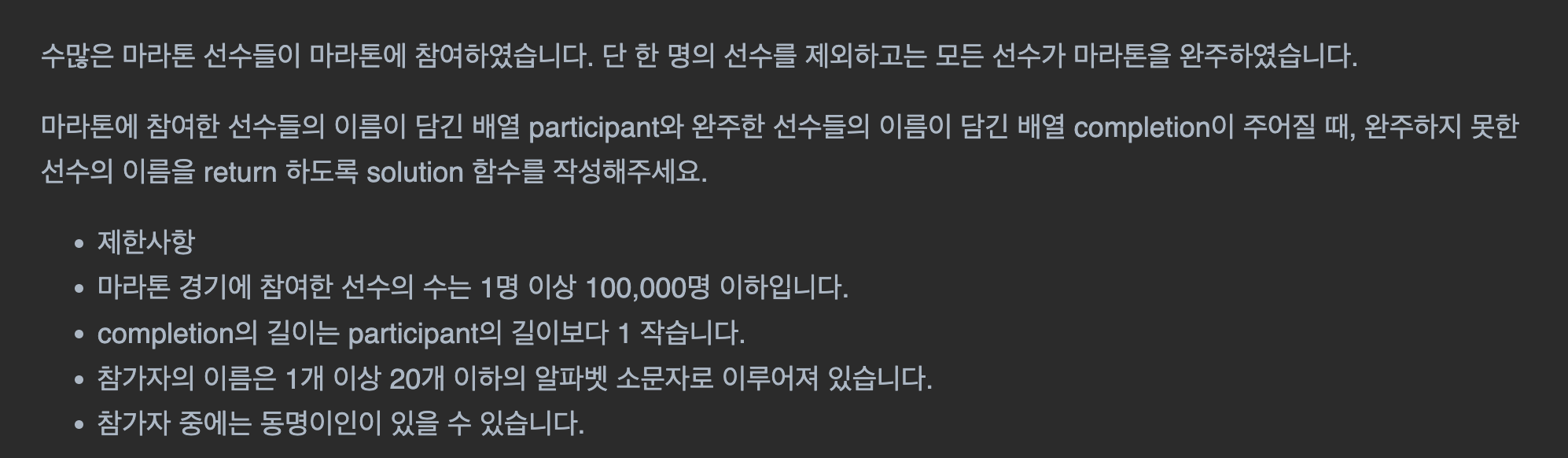
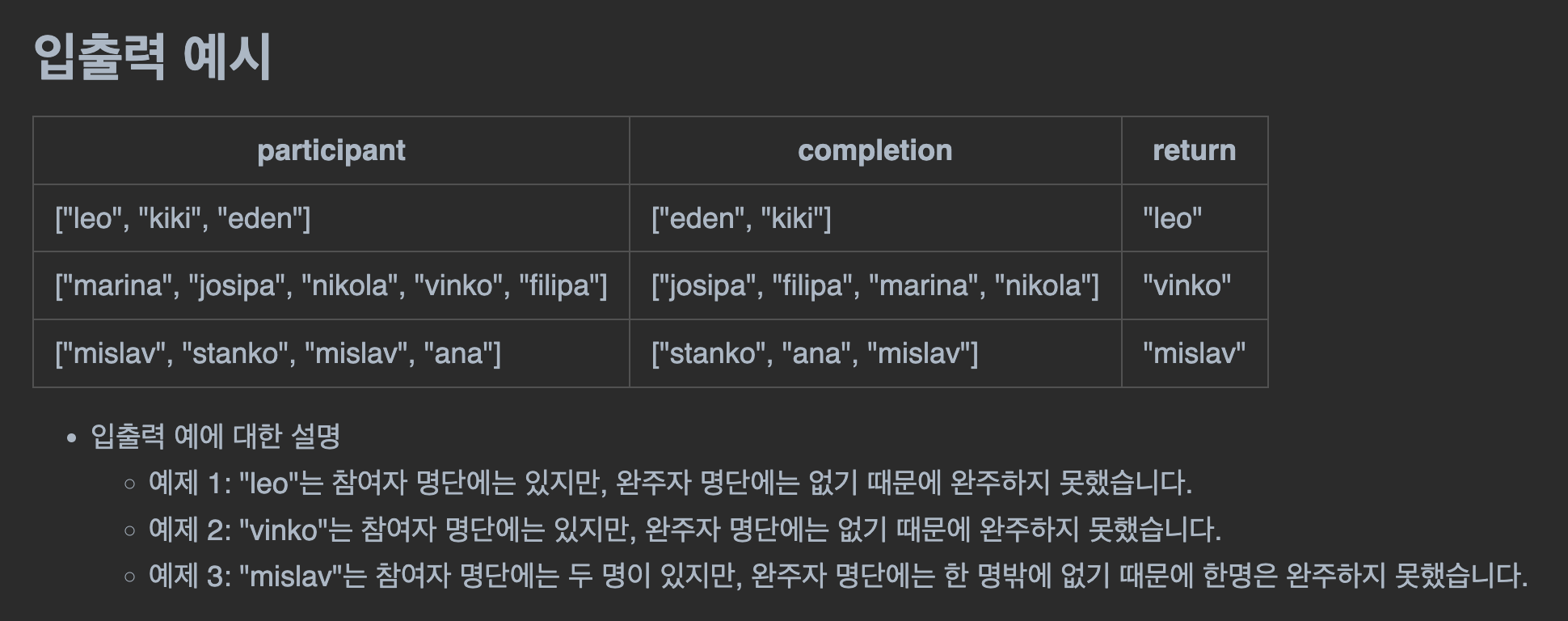
public class Practice4 {
public static String solution(String[] participant, String[] completion) {
String result = "";
Hashtable<String, Integer> ht = new Hashtable<>();
for(String item : participant){
if(ht.containsKey(item)){
ht.put(item, ht.get(item) + 1);
}else{
ht.put(item, 1);
}
}
for(String item : completion){
ht.put(item, ht.get(item) - 1);
}
for(String item: participant){
if(ht.get(item) > 0){
result = item;
break;
}
}
return result;
}
public static void main(String[] args) {
String[] participant = {"leo", "kiki", "eden"};
String[] completion = {"eden", "kiki"};
System.out.println(solution(participant, completion));
