매개변수
- enable: 클릭이 가능한지 여부(boolean)
- shape: 버튼 모양 지정
- onClick: 버튼을 눌렀을 때 동작 정의
- elevation: 그림자, ButtonDefaults.buttonElevation(...)으로 값 지정
- defaultElevation: 기본 그림자
- pressedElevation: 버튼을 눌렀을 때 그림자
- disabledElevation: enabled가 false일 때 그림자
Button(
enabled = false,
onClick = {
Log.d(TAG, "ButtonContainer: 버튼1 클릭")
}
) {
Text(text = "버튼1")
}
Button(
elevation = ButtonDefaults.buttonElevation(
defaultElevation = 10.dp,
pressedElevation = 0.dp,
disabledElevation = 0.dp
),
onClick = {
Log.d(TAG, "ButtonContainer: 버튼2 클릭")
}
) {
Text(text = "버튼2")
}
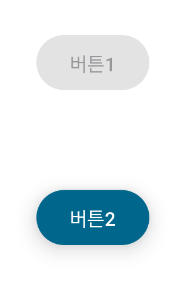
- border: 테두리 크기 및 색상 지정
- BorderStroke(size, color): size.dp만큼 color색 테두리
- BorderStroke(size, Brush): size.dp만큼 Brush색 테두리, 그라데이션 지정 가능
Button(
elevation = ButtonDefaults.buttonElevation(defaultElevation = 10.dp),
shape = RoundedCornerShape(10.dp),
border = BorderStroke(4.dp, Color.Red),
onClick = {
Log.d(TAG, "ButtonContainer: 버튼3 클릭")
}
) {
Text(text = "버튼3")
}
val buttonBorderGradiant = Brush.horizontalGradient(listOf(Color.Yellow, Color.Red))
Button(
elevation = ButtonDefaults.buttonElevation(defaultElevation = 10.dp),
shape = RoundedCornerShape(10.dp),
border = BorderStroke(4.dp, buttonBorderGradiant),
colors = ButtonDefaults.buttonColors(
containerColor = Color.Black,
disabledContainerColor = Color.LightGray
),
onClick = {
Log.d(TAG, "ButtonContainer: 버튼4 클릭")
}
) {
Text(text = "버튼4")
}
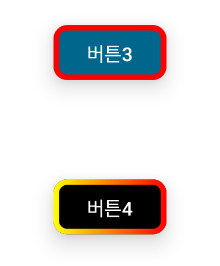
- interactionSource: 사용자의 인터랙션 처리
- remember { MutableInteractionSource() }로 지정
- interactionSource.collectIsPressedAsState()를 위임하여 눌렀을 때 boolean값 지정 가능
- 클릭에 따른 Button shadow animation 지정 가능
- Modifier.coloredShadow 참고코드
val interactionSource = remember { MutableInteractionSource() }
val interactionSourceForSecondBtn = remember { MutableInteractionSource() }
val isPressed by interactionSource.collectIsPressedAsState()
val isPressedForSecondBtn by interactionSourceForSecondBtn.collectIsPressedAsState()
val pressStatusTitle = if (isPressed) "버튼을 누르고 있다." else "버튼에서 손을 뗐다."
val pressedBtnRadius = if (isPressedForSecondBtn) 0.dp else 20.dp
val pressedBtnRadiusWithAnim: Dp by animateDpAsState(targetValue = pressedBtnRadius)
Button(
elevation = ButtonDefaults.buttonElevation(defaultElevation = 10.dp),
shape = RoundedCornerShape(10.dp),
contentPadding = PaddingValues(horizontal = 5.dp, vertical = 20.dp),
interactionSource = interactionSource,
onClick = {
Log.d(TAG, "ButtonContainer: 버튼5 클릭")
}
) {
Text(text = "버튼5")
}
Text(text = pressStatusTitle)
Button(
elevation = ButtonDefaults.buttonElevation(defaultElevation = 10.dp),
shape = RoundedCornerShape(10.dp),
contentPadding = PaddingValues(horizontal = 5.dp, vertical = 20.dp),
interactionSource = interactionSourceForSecondBtn,
modifier = Modifier.coloredShadow(
color = Color.Red,
alpha = 0.5f,
borderRadius = 10.dp,
shadowRadius = pressedBtnRadiusWithAnim,
offsetY = 0.dp,
offsetX = 0.dp
),
onClick = {
Log.d(TAG, "ButtonContainer: 버튼6 클릭")
}
) {
Text(text = "버튼6")
}
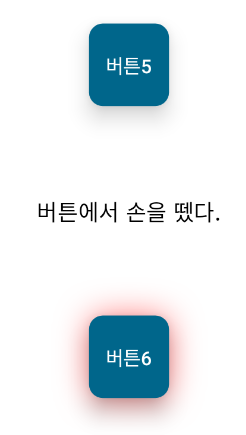