participant = new String[]{"marina", "josipa", "nikola", "vinko", "filipa"};
completion = new String[]{"josipa", "filipa", "marina", "nikola"};
System.out.println(solution(participant, completion));
participant = new String[]{"mislav", "stanko", "mislav", "ana"};
completion = new String[]{"stanko", "ana", "mislav"};
System.out.println(solution(participant, completion));
}
}
문제 5
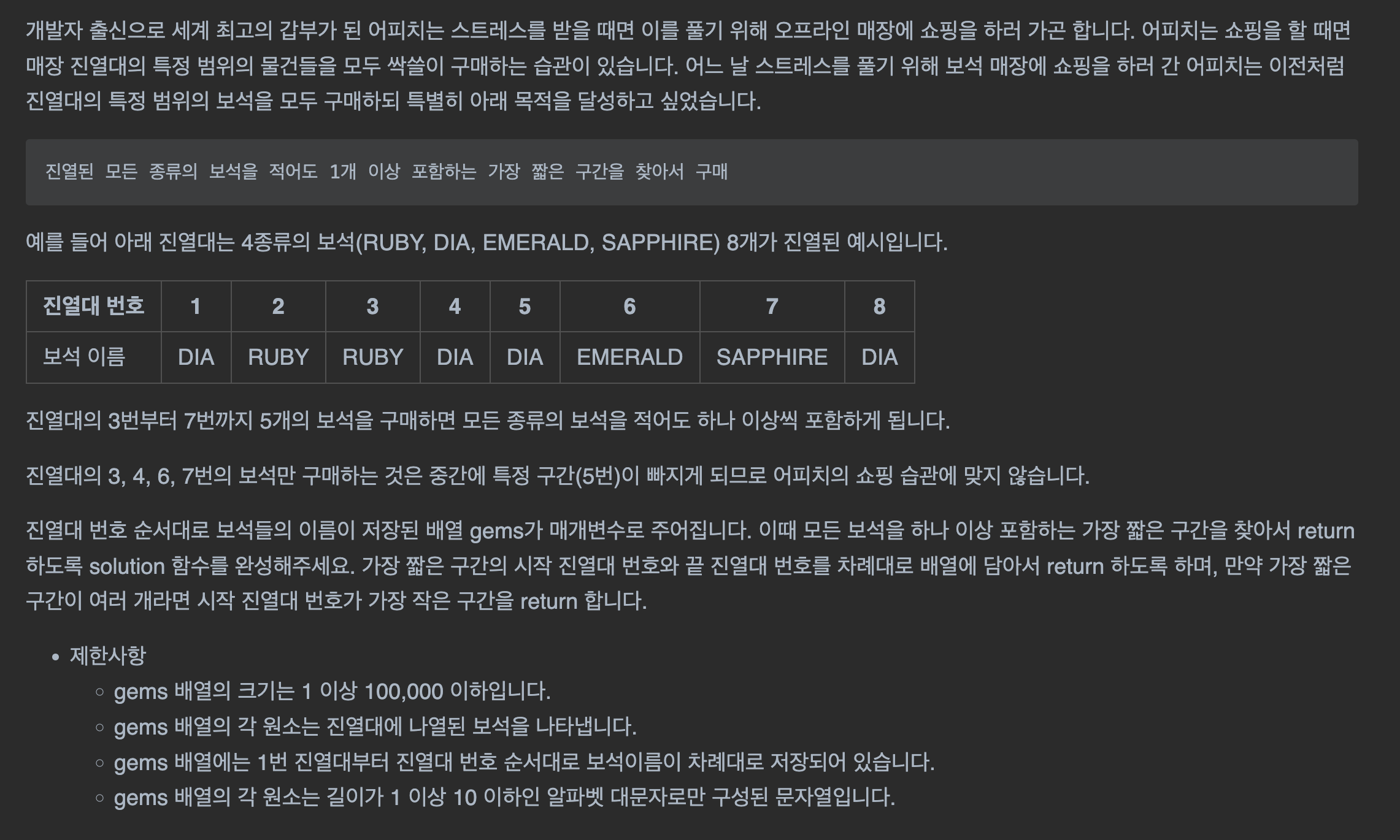
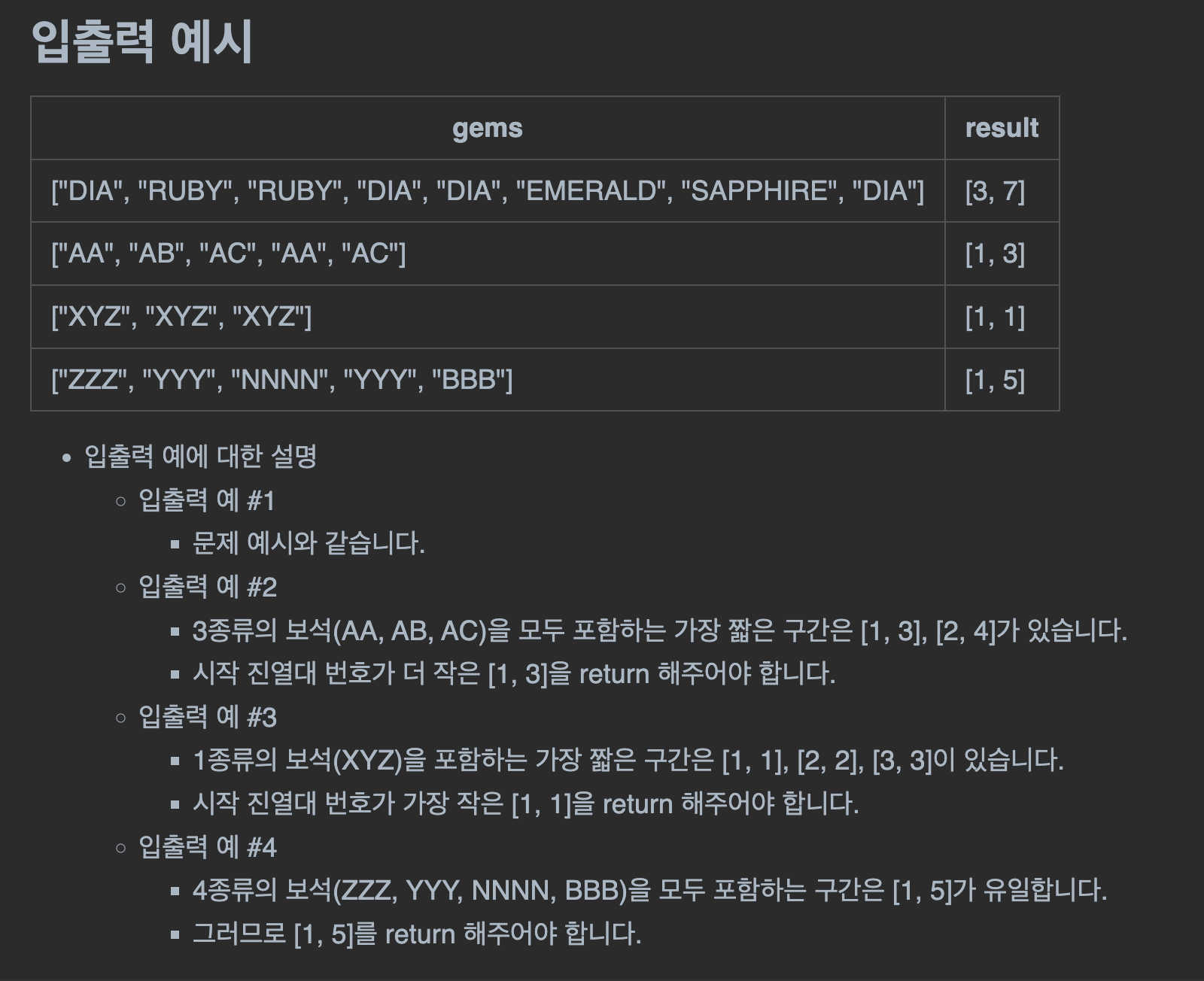
public class Practice5 {
public static ArrayList<Integer> solution(String[] gems) {
ArrayList<ArrayList<Integer>> result = new ArrayList<>();
HashSet<String> set = new HashSet<>();
Stream.of(gems).forEach(x -> set.add(x));
int n = set.size();
if(n == 1){
result.add(new ArrayList<>(Arrays.asList(1,1)));
return result.get(0);
}
Hashtable<String, Integer> ht = new Hashtable<>();
boolean isCandidate = false;
int left = 0; int right = 0;
ht.put(gems[0], 1);
while(true){
if(isCandidate == false){
right += 1;
if(right >= gems.length){
break;
}
if(ht.containsKey(gems[right]) == false){
ht.put(gems[right], 1);
} else{
ht.put(gems[right], ht.get(gems[right]) + 1);
}
if(ht.size() == n){
isCandidate = true;
result.add(new ArrayList<>(Arrays.asList(left + 1, right + 1)));
}
}else{
left += 1;
int cnt = ht.get(gems[left-1]) - 1;
if(cnt == 0){
ht.remove(gems[left - 1]);
isCandidate = false;
} else{
ht.put(gems[left - 1], cnt);
result.add(new ArrayList<>(Arrays.asList(left+1, right + 1)));
}
}
}
int minIdx = 0;
int minNum = Integer.MAX_VALUE;
for (int i = 0; i < result.size(); i++) {
ArrayList<Integer> cur = result.get(i);
left = cur.get(0);
right = cur.get(1);
if(right - left < minNum){
minNum = right - left;
minIdx = i;
}
}
return result.get(minIdx);
}
public static void main(String[] args) {
String[] gems = {"DIA", "RUBY", "RUBY", "DIA", "DIA", "EMERALD", "SAPPHIRE", "DIA"};
System.out.println(solution(gems));
gems = new String[]{"AA", "AB", "AC", "AA", "AC"};
System.out.println(solution(gems));
gems = new String[]{"XYZ", "XYZ", "XYZ"};
System.out.println(solution(gems));
gems = new String[]{"ZZZ", "YYY", "NNNN", "YYY", "BBB"};
System.out.println(solution(gems));
}
}